POJ1456 Supermarket
Time Limit: 2000MS Memory Limit: 65536K
Description
A supermarket has a set Prod of products on sale. It earns a profit px for each product x∈Prod sold by a deadline dx that is measured as an integral number of time units starting from the moment the sale begins. Each product takes precisely one unit of time for being sold. A selling schedule is an ordered subset of products Sell ≤ Prod such that the selling of each product x∈Sell, according to the ordering of Sell, completes before the deadline dx or just when dx expires. The profit of the selling schedule is Profit(Sell)=Σx∈Sellpx. An optimal selling schedule is a schedule with a maximum profit.
For example, consider the products Prod={a,b,c,d} with (pa,da)=(50,2), (pb,db)=(10,1), (pc,dc)=(20,2), and (pd,dd)=(30,1). The possible selling schedules are listed in table 1. For instance, the schedule Sell={d,a} shows that the selling of product d starts at time 0 and ends at time 1, while the selling of product a starts at time 1 and ends at time 2. Each of these products is sold by its deadline. Sell is the optimal schedule and its profit is 80.
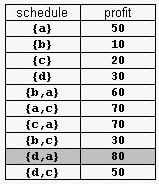
Write a program that reads sets of products from an input text file and computes the profit of an optimal selling schedule for each set of products.
Input
A set of products starts with an integer 0 <= n <= 10000, which is the number of products in the set, and continues with n pairs pi di of integers, 1 <= pi <= 10000 and 1 <= di <= 10000, that designate the profit and the selling deadline of the i-th product. White spaces can occur freely in input. Input data terminate with an end of file and are guaranteed correct.
Output
For each set of products, the program prints on the standard output the profit of an optimal selling schedule for the set. Each result is printed from
the beginning of a separate line. Sample Input
4
50 2 10 1 20 2 30 1
7
20 1 2 1 10 3 100 2 8 2 5 20 50 10
Sample Output
80
185
Hint
The sample input contains two product sets. The first set encodes the products from table 1. The second set is for 7 products. The profit of an optimal schedule for these products is 185.
Source
Southeastern Europe 2003
Code
#include<iostream>
#include<queue>
using namespace std;
const int maxn = 1e4 + 3;
inline int read()
{
int x = 0, f = 1; char ch = getchar();
while (ch < '0' || ch>'9') { if (ch == '-')f = -1; ch = getchar(); }
while (ch >= '0' && ch <= '9') x = x * 10 + ch - 48, ch = getchar();
return x * f;
}
struct node {
int w, t;
node(int w = 0, int t = 0) :w(w), t(t){}
}nodes[maxn];
bool cmp(node a, node b) {
if(a.t != b.t) return a.t < b.t;
return a.w > b.w;
}
int main() {
int n, w, t;
while (cin >> n) {
for (int i = 0; i < n; i++) {
w = read(), t = read();
nodes[i] = node(w, t);
}
sort(nodes, nodes + n, cmp);
priority_queue<int, vector<int>, greater<int>> pq;
for (int i = 0; i < n; i++) {
if (nodes[i].t > pq.size()) pq.push(nodes[i].w);
else if (pq.size() && nodes[i].t == pq.size()) {
if (nodes[i].w > pq.top()) {
pq.pop();
pq.push(nodes[i].w);
}
}
}
int ans = 0;
while (pq.size()) {
ans += pq.top();
pq.pop();
}
cout << ans << endl;
}
return 0;
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)