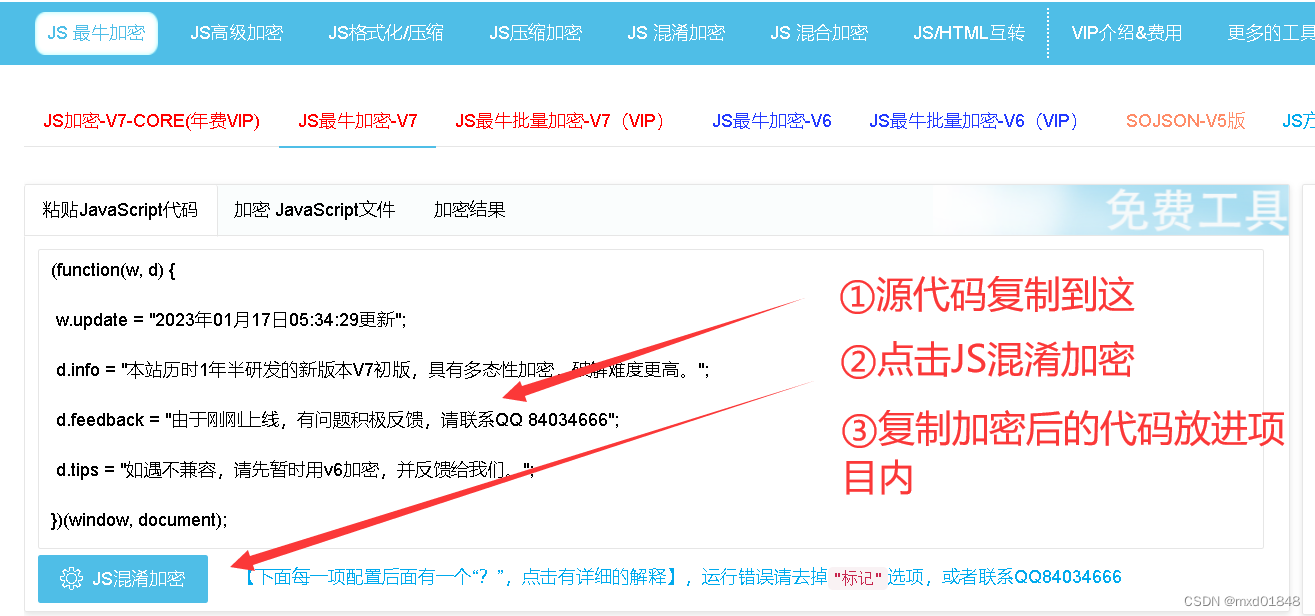
JS中的 this
关键字是一个非常重要的概念,它在不同情况下会指向不同的对象或值。在本文中,我们将深入探讨 JavaScript 中 this
的各种情况,并思考如何将其应用于 JS加密中的一些有趣用途。
1. 全局上下文中的 this
在全局上下文中,this
指向全局对象,通常是浏览器环境中的 window
对象。这种情况下,this
可以用于创建全局变量。
console.log(this === window); // 输出 true(在浏览器中)
this.globalVar = 42;
console.log(window.globalVar); // 输出 42
2. 函数中的 this
在函数内部,this
的值取决于函数的调用方式。以下是几种常见的情况:
2.1 方法中的 this
当函数作为对象的方法被调用时,this
指向调用该方法的对象。
const person = {
name: 'Alice',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // 输出 "Hello, my name is Alice"
2.2 全局函数中的 this
如果函数在全局作用域中被调用,this
仍然指向全局对象。
function globalFunction() {
console.log(this === window); // 输出 true(在浏览器中)
}
globalFunction();
2.3 构造函数中的 this
使用 new
关键字创建对象实例时,构造函数内部的 this
指向新创建的对象。
function Person(name) {
this.name = name;
}
const alice = new Person('Alice');
console.log(alice.name); // 输出 "Alice"
2.4 使用 call
或 apply
改变 this
可以使用 call
或 apply
方法显式地指定函数内部的 this
。
function greet() {
console.log(`Hello, ${this.name}`);
}
const person1 = { name: 'Bob' };
const person2 = { name: 'Carol' };
greet.call(person1); // 输出 "Hello, Bob"
greet.apply(person2); // 输出 "Hello, Carol"
3. 箭头函数中的 this
箭头函数 (=>
) 与常规函数不同,它们没有自己的 this
绑定,而是继承外部函数的 this
。
const obj = {
value: 42,
getValue: function() {
const arrowFunc = () => {
console.log(this.value);
};
arrowFunc();
}
};
obj.getValue(); // 输出 "42",因为箭头函数继承了 obj 的 this
4. 事件处理程序中的 this
在事件处理程序中,this
通常指向触发事件的元素。
<button id="myButton">Click me</button>
<script>
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
console.log(this); // 输出按钮元素
});
</script>
JavaScript 加密中的 this
应用
将 this
应用于 JavaScript 加密中可能不是常见的用途,但在某些情况下,它可以用于改善加密算法的灵活性和安全性。以下是一些可能的用途:
1. 动态密钥生成
使用函数中的 this
可以生成动态密钥。例如,可以创建一个对象,该对象包含一个方法,该方法使用 this
来生成加密密钥。
const keyGenerator = {
generateKey: function() {
// 使用 this 中的信息生成动态密钥
return this.sensitiveData + Math.random();
},
sensitiveData: 'mySecretKey'
};
const dynamicKey = keyGenerator.generateKey();
console.log(dynamicKey);
2. 安全存储
在加密中,this
可以用于安全存储加密密钥或敏感数据。通过将密钥存储在对象属性中,并使用适当的访问控制,可以提高密钥的安全性。
const encryptionConfig = {
key: null,
setKey: function(newKey) {
if (this.key === null) {
this.key = newKey;
console.log('Key set successfully.');
} else {
console.log('Key already set. Cannot overwrite.');
}
}
};
encryptionConfig.setKey('mySuperSecretKey'); // 设置密钥
console.log(encryptionConfig.key); // 访问密钥
encryptionConfig.setKey('newKey'); // 尝试覆盖密钥
需要注意的是,JS加密应用通常需要更复杂的安全措施,因此应该谨慎处理密钥和敏感数据,以确保其安全性。
结论
this
在 JavaScript 中是一个关键的概念,它的指向在不同情况下有所不同。了解这些不同情况对于编写高效的 JavaScript 代码至关重要。此外,虽然 this
可能不是 JavaScript 加密的核心概念,但在某些情况下,它可以用于改进加密算法的功能和安全性,前提是需要小心处理和保护密钥和敏感数据。