文章目录
- 一、单目运算符的重载
- 二、双目运算符的重载,使其能运算字符串的> < ==
一、单目运算符的重载
#include <iostream>
using namespace std;
class Time
{
public:
Time()
{
minute=0; sec=0;
}
Time(int m, int s):minute(m), sec(s){ }
Time operator ++ ();
void display()
{
cout << minute << ":" << sec << " ";
}
private:
int minute;
int sec;
};
Time Time::operator ++()
{
if(++sec>=60)
{
sec -= 60;
++minute;
return *this;
}
}
int main()
{
Time time1(34,0);
for(int i=0; i<61; i++)
{
++time1;
time1.display();
}
return 0;
}
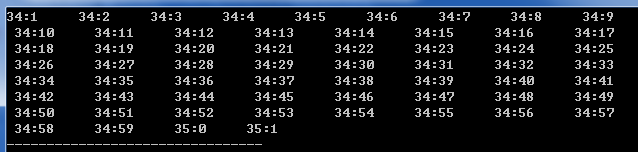
二、双目运算符的重载,使其能运算字符串的> < ==
#include <iostream>
#include <string.h>
using namespace std;
class String
{
public:
String()
{
p=NULL;
}
String(char *str);
friend bool operator > (String &string1, String &string2);
void display();
private:
char *p;
};
String::String(char *str)
{
p= str;
}
void String::display()
{
cout << p;
}
bool operator > (String &string1, String &string2)
{
if(strcmp(string1.p, string2.p)>0)
return true;
else return false;
}
int main()
{
String string1("Hello"), string2("Book");
string1.display();
cout << endl;
string2.display();
bool res;
string result;
cout << endl;
res= string1>string2;
if(res==1)
result= "大于";
else
result= "小于";
cout << "字符串";
string1.display();
cout << result;
string2.display();
cout << endl;
return 0;
}
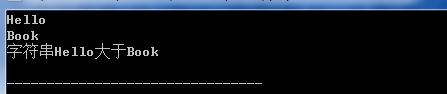
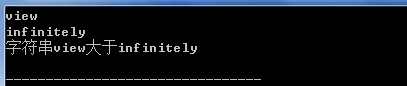

本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)