多表关系
在关系型数据库中存在着三种多表关系,分别是一对多
(多对一
)、多对多
以及一对一
。之所以会产生这些关系,是因为在进行数据设计的时候,分析得出业务之间存在着一定的关系,进而在数据中也就存在了这些关系。
一对多
一对多是最基础的表间关系,意思是一张表A中的一条记录可以对应另一张表B中的多条记录,另一张表B中的一条记录只能对应一张表A中的一条记录。
案例: 部门
与 员工
的关系
关系: 一个部门对应多个员工,一个员工对应一个部门
实现: 在多的一方建立外键,指向一的一方的主键
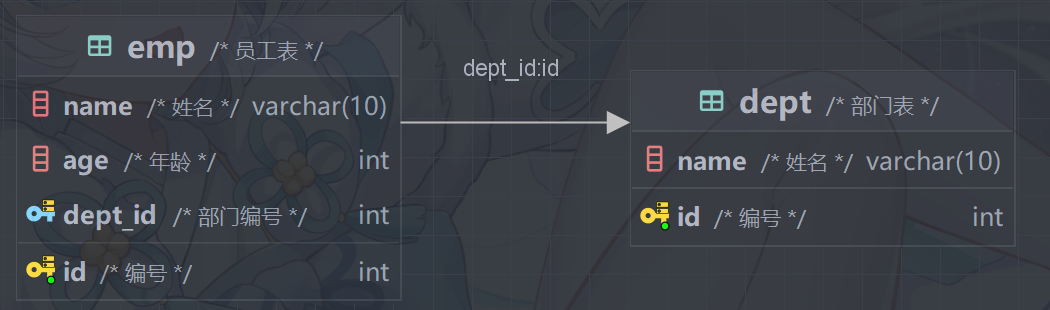
对应的SQL脚本
-- 创建部门表
create table dept
(
id int primary key auto_increment comment '编号',
name varchar(10) comment '姓名'
) comment '部门表';
-- 创建员工表
create table emp
(
id int primary key auto_increment comment '编号',
name varchar(10) comment '姓名',
age int comment '年龄',
dept_id int comment '部门编号',
constraint fk_dept_emp foreign key (dept_id) references dept (id)
) comment '员工表';
多对多
一张表A中的一条记录可以对应另一张表B中的多条记录,另一张表B中的一条记录也可以对应一张表A中的多条记录。
案例: 学生
与 课程
的关系
关系: 一个学生可以选修多门课程,一门课程也可以供多个学生选择
实现: 建立第三张中间表,中间表至少包含两个外键,分别关联两方主键
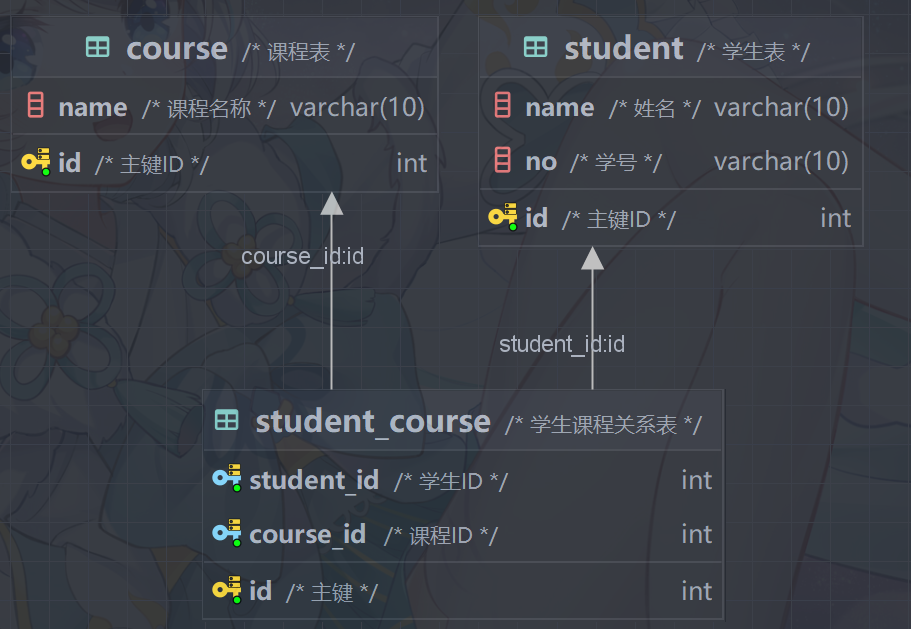
对应的SQL脚本
-- 创建学生表
create table student
(
id int auto_increment primary key comment '主键ID',
name varchar(10) comment '姓名',
no varchar(10) comment '学号'
) comment '学生表';
-- 创建课程表
create table course
(
id int auto_increment primary key comment '主键ID',
name varchar(10) comment '课程名称'
) comment '课程表';
-- 创建学生与课程的关系表
create table student_course
(
id int auto_increment primary key comment '主键',
student_id int not null comment '学生ID',
course_id int not null comment '课程ID',
constraint fk_courseId foreign key (course_id) references course (id),
constraint fk_studentId foreign key (student_id) references student (id)
) comment '学生课程关系表';
一对一
一对一的关系就是一种特殊的多对多的关系,一张表A中的一条记录只能对应另一张表B中的一条记录,另一张表B中的一条记录也只能对应一张表A中的一条记录。
案例: 用户
与 用户
详情的关系
关系: 一对一关系,多用于单表拆分,将一张表的基础字段放在一张表中,其他详情字段放在另一张表中,以提升操作效率
实现: 在任意一方加入外键,关联另外一方的主键,并且设置外键为唯一的(UNIQUE)
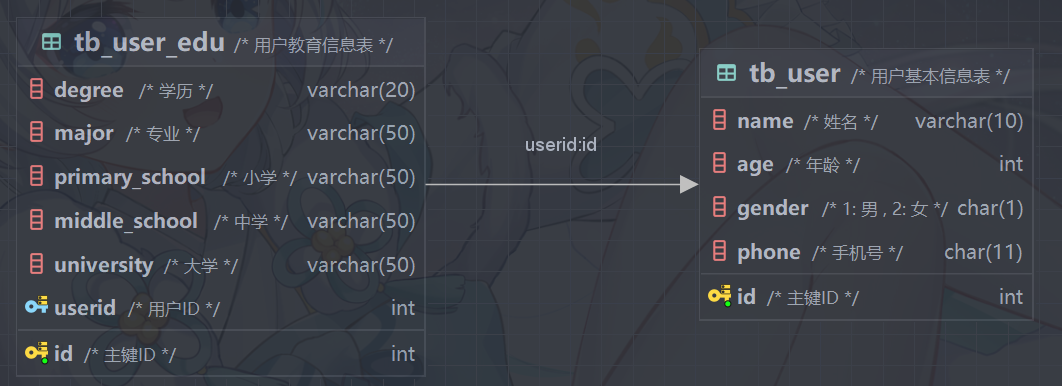
对应的SQL脚本
-- 创建用户表
create table tb_user
(
id int auto_increment primary key comment '主键ID',
name varchar(10) comment '姓名',
age int comment '年龄',
gender char(1) comment '1: 男 , 2: 女',
phone char(11) comment '手机号'
) comment '用户基本信息表';
-- 创建用户教育表
create table tb_user_edu
(
id int auto_increment primary key comment '主键ID',
degree varchar(20) comment '学历',
major varchar(50) comment '专业',
primary_school varchar(50) comment '小学',
middle_school varchar(50) comment '中学',
university varchar(50) comment '大学',
userid int unique comment '用户ID',
constraint fk_userid foreign key (userid) references tb_user (id)
) comment '用户教育信息表';
多表查询概述
准备数据
-- 删除员工表(若存在)
drop table if exists emp;
-- 删除部门表(若存在)
drop table if exists dept;
-- 创建dept表,并插入数据
create table dept
(
id int auto_increment comment 'ID' primary key,
name varchar(50) not null comment '部门名称'
) comment '部门表';
INSERT INTO dept (id, name)
VALUES (1, '研发部'),
(2, '市场部'),
(3, '财务部'),
(4, '销售部'),
(5, '总经办'),
(6, '人事部');
-- 创建emp表,并插入数据
create table emp
(
id int auto_increment comment 'ID' primary key,
name varchar(50) not null comment '姓名',
age int comment '年龄',
job varchar(20) comment '职位',
salary int comment '薪资',
entry_date date comment '入职时间',
manager_id int comment '直属领导ID',
dept_id int comment '部门ID'
) comment '员工表';
-- 添加外键
alter table emp
add constraint fk_emp_dept_id foreign key (dept_id) references dept (id);
INSERT INTO emp (id, name, age, job, salary, entry_date, manager_id, dept_id)
VALUES (1, '金庸', 66, '总裁', 20000, '2000-01-01', null, 5),
(2, '张无忌', 20, '项目经理', 12500, '2005-12-05', 1, 1),
(3, '杨逍', 33, '开发', 8400, '2000-11-03', 2, 1),
(4, '韦一笑', 48, '开发', 11000, '2002-02-05', 2, 1),
(5, '常遇春', 43, '开发', 10500, '2004-09-07', 3, 1),
(6, '小昭', 19, '程序员鼓励师', 6600, '2004-10-12', 2, 1),
(7, '灭绝', 60, '财务总监', 8500, '2002-09-12', 1, 3),
(8, '周芷若', 19, '会计', 48000, '2006-06-02', 7, 3),
(9, '丁敏君', 23, '出纳', 5250, '2009-05-13', 7, 3),
(10, '赵敏', 20, '市场部总监', 12500, '2004-10-12', 1, 2),
(11, '鹿杖客', 56, '职员', 3750, '2006-10-03', 10, 2),
(12, '鹤笔翁', 19, '职员', 3750, '2007-05-09', 10, 2),
(13, '方东白', 19, '职员', 5500, '2009-02-12', 10, 2),
(14, '张三丰', 88, '销售总监', 14000, '2004-10-12', 1, 4),
(15, '俞莲舟', 38, '销售', 4600, '2004-10-12', 14, 4),
(16, '宋远桥', 40, '销售', 4600, '2004-10-12', 14, 4),
(17, '陈友谅', 42, null, 2000, '2011-10-12', 1, null);
表之间的对应关系如下:
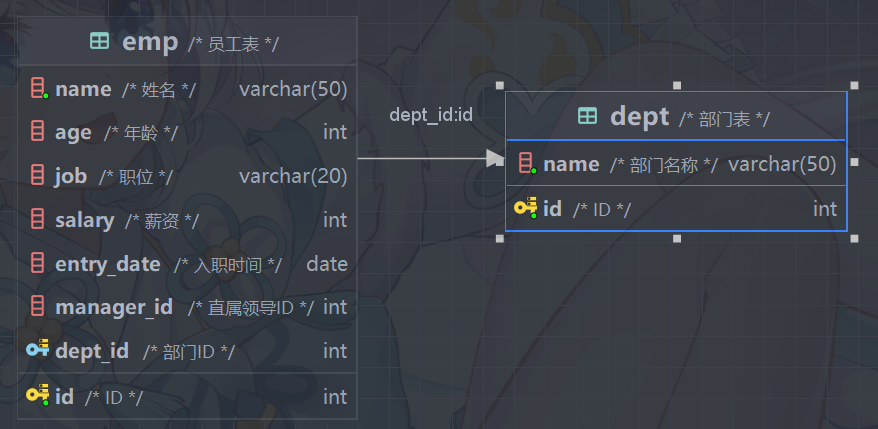
概述
多表查询就是指从多张表中查询数据。
原来查询单表数据,执行的SQL形式为:
select *
from emp;
那么我们要执行多表查询,就只需要使用逗号分隔多张表即可,如:
select *
from emp,
dept;
具体的执行结果如下:
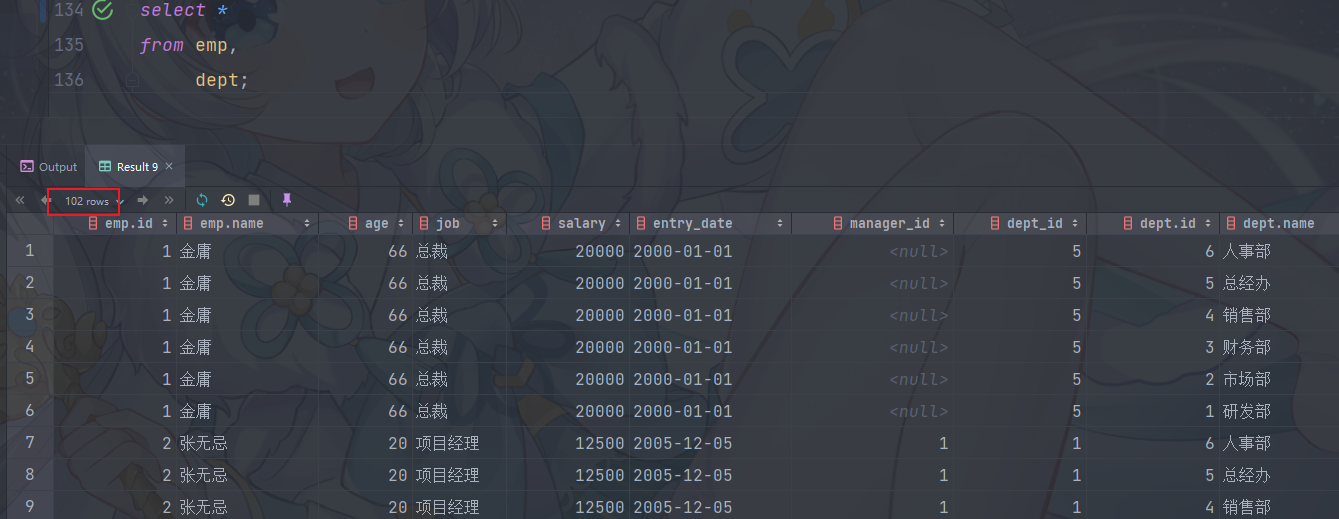
此时,我们看到查询结果中包含了大量的结果集,总共102条记录,而这其实就是员工表emp所有的记录(17条) 与 部门表dept所有记录(6条) 的所有组合情况,这种现象称之为笛卡尔积。
可以通过查询时,加上连接条件来消除无效的笛卡尔积。
select *
from emp,
dept
where emp.dept_id = dept.id;
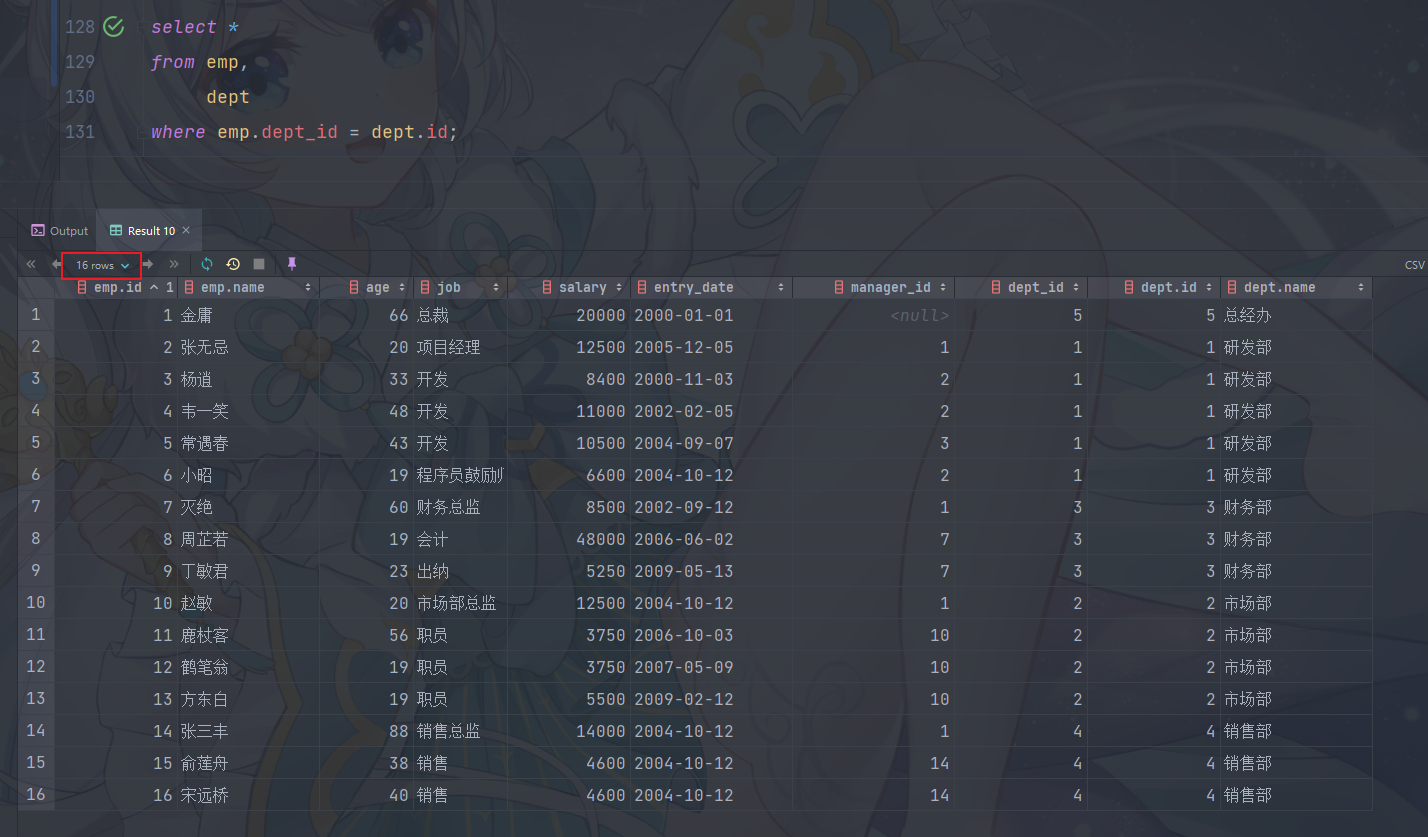
而由于id为17的员工,没有dept_id字段值,所以在多表查询时,根据连接查询的条件并没有查询到。
分类
- 连接查询
- 内连接:相当于查询A、B交集部分数据
- 外连接:
- 左外连接:查询左表所有数据,以及两张表交集部分数据
- 右外连接:查询右表所有数据,以及两张表交集部分数据
- 自连接:当前表与自身的连接查询,自连接必须使用表别名
- 子查询
内连接
内连接查询的是两张表交集部分的数据。
内连接的语法分为两种: 隐式内连接、显式内连接。
隐式内连接
SELECT 字段列表 FROM 表1 , 表2 WHERE 条件 ... ;
显式内连接
SELECT 字段列表 FROM 表1 [ INNER ] JOIN 表2 ON 连接条件 ... ;
案例
A. 查询每一个员工的姓名 , 及关联的部门的名称 (隐式内连接实现)
表结构: emp
, dept
连接条件: emp.dept_id = dept.id
select e.name, d.name
from dept d,
emp e
where d.id = e.dept_id;
B. 查询每一个员工的姓名 , 及关联的部门的名称 (显式内连接实现) — INNER JOIN … ON …
表结构: emp
, dept
连接条件: emp.dept_id = dept.id
select e.name, d.name
from dept d
inner join
emp e
on d.id = e.dept_id;
注意:一旦为表起了别名,就不能再使用表名来指定对应的字段了,此时只能够使用别名来指定字段。
外连接
外连接分为两种,分别是:左外连接 和 右外连接。
左外连接
具体的语法结构为:
SELECT 字段列表 FROM 表1 LEFT [ OUTER ] JOIN 表2 ON 条件 ... ;
左外连接相当于查询表1(左表)的所有数据,当然也包含表1和表2交集部分的数据。
右外连接
具体的语法结构为:
SELECT 字段列表 FROM 表1 RIGHT [ OUTER ] JOIN 表2 ON 条件 ... ;
右外连接相当于查询表2(右表)的所有数据,当然也包含表1和表2交集部分的数据。
案例
A. 查询emp表的所有数据, 和对应的部门信息
由于需求中提到,要查询emp的所有数据,所以是不能内连接查询的,需要考虑使用外连接查询。
表结构: emp
, dept
连接条件: emp.dept_id = dept.id
select e.*, d.name
from emp e
left join dept d on d.id = e.dept_id;
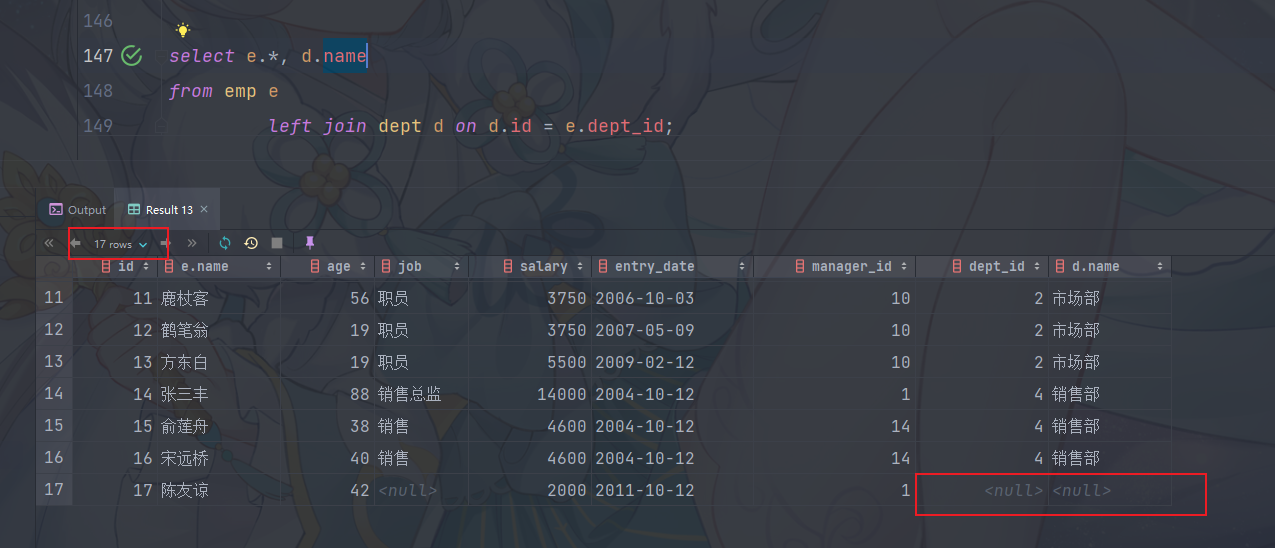
B. 查询dept表的所有数据, 和对应的员工信息(右外连接)
由于需求中提到,要查询dept表的所有数据,所以是不能内连接查询的,需要考虑使用外连接查询。
表结构: emp
, dept
连接条件: emp.dept_id = dept.id
select d.*, e.*
from emp e
right join dept d on d.id = e.dept_id;
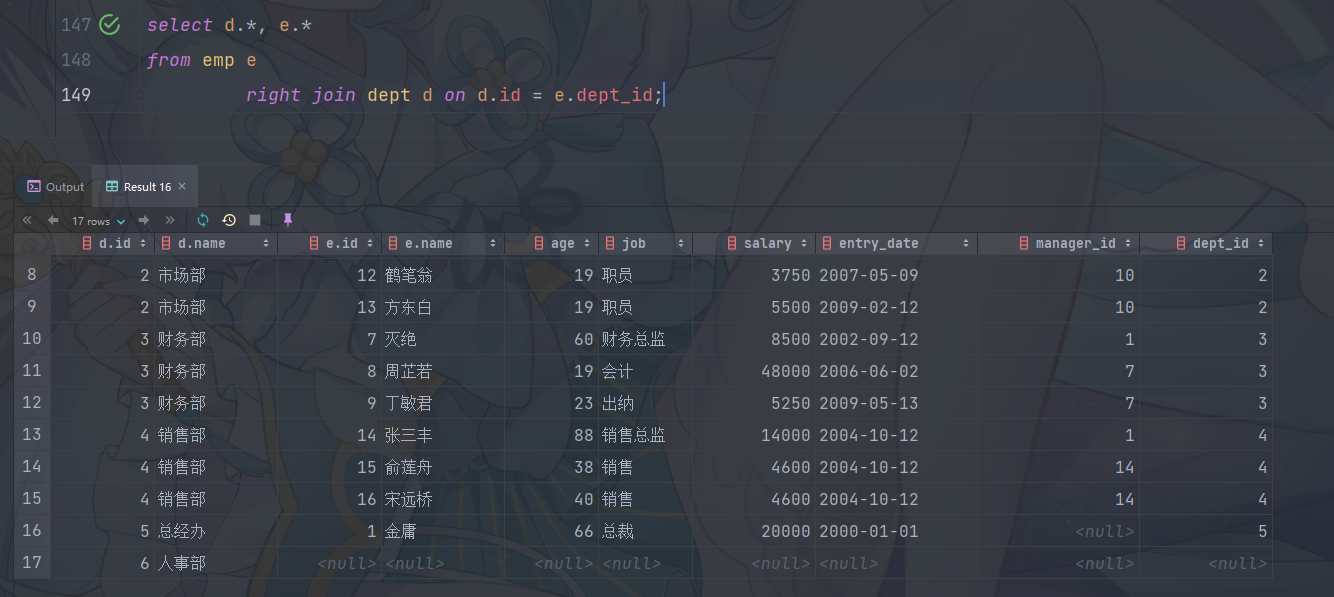
注意:左外连接和右外连接是可以相互替换的,只需要调整在连接查询时SQL中,表结构的先后顺
序就可以了。在日常开发使用时,更偏向于左外连接。
自连接
自连接查询
自连接查询,顾名思义,就是自己连接自己,也就是把一张表连接查询多次。
自连接的查询语法:
SELECT 字段列表 FROM 表A 别名A JOIN 表A 别名B ON 条件 ... ;
而对于自连接查询,可以是内连接查询,也可以是外连接查询。
案例:
A. 查询员工 及其 所属领导的名字
表结构: emp
select a.name, b.name
from emp a
inner join emp b on a.manager_id = b.id;
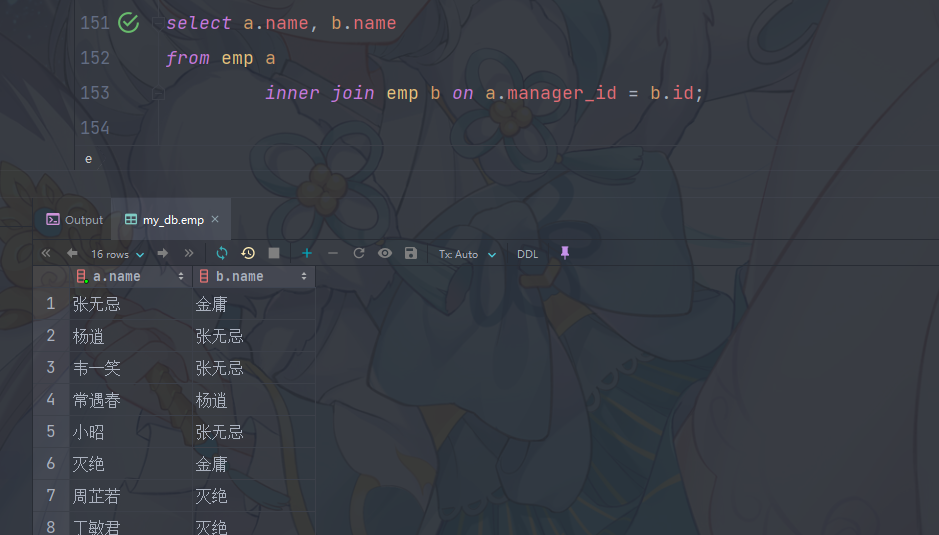
B. 查询所有员工 及其领导的名字 , 如果员工没有领导, 也需要查询出来
表结构: emp a
, emp b
select a.name, b.name
from emp a
left join emp b on a.manager_id = b.id;
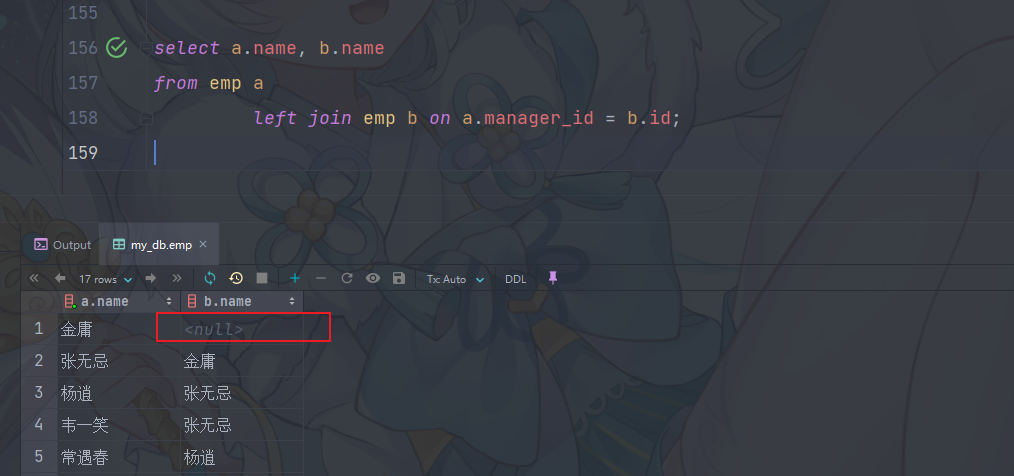
注意:在自连接查询中,必须要为表起别名,要不然我们不清楚所指定的条件、返回的字段,到底是哪一张表的字段。
联合查询
对于union查询,就是把多次查询的结果合并起来,形成一个新的查询结果集。
基本语法结构:
SELECT 字段列表 FROM 表A ...
UNION [ ALL ]
SELECT 字段列表 FROM 表B ....;
对于联合查询的多张表的列数必须保持一致,字段类型也需要保持一致。
union all
会将全部的数据直接合并在一起,union
会对合并之后的数据去重。
案例
A. 将薪资低于 5000 的员工 , 和 年龄大于 50 岁的员工全部查询出来.
当前对于这个需求,我们可以直接使用多条件查询,使用逻辑运算符 or
连接即可。 那这里呢,也可以通过 union all
/ union
来联合查询.
select *
from emp
where salary < 5000
union all
select *
from emp
where age > 50;
union all
查询出来的结果,仅仅进行简单的合并,并未去重。
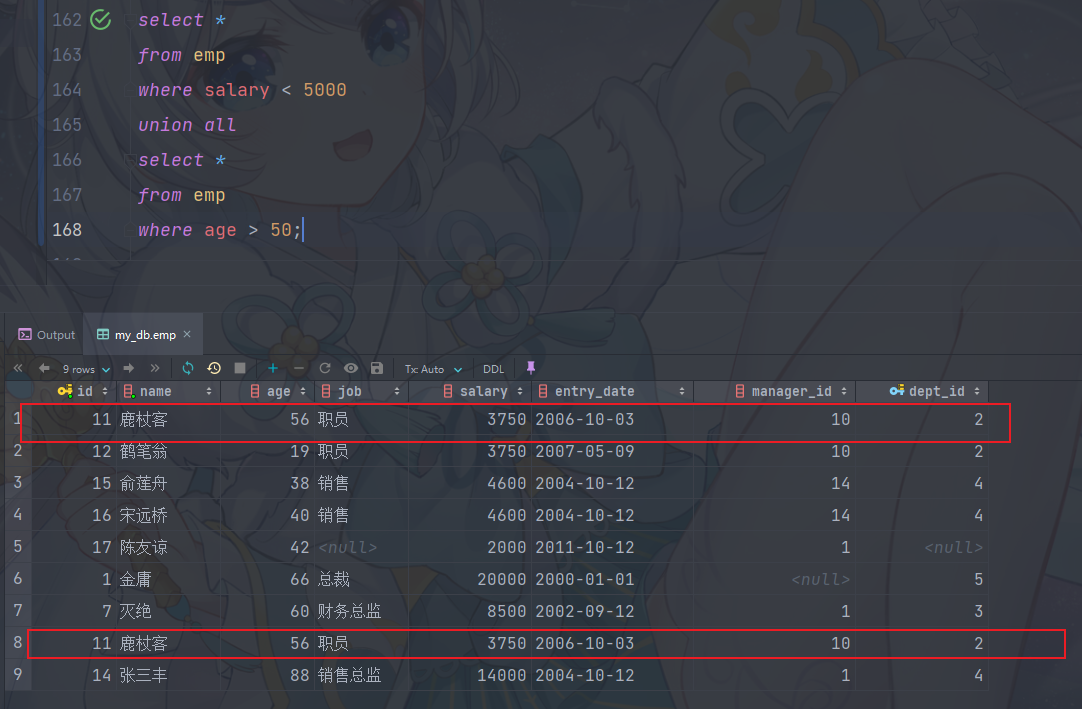
select *
from emp
where salary < 5000
union
select *
from emp
where age > 50;
union
联合查询,会对查询出来的结果进行去重处理。
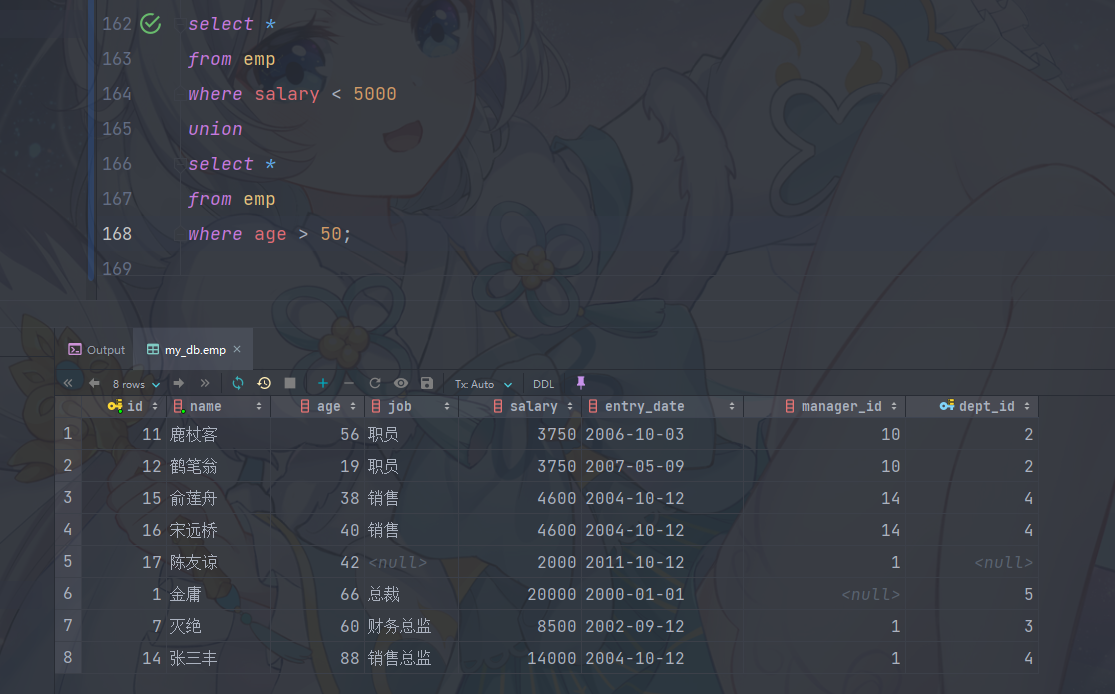
注意:如果多条查询语句查询出来的结果,字段数量不一致,在进行 union all
/ union
联合查询时,将会报错[21000][1222] The used SELECT statements have a different number of columns
。如:
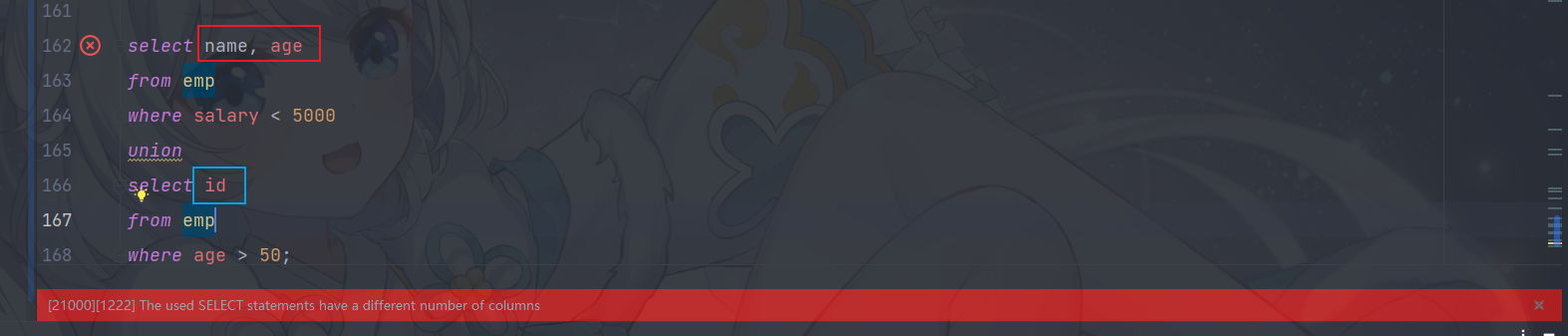
子查询
概述
概念
SQL语句中嵌套SELECT语句,称为嵌套查询,又称子查询。
SELECT * FROM t1 WHERE column1 = ( SELECT column1 FROM t2 );
子查询外部的语句可以是INSERT
/ UPDATE
/ DELETE
/ SELECT
的任何一个。
分类
根据子查询结果不同,分为:
- 标量子查询(子查询结果为单个值)
- 列子查询(子查询结果为一列)
- 行子查询(子查询结果为一行)
- 表子查询(子查询结果为多行多列)
根据子查询位置,分为:
标量子查询
子查询返回的结果是单个值(数字、字符串、日期等),最简单的形式,这种子查询称为标量子查询。
常用的操作符:=
<>
>
>=
<
<=
案例
A. 查询 “销售部” 的所有员工信息
完成这个需求时,我们可以将需求分解为两步:
①. 查询 “销售部” 部门ID
select id
from dept
where name = '销售部';
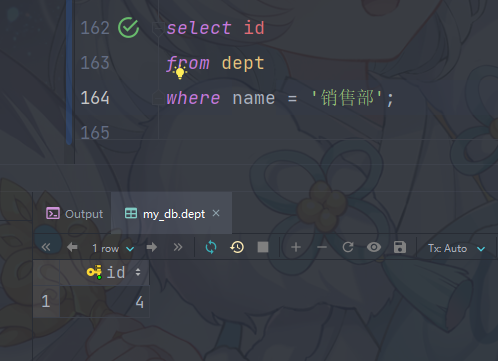
②. 根据 “销售部” 部门ID, 查询员工信息
select *
from emp
where dept_id = (select id
from dept
where name = '销售部');
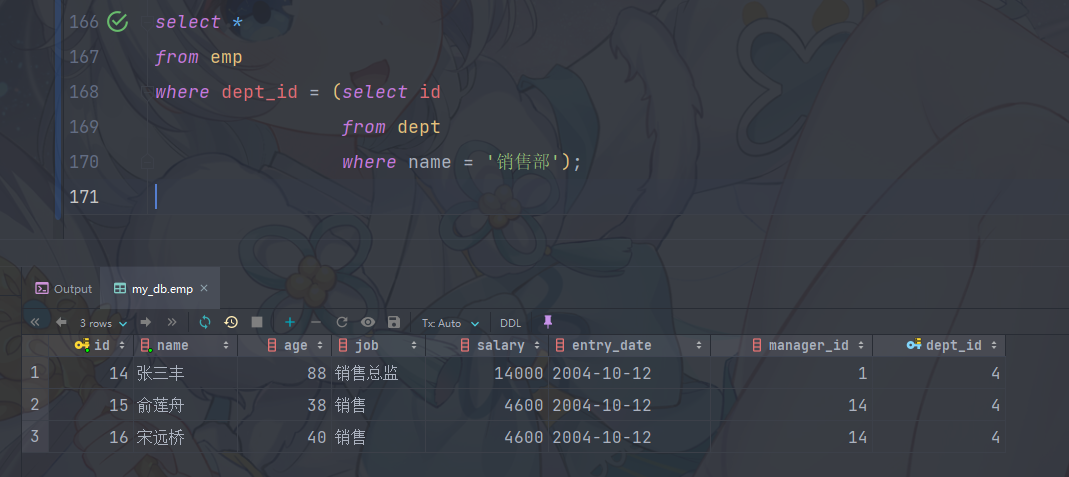
B. 查询在 “方东白” 入职之后的员工信息
完成这个需求时,我们可以将需求分解为两步:
①. 查询 方东白 的入职日期
select entry_date
from emp
where name = '方东白';
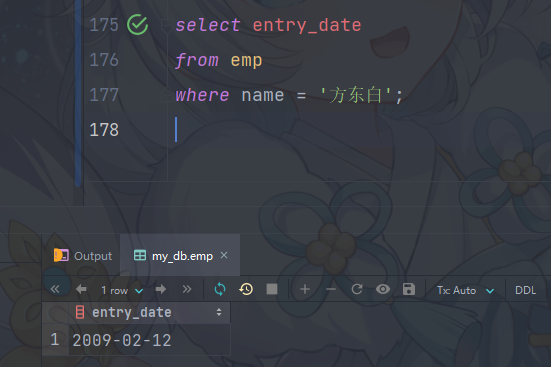
②. 查询指定入职日期之后入职的员工信息
select *
from emp
where entry_date > (select entry_date
from emp
where name = '方东白');
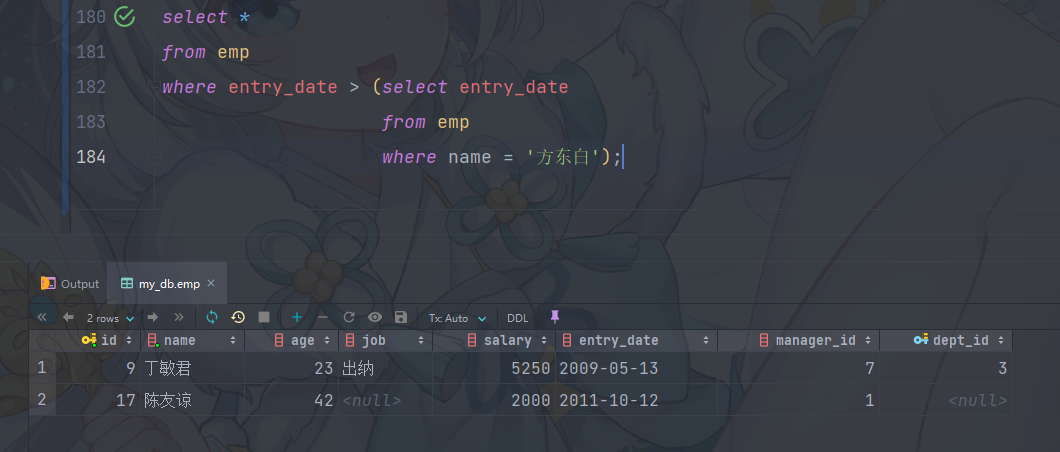
列子查询
子查询返回的结果是一列(可以是多行),这种子查询称为列子查询。
常用的操作符:IN
、NOT IN
、 ANY
、SOME
、 ALL
操作符 |
描述 |
IN |
在指定的集合范围之内,多选一 |
NOT IN |
不在指定的集合范围之内 |
ANY |
子查询返回列表中,有任意一个满足即可 |
SOME |
与ANY等同,使用SOME的地方都可以使用ANY |
ALL |
子查询返回列表的所有值都必须满足 |
案例
A. 查询 “销售部” 和 “市场部” 的所有员工信息
分解为以下两步:
①. 查询 “销售部” 和 “市场部” 的部门ID
select id
from dept
where name in ('销售部', '市场部');
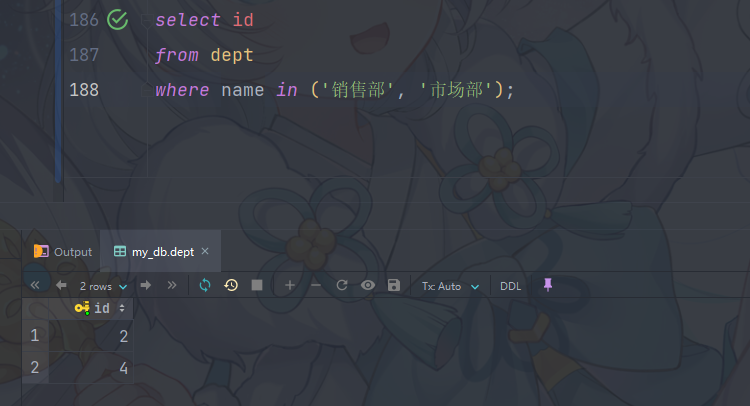
②. 根据部门ID, 查询员工信息
select *
from emp
where dept_id in (select id
from dept
where name in ('销售部', '市场部'));
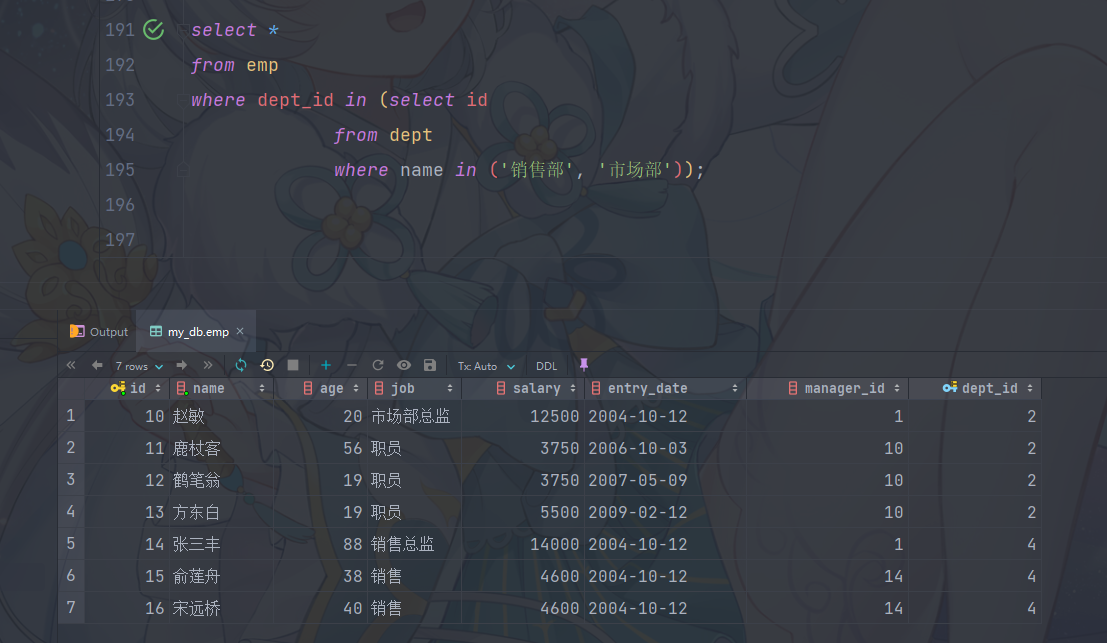
B. 查询比 财务部 所有人工资都高的员工信息
分解为以下三步:
①. 查询所有 财务部 的部门ID
select id
from dept
where name = '财务部';
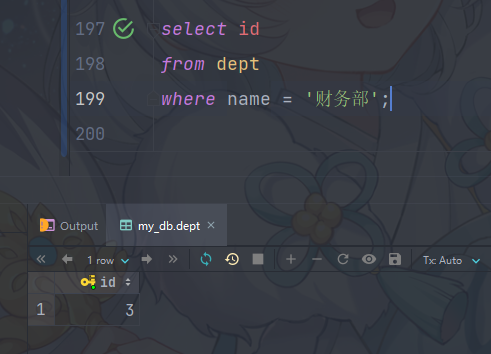
②. 查询所有 财务部 人员工资
select salary
from emp
where dept_id = (select id
from dept
where name = '财务部');
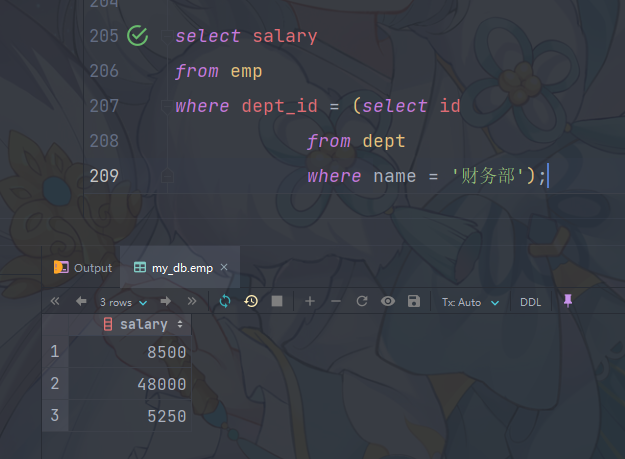
③. 比 财务部 所有人工资都高的员工信息
select *
from emp
where salary > all (select salary
from emp
where dept_id = (select id
from dept
where name = '财务部'));
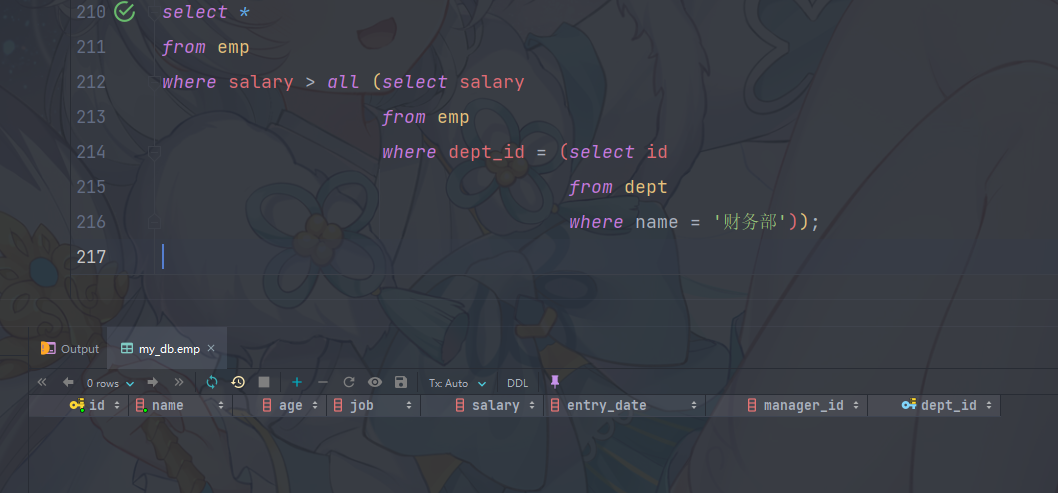
C. 查询比研发部其中任意一人工资高的员工信息
分解为以下三步:
①. 查询 研发部 部门ID
select id
from dept
where name = '研发部';
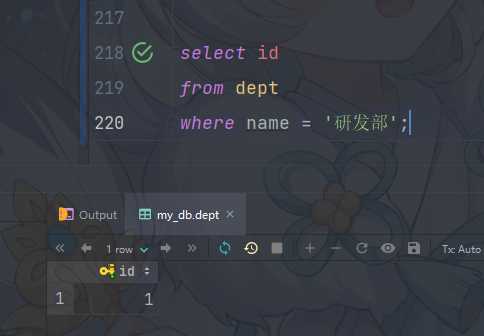
②. 查询 研发部 所有人工资
select salary
from emp
where dept_id = (select id
from dept
where name = '研发部');
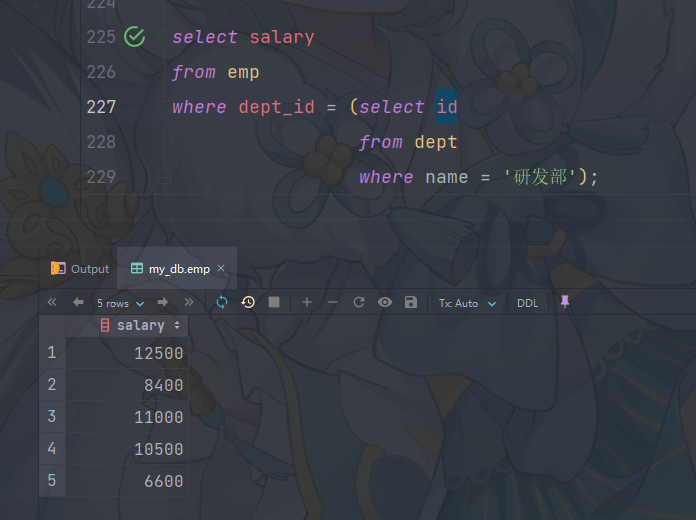
③. 比 研发部 其中任意一人工资高的员工信息
select *
from emp
where salary > any (select salary
from emp
where dept_id = (select id
from dept
where name = '研发部'));
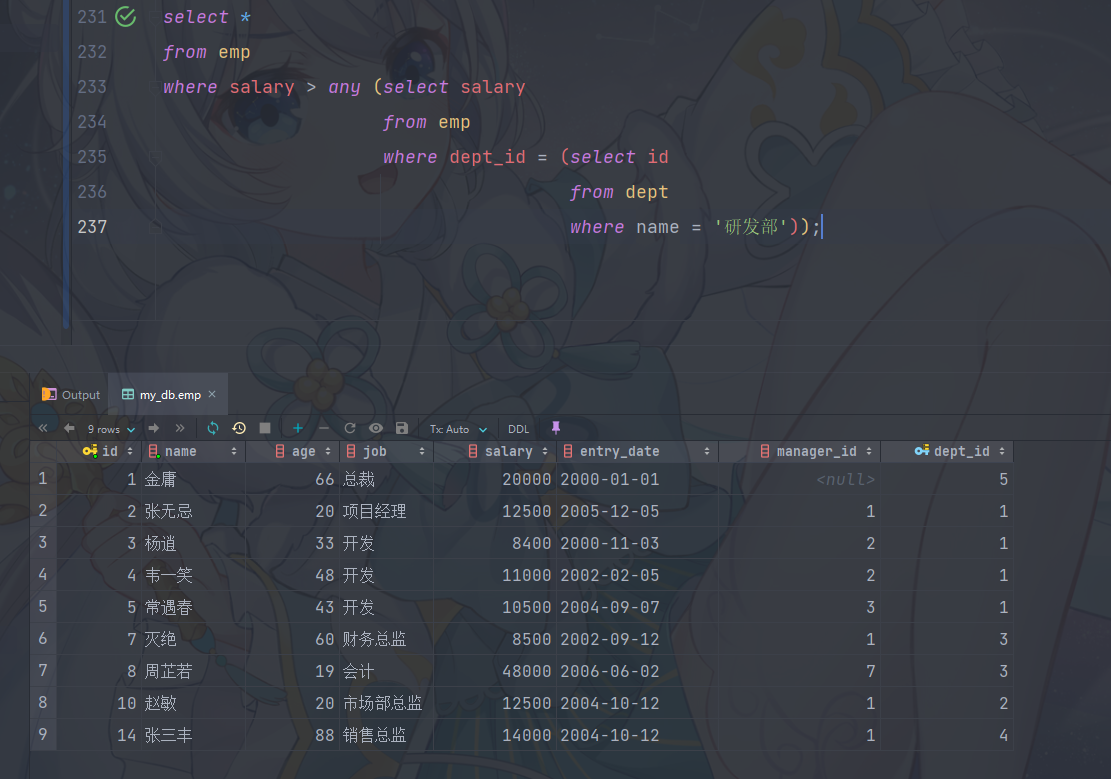
行子查询
子查询返回的结果是一行(可以是多列),这种子查询称为行子查询。
常用的操作符:=
、<>
、IN
、NOT IN
案例
查询与 “张无忌” 的薪资及直属领导相同的员工信息 ;
这个需求同样可以拆解为两步进行:
①. 查询 “张无忌” 的薪资及直属领导
select salary, manager_id
from emp
where name = '张无忌';
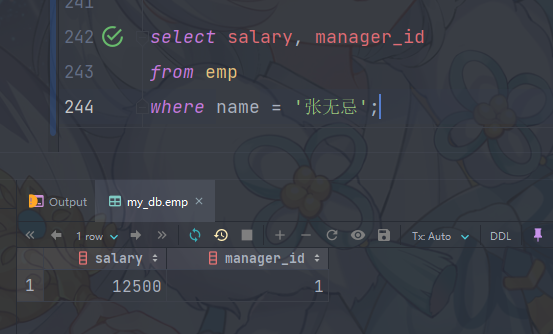
②. 查询与 “张无忌” 的薪资及直属领导相同的员工信息
select *
from emp
where (salary, manager_id) = (select salary, manager_id
from emp
where name = '张无忌');
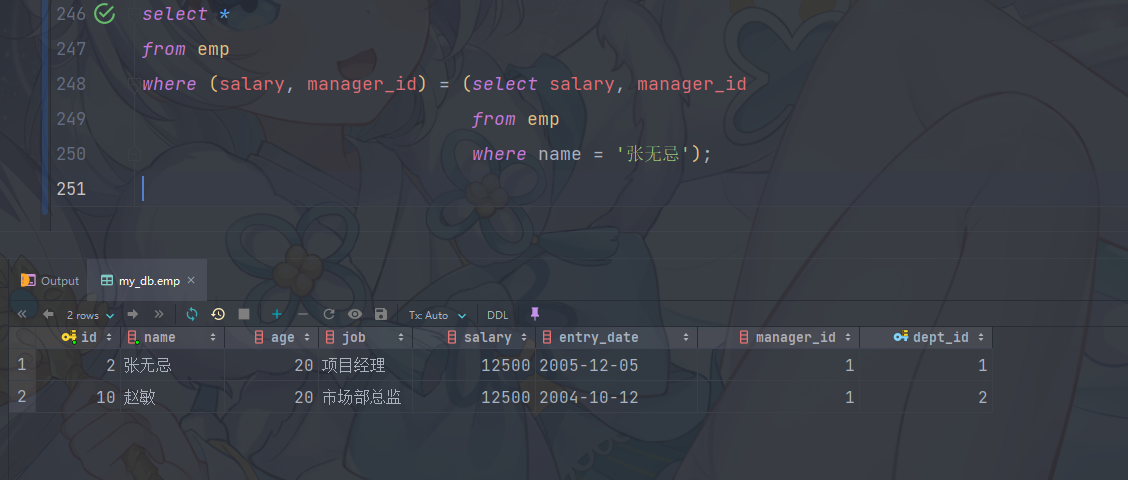
表子查询
子查询返回的结果是多行多列,这种子查询称为表子查询。
常用的操作符:IN
案例
A. 查询与 “鹿杖客” , “宋远桥” 的职位和薪资相同的员工信息
分解为两步执行:
①. 查询 “鹿杖客” , “宋远桥” 的职位和薪资
select job, salary
from emp
where name in ('鹿杖客', '宋远桥');
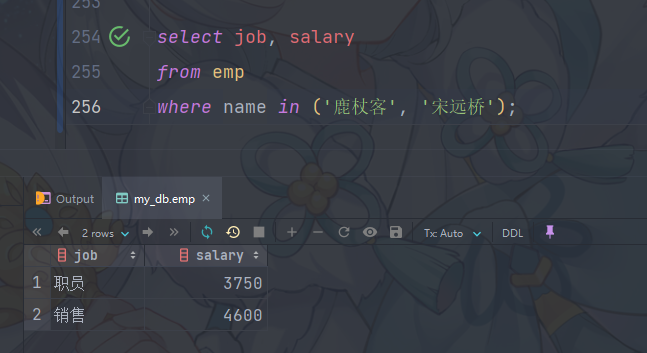
②. 查询与 “鹿杖客” , “宋远桥” 的职位和薪资相同的员工信息
select *
from emp
where (job, salary) in (select job, salary
from emp
where name in ('鹿杖客', '宋远桥'));
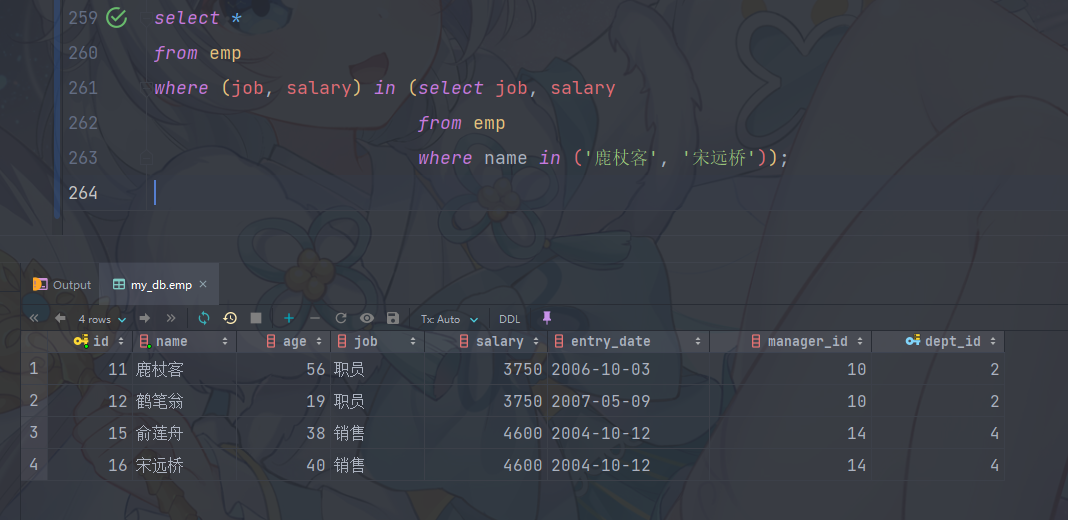
B. 查询入职日期是 “2006-01-01” 之后的员工信息 , 及其部门信息
分解为两步执行:
①. 入职日期是 “2006-01-01” 之后的员工信息
select *
from emp
where entry_date > '2006-01-01';
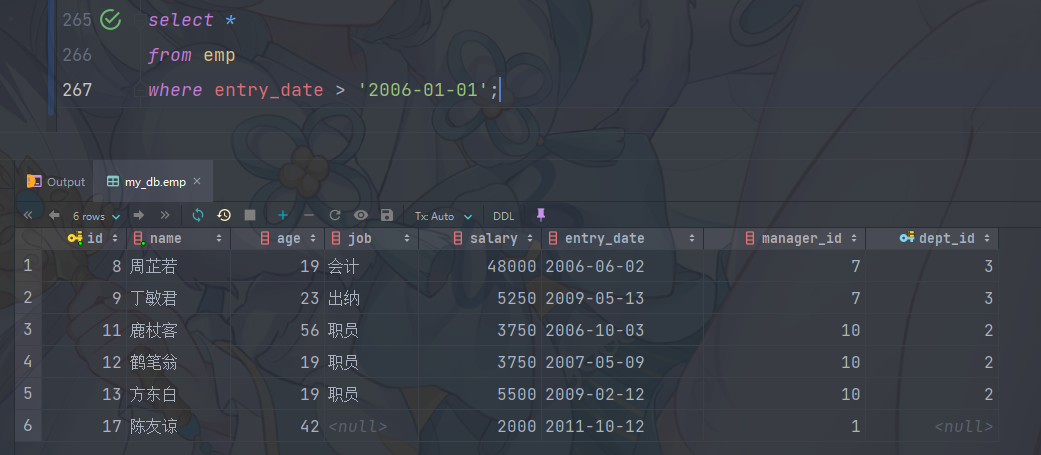
②. 查询这部分员工, 对应的部门信息;
select e.*, d.*
from (select *
from emp
where entry_date > '2006-01-01') e
left join dept d on e.dept_id = d.id;
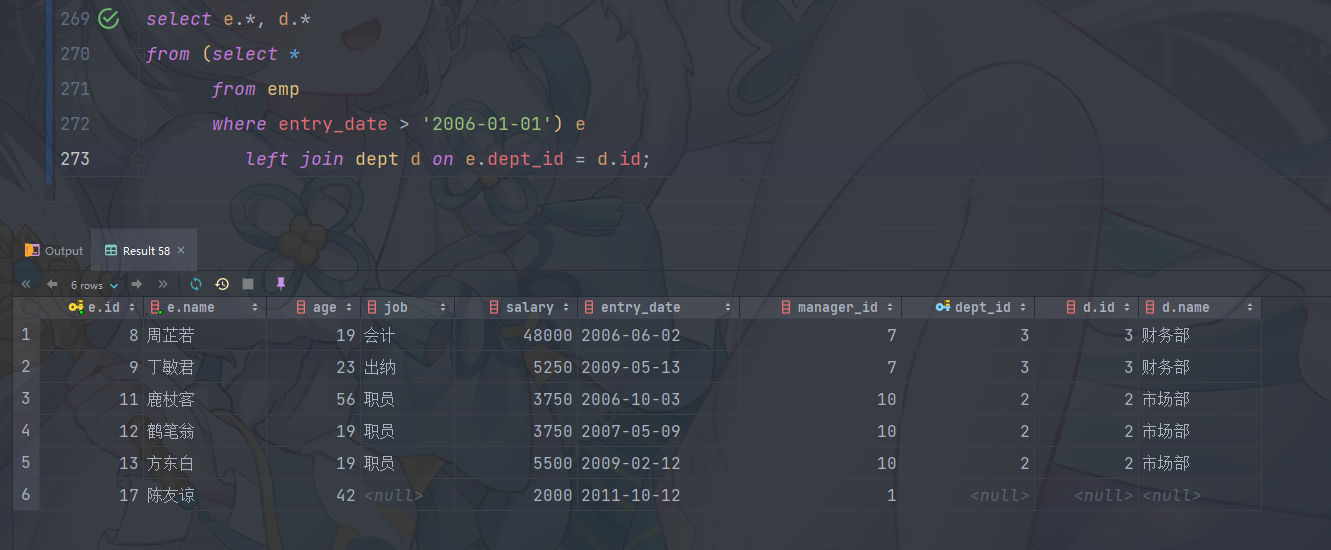
多表查询案例
准备员工、部门以及薪资等级这三张数据表,其中两张表在上面已经创建过来,这里不赘述。
-- 创建薪资等级表
create table salary_grade
(
grade int comment '薪资等级',
low_salary int comment '该等级最低薪资',
high_salary int comment '该等级最高薪资'
) comment '薪资等级表';
-- 添加数据
insert into salary_grade
values (1, 0, 3000);
insert into salary_grade
values (2, 3001, 5000);
insert into salary_grade
values (3, 5001, 8000);
insert into salary_grade
values (4, 8001, 10000);
insert into salary_grade
values (5, 10001, 15000);
insert into salary_grade
values (6, 15001, 20000);
insert into salary_grade
values (7, 20001, 25000);
insert into salary_grade
values (8, 25001, 30000);
准备学生、课程学生选课这三张表。
drop table if exists student_course;
drop table if exists student;
drop table if exists course;
create table student
(
id int auto_increment primary key comment '主键ID',
name varchar(10) comment '姓名',
no varchar(10) comment '学号'
) comment '学生表';
insert into student
values (null, '黛绮丝', '2000100101'),
(null, '谢逊','2000100102'),
(null, '殷天正', '2000100103'),
(null, '韦一笑', '2000100104');
create table course
(
id int auto_increment primary key comment '主键ID',
name varchar(10) comment '课程名称'
) comment '课程表';
insert into course
values (null, 'Java'),
(null, 'PHP'),
(null, 'MySQL'),
(null, 'Hadoop');
create table student_course
(
id int auto_increment comment '主键' primary key,
student_id int not null comment '学生ID',
course_id int not null comment '课程ID',
constraint fk_courseId foreign key (course_id) references course (id),
constraint fk_studentId foreign key (student_id) references student (id)
) comment '学生课程中间表';
insert into student_course
values (null, 1, 1),
(null, 1, 2),
(null, 1, 3),
(null, 2, 2),
(null, 2, 3),
(null, 3, 4);
1). 查询员工的姓名、年龄、职位、部门信息 (隐式内连接)
select e.name, e.age, e.job, d.name
from emp e,
dept d
where e.dept_id = d.id;
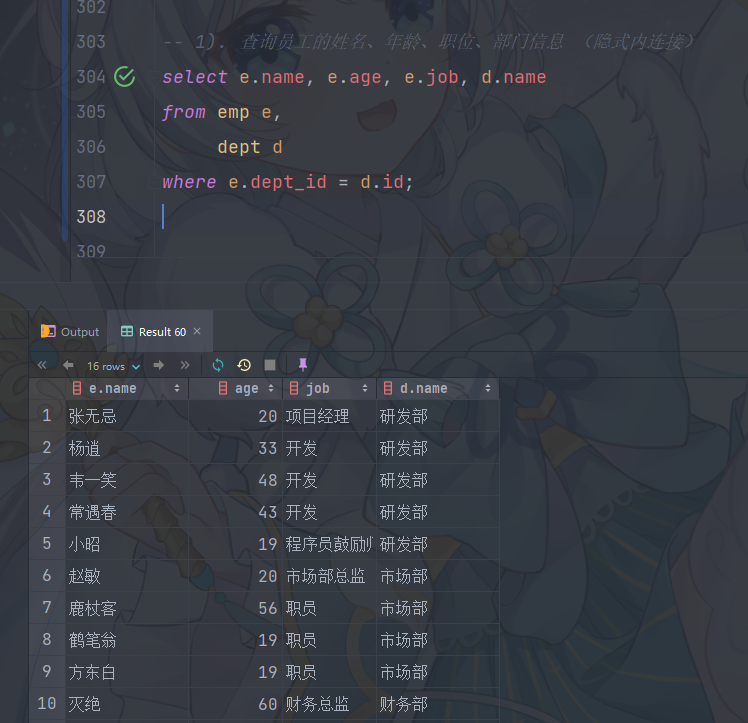
2). 查询年龄小于30岁的员工的姓名、年龄、职位、部门信息(显式内连接)
select e.name, e.age, e.job, d.name
from emp e
inner join dept d on e.dept_id = d.id
where e.age < 30;
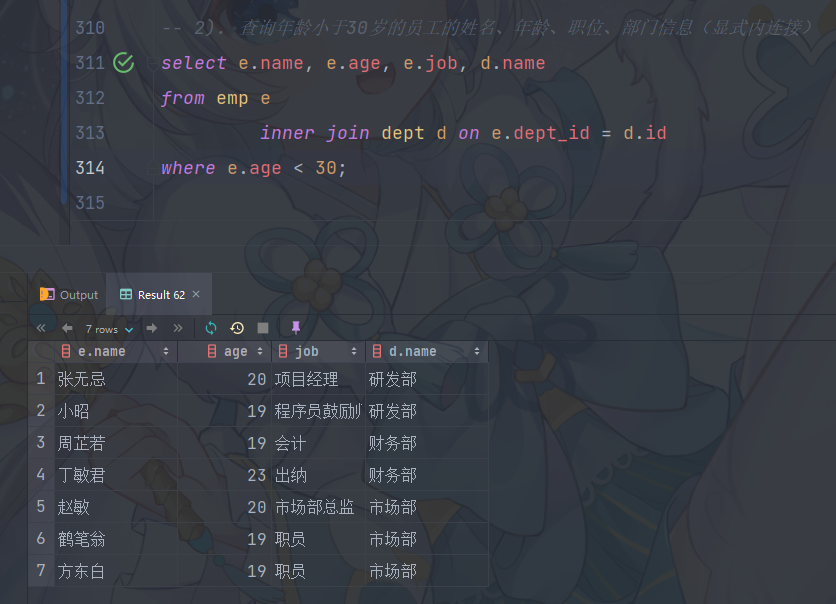
3). 查询拥有员工的部门ID、部门名称
select distinct d.id, d.name
from emp e
inner join dept d on d.id = e.dept_id;
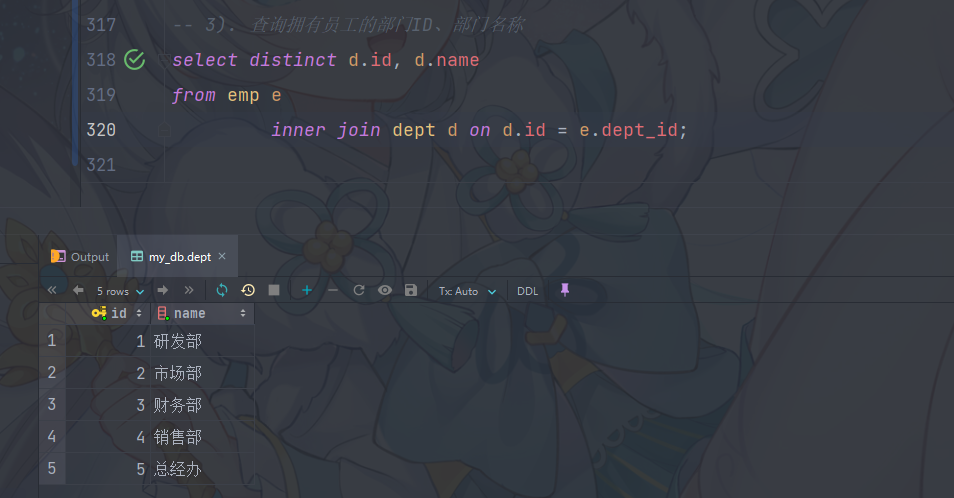
4). 查询所有年龄大于40岁的员工, 及其归属的部门名称; 如果员工没有分配部门, 也需要展示出来(外连接)
select e.*, d.name
from emp e
left join dept d on d.id = e.dept_id
where e.age > 40;
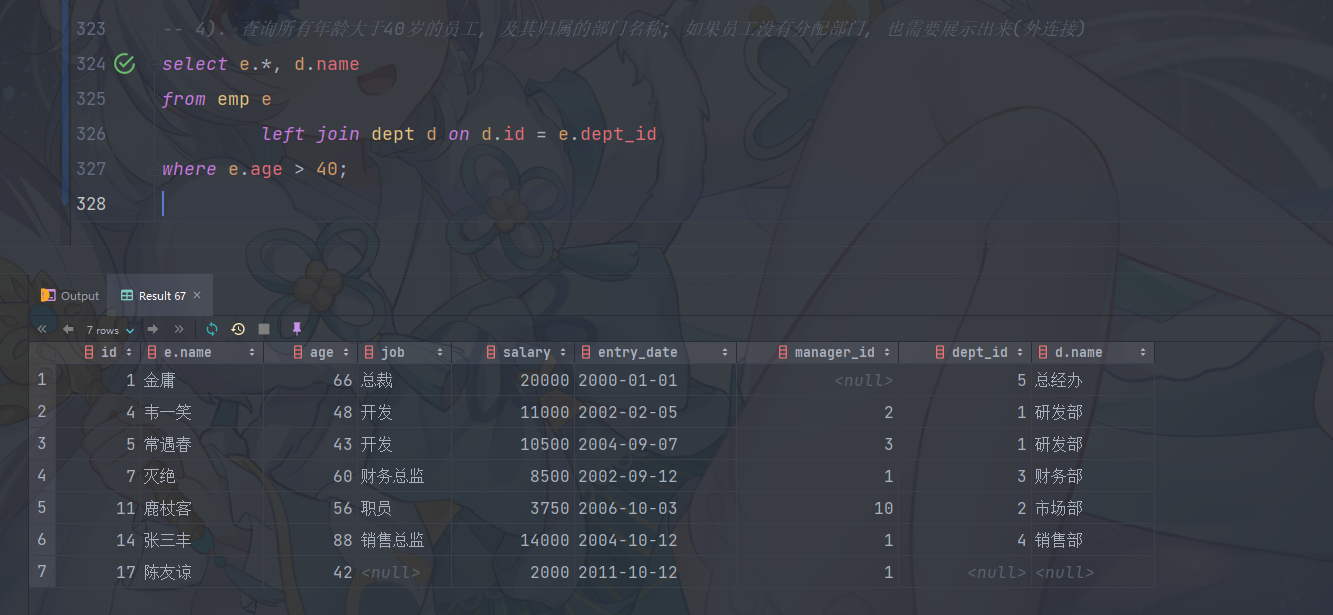
5). 查询所有员工的工资等级
select e.name, e.salary, sg.grade
from emp e
inner join salary_grade sg on e.salary between sg.low_salary and sg.high_salary;
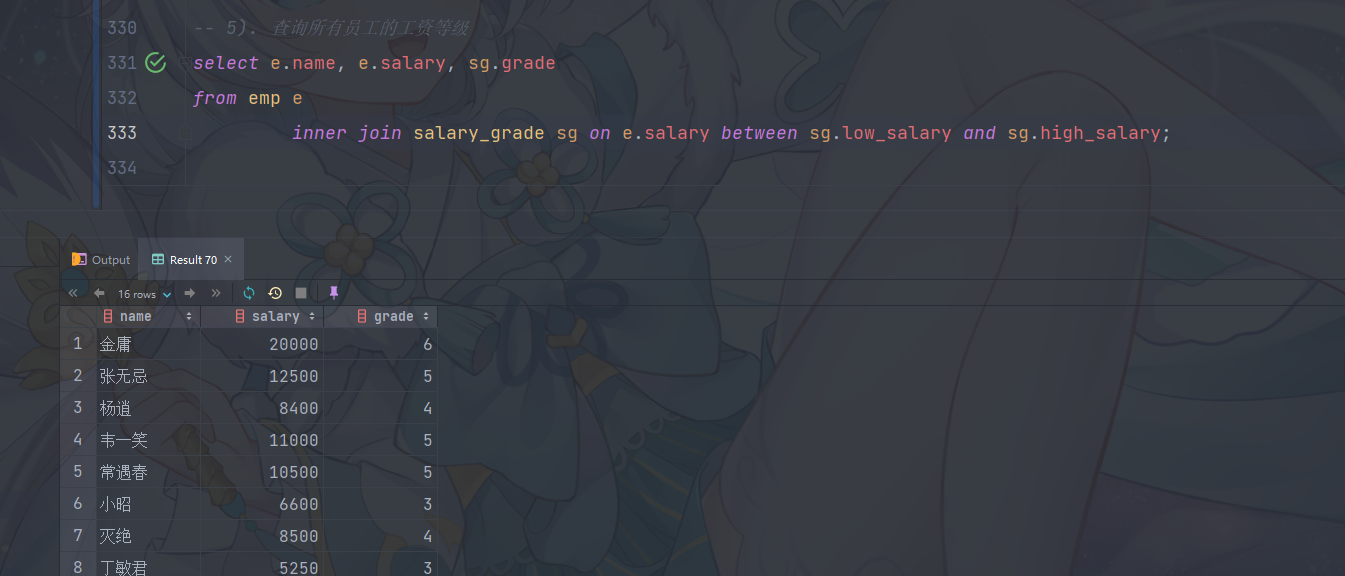
6). 查询 “研发部” 所有员工的信息及 工资等级
select e.*, sg.grade
from emp e
inner join dept d on e.dept_id = d.id
inner join salary_grade sg on e.salary between sg.low_salary and sg.high_salary
where d.name = '研发部';
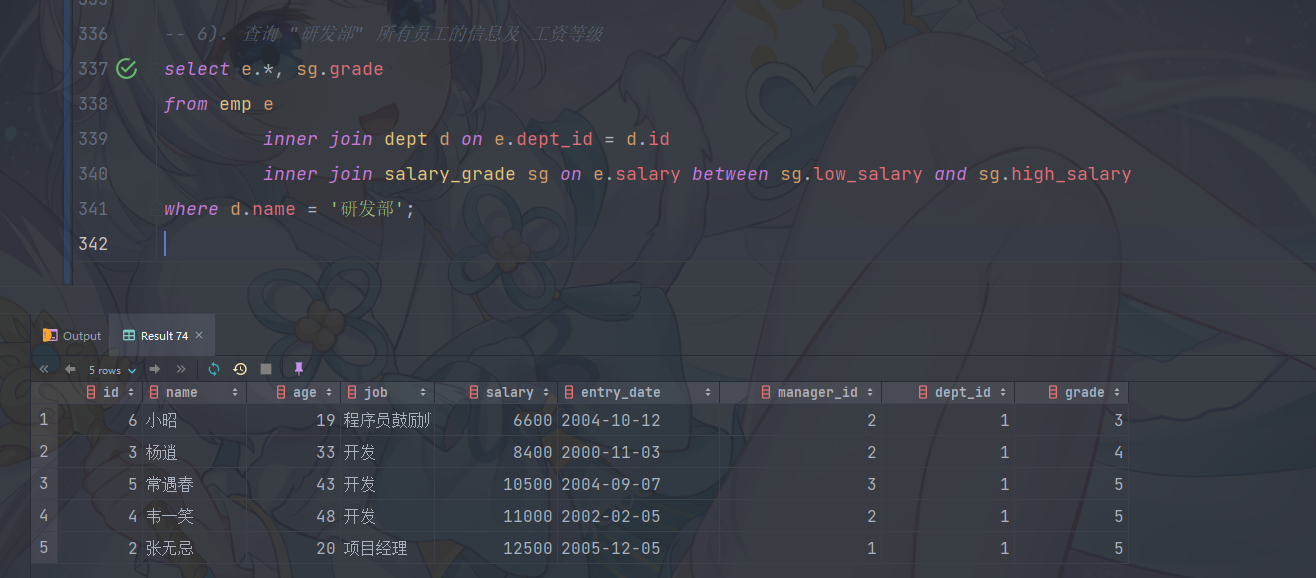
7). 查询 “研发部” 员工的平均工资
select avg(e.salary)
from emp e
inner join dept d on e.dept_id = d.id
where d.name = '研发部';
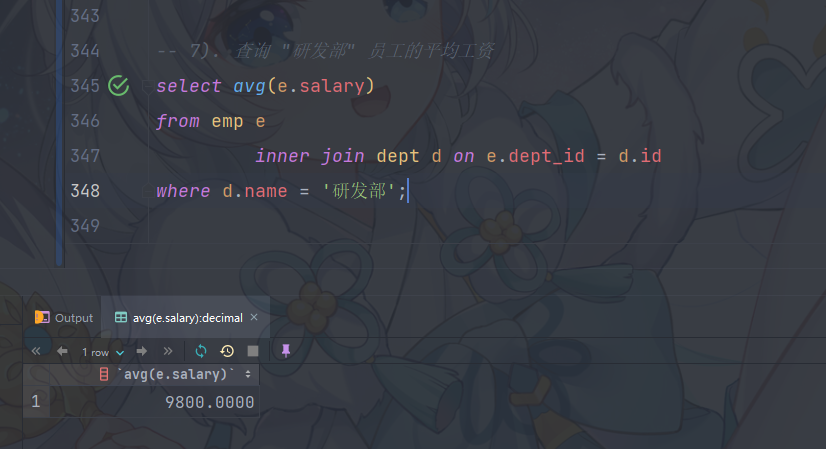
8). 查询工资比 “灭绝” 高的员工信息。
select *
from emp
where salary > (select salary
from emp
where name = '灭绝');
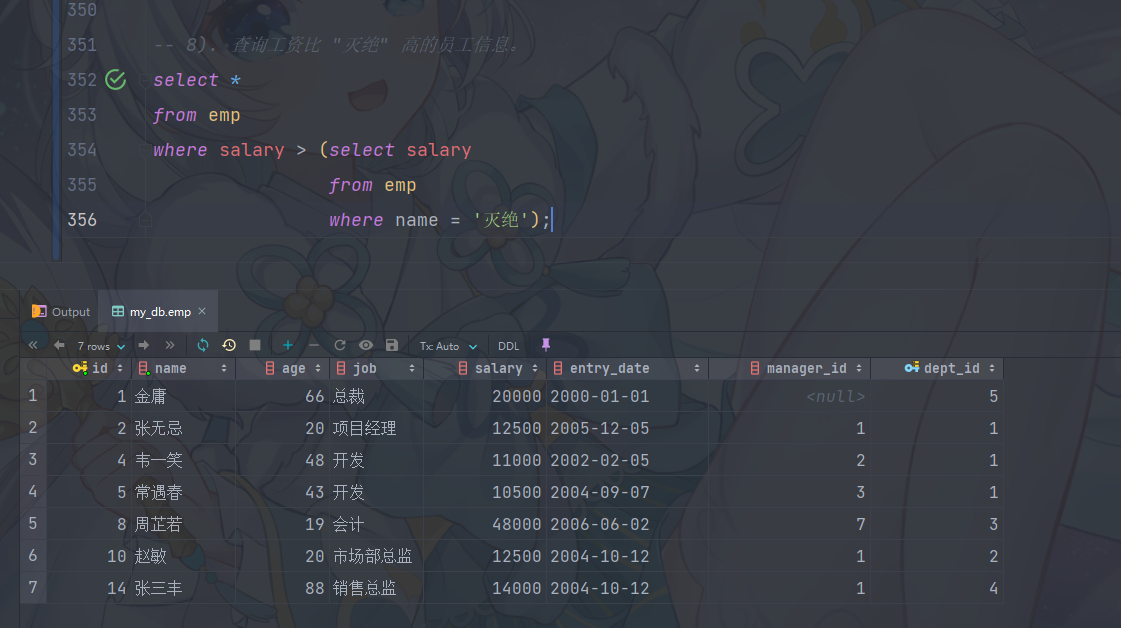
9). 查询比平均薪资高的员工信息
select *
from emp
where salary > (select avg(salary)
from emp);
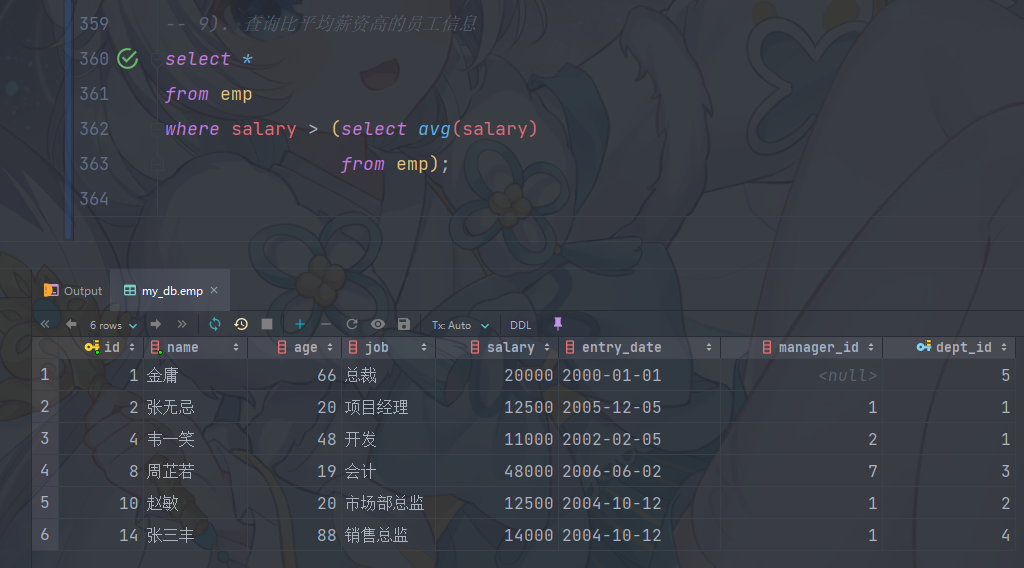
10). 查询低于本部门平均工资的员工信息
①. 查询指定部门平均薪资
select avg(e1.salary)
from emp e1
where e1.dept_id = 1;
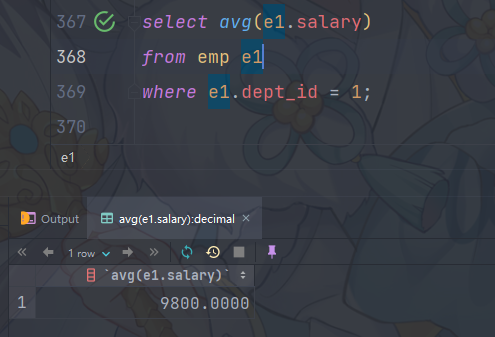
②. 查询低于本部门平均工资的员工信息
select *
from emp e2
where e2.salary < (select avg(e1.salary)
from emp e1
where e1.dept_id = e2.dept_id);
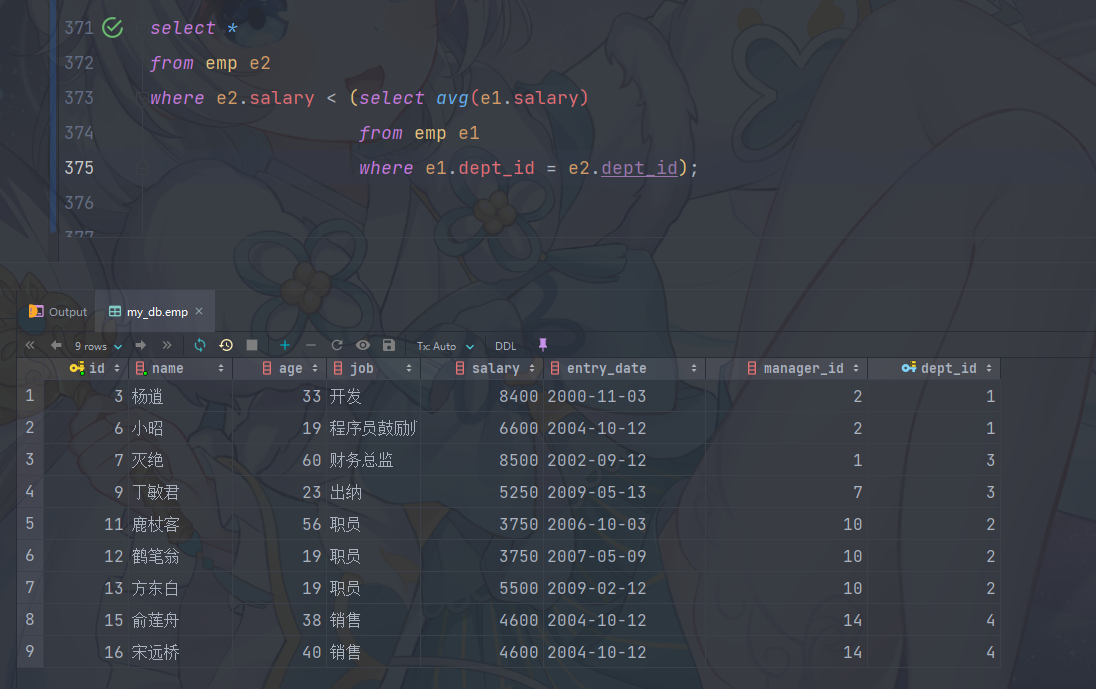
为了验证结果是否正确,平均薪资也展示出来。
select *,
(select avg(e1.salary)
from emp e1
where e1.dept_id = e2.dept_id) '平均薪资'
from emp e2
where e2.salary < (select avg(e1.salary)
from emp e1
where e1.dept_id = e2.dept_id);
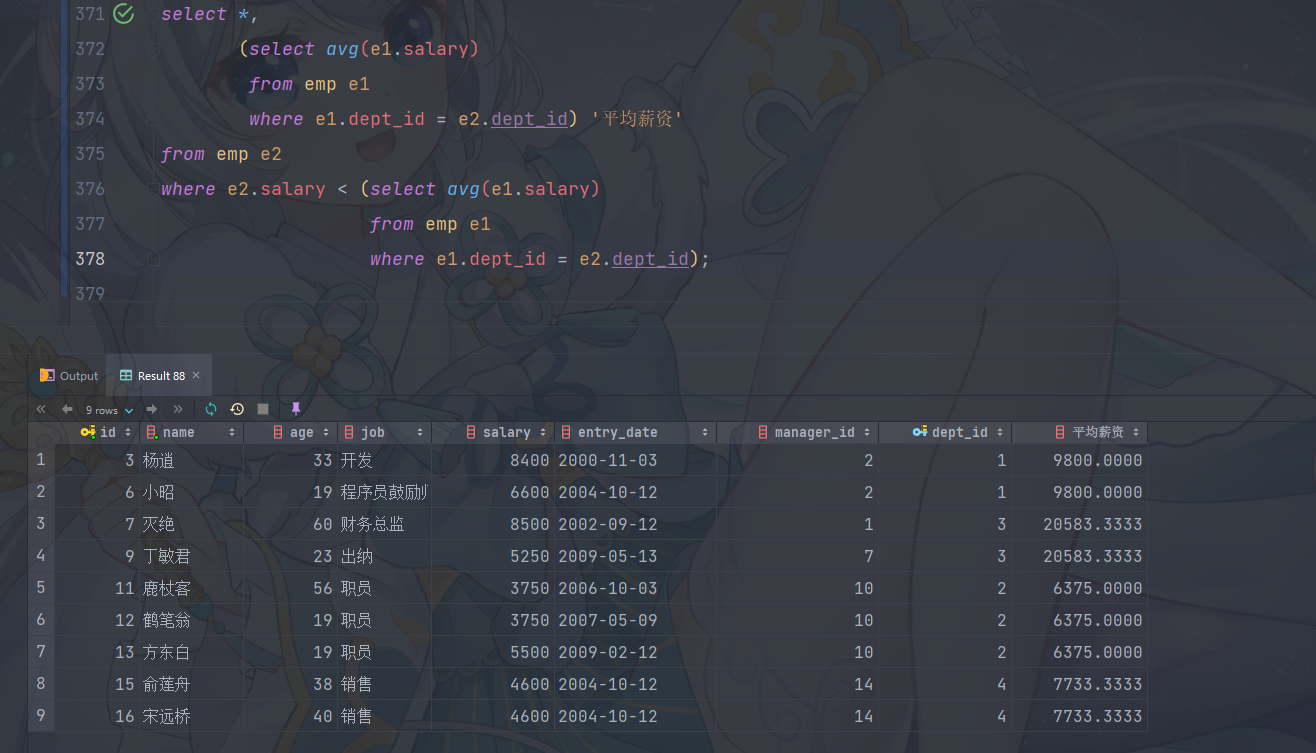
11). 查询所有的部门信息, 并统计部门的员工人数
select d.id,
d.name,
(select count(e.dept_id)
from emp e
where e.dept_id = d.id) '人数'
from dept d;
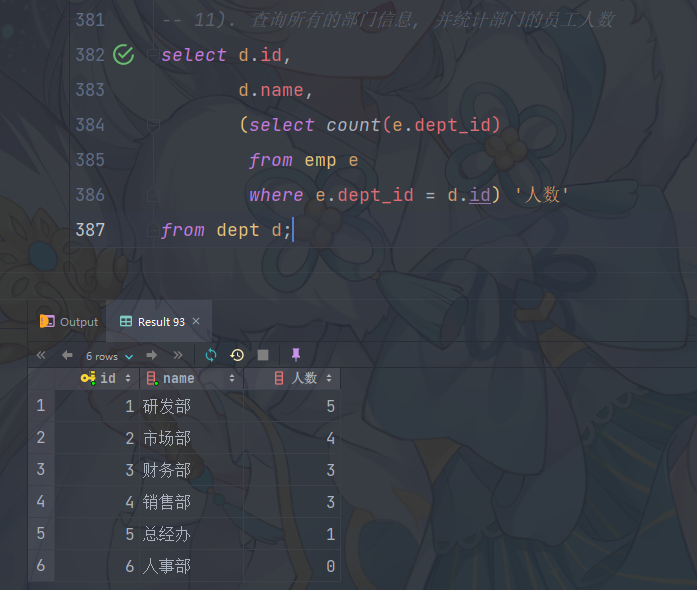
12). 查询所有学生的选课情况, 展示出学生名称, 学号, 课程名称
select s.name, s.no, c.name
from student s
inner join student_course sc on s.id = sc.student_id
inner join course c on sc.course_id = c.id;
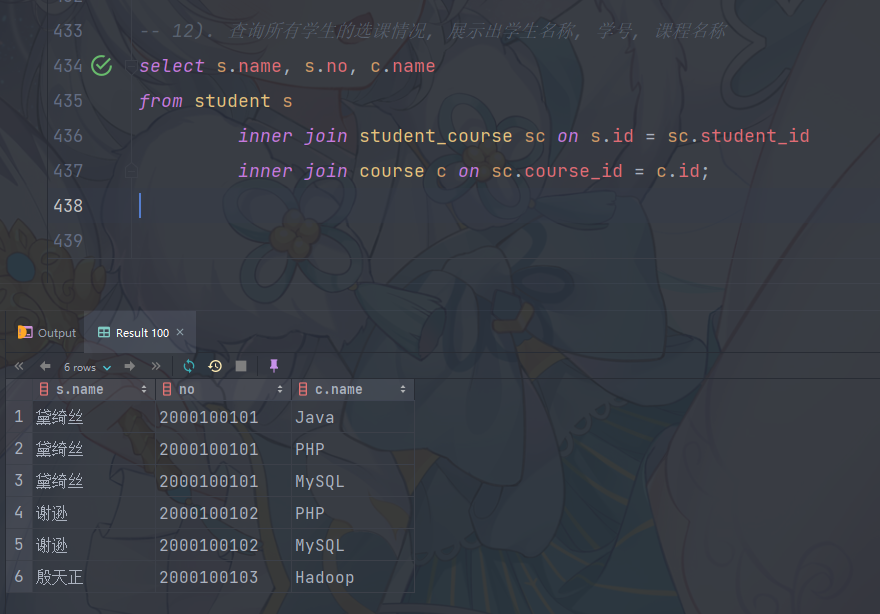