目录
1、三角函数 Trigonometric functions
1.1、 cos() 函数
1.2 sin() 正弦函数
1.3、 tan() 正切函数
1.4、 acos() 反余弦函数
1.5、asin() 反正弦函数
1.6、atan() 反正切函数
1.7、atan2() 带两个参数的反正切函数
2、双曲函数 Hyperbolic functions
2.1、双曲余弦函数
2.2、双曲正弦函数
2.3、双曲正切函数
3、指数函数与对数函数 Exponential and logarithmic functions
3.1、exp () 指数函数,以 e 为底数
3.2、frexp(param,n) 二进制浮点数表示方法 x=param*2^n
3.3、log(x) x的自然对数 (Natural logarithm of x)
3.4、log10() 常用对数,以10为底 ( Common logarithm of x )
3.5、modf() 返回x的小数部分,其符号与x相同 ,但是参数中可以添加整数部分的变量( The fractional part of x, with the same sign)
3.6、exp2() 返回2的x次方,2 raised to the power of x.
3.7、log2() x的二进制对数( The binary logarithm of x)
4、幂函数 Power functions
4.1、pow(base, power) 幂函数 The result of raising base to the power exponent
4.2、sqrt(x) 计算x的平方根
4.3、cbrt(x) 计算x的立方根
4.4、hypot(x,y) 计算直角三角形的斜边 ( The square root of (x^2+y^2) )
5、误差与伽马函数 Error and gamma functions
5.1、误差函数erf(x)
5.2、余差函数erfc(x) erfc(x) = 1-erf(x) 误差函数的补函数
5.3、tgamma(x) 伽马函数 ( the gamma function )
5.4、lgamma(x) log伽马函数 ( log-gamma function )
6、四舍五入与余数函数Rounding and remainder functions
6.1、ceil(x) x上取整函数
6.2、floor(x) x的下取整函数
6.3、fmod(y, x) y/x的余数
6.4、round(x) x的四舍五入值
7、绝对值、最小、最大 Absolute、Minimum, maximum
7.1、fabs(x) x的绝对值函数
7.2、abs(x) x的绝对值
7.3、fmax(x, y) 两个参数中的最大值 (The maximum numeric value of its arguments. Values among which the function selects a maximum )
7.4、fmin(x, y) 两个参数中的最小值
1、三角函数 Trigonometric functions
1.1、 cos() 函数
/* cos example */
#include <stdio.h> /* printf */
#include <math.h> /* cos */
#define PI 3.14159265
int main ()
{
double param, result;
param = 60.0;
result = cos ( param * PI / 180.0 );
printf ("The cosine of %f degrees is %f.\n", param, result );
return 0;
}
1.2 sin() 正弦函数
/* sin example */
#include <stdio.h> /* printf */
#include <math.h> /* sin */
#define PI 3.14159265
int main ()
{
double param, result;
param = 30.0;
result = sin (param*PI/180);
printf ("The sine of %f degrees is %f.\n", param, result );
return 0;
}
1.3、 tan() 正切函数
/* tan example */
#include <stdio.h> /* printf */
#include <math.h> /* tan */
#define PI 3.14159265
int main ()
{
double param, result;
param = 45.0;
result = tan ( param * PI / 180.0 );
printf ("The tangent of %f degrees is %f.\n", param, result );
return 0;
}
1.4、 acos() 反余弦函数
/* acos example */
#include <stdio.h> /* printf */
#include <math.h> /* acos */
#define PI 3.14159265
int main ()
{
double param, result;
param = 0.5;
result = acos (param) * 180.0 / PI;
printf ("The arc cosine of %f is %f degrees.\n", param, result);
return 0;
}
1.5、asin() 反正弦函数
/* asin example */
#include <stdio.h> /* printf */
#include <math.h> /* asin */
#define PI 3.14159265
int main ()
{
double param, result;
param = 0.5;
result = asin (param) * 180.0 / PI;
printf ("The arc sine of %f is %f degrees\n", param, result);
return 0;
}
1.6、atan() 反正切函数
/* atan example */
#include <stdio.h> /* printf */
#include <math.h> /* atan */
#define PI 3.14159265
int main ()
{
double param, result;
param = 1.0;
result = atan (param) * 180 / PI;
printf ("The arc tangent of %f is %f degrees\n", param, result );
return 0;
}
1.7、atan2() 带两个参数的反正切函数
/* atan2 example */
#include <stdio.h> /* printf */
#include <math.h> /* atan2 */
#define PI 3.14159265
int main ()
{
double x, y, result;
x = -10.0;
y = 10.0;
result = atan2 (y,x) * 180 / PI;
printf ("The arc tangent for (x=%f, y=%f) is %f degrees\n", x, y, result );
return 0;
}
2、双曲函数 Hyperbolic functions
2.1、双曲余弦函数
/* cosh example */
#include <stdio.h> /* printf */
#include <math.h> /* cosh, log */
int main ()
{
double param, result;
param = log(2.0);
result = cosh (param);
printf ("The hyperbolic cosine of %f is %f.\n", param, result );
return 0;
}
2.2、双曲正弦函数
/* sinh example */
#include <stdio.h> /* printf */
#include <math.h> /* sinh, log */
int main ()
{
double param, result;
param = log(2.0);
result = sinh (param);
printf ("The hyperbolic sine of %f is %f.\n", param, result );
return 0;
}
2.3、双曲正切函数
/* tanh example */
#include <stdio.h> /* printf */
#include <math.h> /* tanh, log */
int main ()
{
double param, result;
param = log(2.0);
result = tanh (param);
printf ("The hyperbolic tangent of %f is %f.\n", param, result);
return 0;
}
3、指数函数与对数函数 Exponential and logarithmic functions
3.1、exp () 指数函数,以 e 为底数
/* exp example */
#include <stdio.h> /* printf */
#include <math.h> /* exp */
int main ()
{
double param, result;
param = 5.0;
result = exp (param);
printf ("The exponential value of %f is %f.\n", param, result );
return 0;
}
3.2、frexp(param,n) 二进制浮点数表示方法 x=param*2^n
/* frexp example */
#include <stdio.h> /* printf */
#include <math.h> /* frexp */
int main ()
{
double param, result;
int n;
param = 8.0;
result = frexp (param , &n);
printf ("%f = %f * 2^%d\n", param, result, n);
return 0;
}
3.3、log(x) x的自然对数 (Natural logarithm of x)
/* log example */
#include <stdio.h> /* printf */
#include <math.h> /* log */
int main ()
{
double param, result;
param = 5.5;
result = log (param);
printf ("log(%f) = %f\n", param, result );
return 0;
}
3.4、log10() 常用对数,以10为底 ( Common logarithm of x )
/* log10 example */
#include <stdio.h> /* printf */
#include <math.h> /* log10 */
int main ()
{
double param, result;
param = 1000.0;
result = log10 (param);
printf ("log10(%f) = %f\n", param, result );
return 0;
}
3.5、modf() 返回x的小数部分,其符号与x相同 ,但是参数中可以添加整数部分的变量( The fractional part of x, with the same sign)
/* modf example */
#include <stdio.h> /* printf */
#include <math.h> /* modf */
int main ()
{
double param, fractpart, intpart;
param = 3.14159265;
fractpart = modf (param , &intpart);
printf ("%f = %f + %f \n", param, intpart, fractpart);
return 0;
}
3.6、exp2() 返回2的x次方,2 raised to the power of x.
/* exp2 example */
#include <stdio.h> /* printf */
#include <math.h> /* exp2 */
int main ()
{
double param, result;
param = 8.0;
result = exp2 (param);
printf ("2 ^ %f = %f.\n", param, result );
return 0;
}
3.7、log2() x的二进制对数( The binary logarithm of x)
/* log2 example */
#include <stdio.h> /* printf */
#include <math.h> /* log2 */
int main ()
{
double param, result;
param = 1024.0;
result = log2 (param);
printf ("log2 (%f) = %f.\n", param, result );
return 0;
}
4、幂函数 Power functions
4.1、pow(base, power) 幂函数 The result of raising base to the power exponent
/* pow example */
#include <stdio.h> /* printf */
#include <math.h> /* pow */
int main ()
{
printf ("7 ^ 3 = %f\n", pow (7.0, 3.0) );
printf ("4.73 ^ 12 = %f\n", pow (4.73, 12.0) );
printf ("32.01 ^ 1.54 = %f\n", pow (32.01, 1.54) );
return 0;
}
4.2、sqrt(x) 计算x的平方根
/* sqrt example */
#include <stdio.h> /* printf */
#include <math.h> /* sqrt */
int main ()
{
double param, result;
param = 1024.0;
result = sqrt (param);
printf ("sqrt(%f) = %f\n", param, result );
return 0;
}
4.3、cbrt(x) 计算x的立方根
/* cbrt example */
#include <stdio.h> /* printf */
#include <math.h> /* cbrt */
int main ()
{
double param, result;
param = 27.0;
result = cbrt (param);
printf ("cbrt (%f) = %f\n", param, result);
return 0;
}
4.4、hypot(x,y) 计算直角三角形的斜边 ( The square root of (x^2+y^2) )
/* hypot example */
#include <stdio.h> /* printf */
#include <math.h> /* hypot */
int main ()
{
double leg_x, leg_y, result;
leg_x = 3;
leg_y = 4;
result = hypot (leg_x, leg_y);
printf ("%f, %f and %f form a right-angled triangle.\n",leg_x,leg_y,result);
return 0;
}
5、误差与伽马函数 Error and gamma functions
5.1、误差函数erf(x)
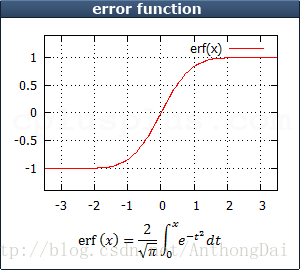
/* erf example */
#include <stdio.h> /* printf */
#include <math.h> /* erf */
int main ()
{
double param, result;
param = 1.0;
result = erf (param);
printf ("erf (%f) = %f\n", param, result );
return 0;
}
5.2、余差函数erfc(x) erfc(x) = 1-erf(x) 误差函数的补函数
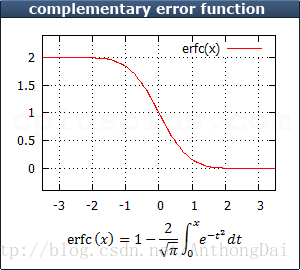
/* erfc example */
#include <stdio.h> /* printf */
#include <math.h> /* erfc */
int main ()
{
double param, result;
param = 1.0;
result = erfc (param);
printf ("erfc(%f) = %f\n", param, result );
return 0;
}
5.3、tgamma(x) 伽马函数 ( the gamma function )
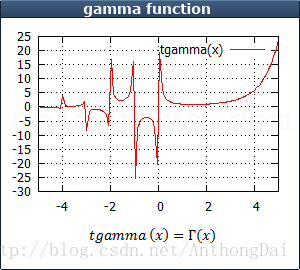
/* tgamma example */
#include <stdio.h> /* printf */
#include <math.h> /* tgamma */
int main ()
{
double param, result;
param = 0.5;
result = tgamma (param);
printf ("tgamma(%f) = %f\n", param, result );
return 0;
}
5.4、lgamma(x) log伽马函数 ( log-gamma function )
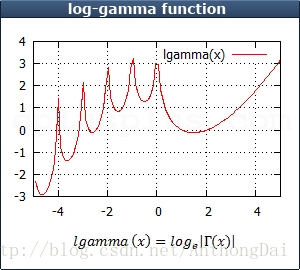
/* lgamma example */
#include <stdio.h> /* printf */
#include <math.h> /* lgamma */
int main ()
{
double param, result;
param = 0.5;
result = lgamma (param);
printf ("lgamma(%f) = %f\n", param, result );
return 0;
}
6、四舍五入与余数函数Rounding and remainder functions
6.1、ceil(x) x上取整函数
/* ceil example */
#include <stdio.h> /* printf */
#include <math.h> /* ceil */
int main ()
{
printf ( "ceil of 2.3 is %.1f\n", ceil(2.3) );
printf ( "ceil of 3.8 is %.1f\n", ceil(3.8) );
printf ( "ceil of -2.3 is %.1f\n", ceil(-2.3) );
printf ( "ceil of -3.8 is %.1f\n", ceil(-3.8) );
return 0;
}
6.2、floor(x) x的下取整函数
/* floor example */
#include <stdio.h> /* printf */
#include <math.h> /* floor */
int main ()
{
printf ( "floor of 2.3 is %.1lf\n", floor (2.3) );
printf ( "floor of 3.8 is %.1lf\n", floor (3.8) );
printf ( "floor of -2.3 is %.1lf\n", floor (-2.3) );
printf ( "floor of -3.8 is %.1lf\n", floor (-3.8) );
return 0;
}
6.3、fmod(y, x) y/x的余数
/* fmod example */
#include <stdio.h> /* printf */
#include <math.h> /* fmod */
int main ()
{
printf ( "fmod of 5.3 / 2 is %f\n", fmod (5.3,2) );
printf ( "fmod of 18.5 / 4.2 is %f\n", fmod (18.5,4.2) );
return 0;
}
6.4、round(x) x的四舍五入值
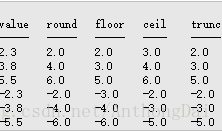
/* round vs floor vs ceil vs trunc */
#include <stdio.h> /* printf */
#include <math.h> /* round, floor, ceil, trunc */
int main ()
{
const char * format = "%.1f \t%.1f \t%.1f \t%.1f \t%.1f\n";
printf ("value\tround\tfloor\tceil\ttrunc\n");
printf ("-----\t-----\t-----\t----\t-----\n");
printf (format, 2.3,round( 2.3),floor( 2.3),ceil( 2.3),trunc( 2.3));
printf (format, 3.8,round( 3.8),floor( 3.8),ceil( 3.8),trunc( 3.8));
printf (format, 5.5,round( 5.5),floor( 5.5),ceil( 5.5),trunc( 5.5));
printf (format,-2.3,round(-2.3),floor(-2.3),ceil(-2.3),trunc(-2.3));
printf (format,-3.8,round(-3.8),floor(-3.8),ceil(-3.8),trunc(-3.8));
printf (format,-5.5,round(-5.5),floor(-5.5),ceil(-5.5),trunc(-5.5));
return 0;
}
7、绝对值、最小、最大 Absolute、Minimum, maximum
7.1、fabs(x) x的绝对值函数
/* fabs example */
#include <stdio.h> /* printf */
#include <math.h> /* fabs */
int main ()
{
printf ("The absolute value of 3.1416 is %f\n", fabs (3.1416) );
printf ("The absolute value of -10.6 is %f\n", fabs (-10.6) );
return 0;
}
7.2、abs(x) x的绝对值
// cmath's abs example
#include <iostream> // std::cout
#include <cmath> // std::abs
int main ()
{
std::cout << "abs (3.1416) = " << std::abs (3.1416) << '\n';
std::cout << "abs (-10.6) = " << std::abs (-10.6) << '\n';
return 0;
}
7.3、fmax(x, y) 两个参数中的最大值 (The maximum numeric value of its arguments. Values among which the function selects a maximum )
/* fmax example */
#include <stdio.h> /* printf */
#include <math.h> /* fmax */
int main ()
{
printf ("fmax (100.0, 1.0) = %f\n", fmax(100.0,1.0));
printf ("fmax (-100.0, 1.0) = %f\n", fmax(-100.0,1.0));
printf ("fmax (-100.0, -1.0) = %f\n", fmax(-100.0,-1.0));
return 0;
}
7.4、fmin(x, y) 两个参数中的最小值
/* fmin example */
#include <stdio.h> /* printf */
#include <math.h> /* fmin */
int main ()
{
printf ("fmin (100.0, 1.0) = %f\n", fmin(100.0,1.0));
printf ("fmin (-100.0, 1.0) = %f\n", fmin(-100.0,1.0));
printf ("fmin (-100.0, -1.0) = %f\n", fmin(-100.0,-1.0));
return 0;
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)