页面效果图展示:
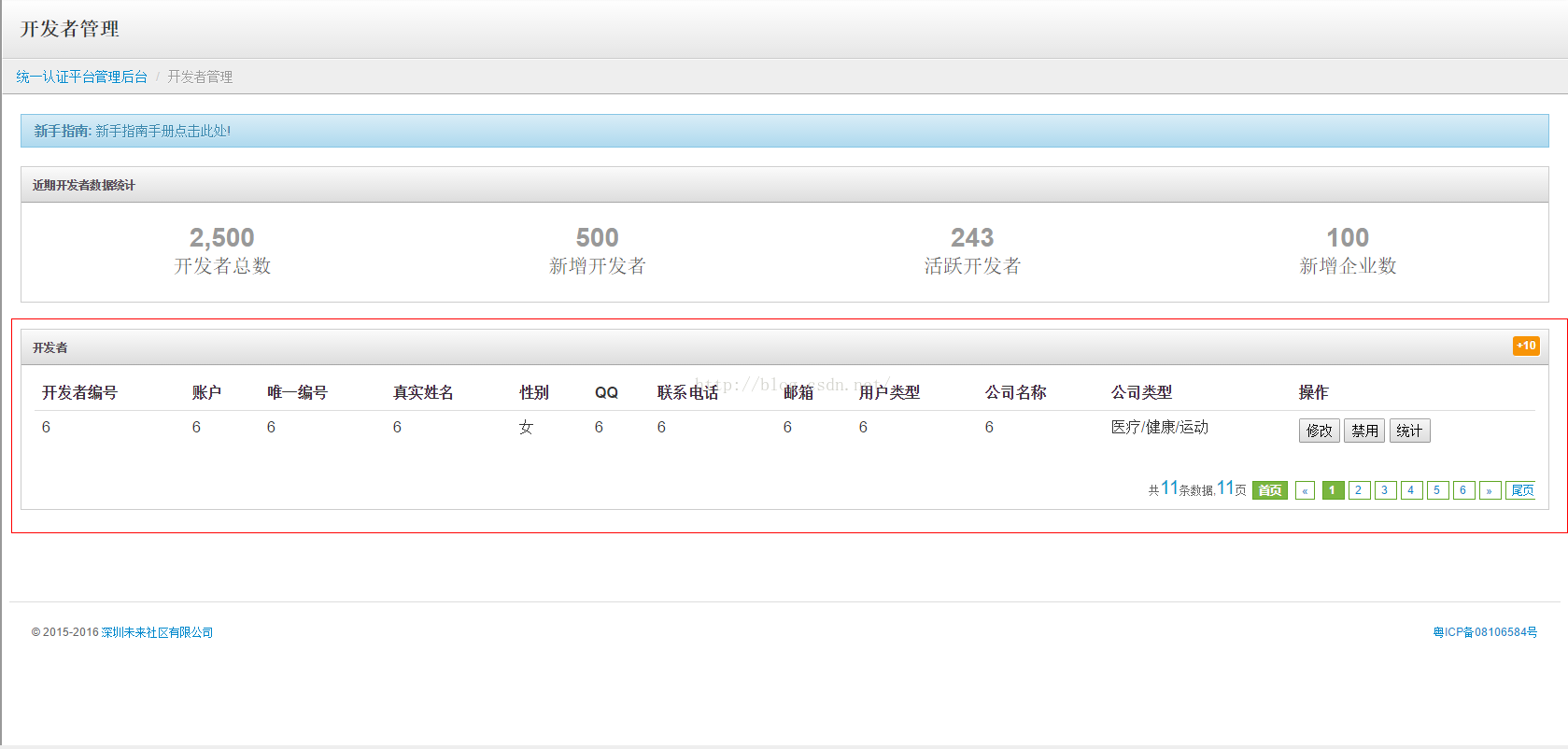
分页工具类:Pagination
package com.wlsq.kso.util;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* 储存分页处理工具类 在调用此类的方法之前需设置总页数(即得先从数据库查询到相应数据的数据量)
*
* @author ahomeeye
* @version 1.0
*/
public class Pagination implements Serializable {
private static final long serialVersionUID = 1L;
private int start; // start表示当前页开始的记录数,start=每页行数*(当前页数-1)
private int end; // 当前页结束的记录行数
private int totalCount; // 总行数
private int rowsPerPage = 1; // 每页行数,默认10
private int currentPage; // 当前页数
private int pageListSize = 6;// 页码列表大小,默认9
private List<Integer> pageNumList = new ArrayList<Integer>();
public Pagination() {
start = 0;
end = 0;
currentPage = 1;
this.totalCount = 0;
}
public Pagination(int totalCount) {
start = 0;
end = 0;
currentPage = 1;
this.totalCount = totalCount;
}
public Pagination(int totalCount, int numPerPage) {
start = 0;
end = 0;
this.totalCount = totalCount;
currentPage = 1;
if (numPerPage > 0) {
rowsPerPage = numPerPage;
}
}
/**
* 执行翻页动作
*
* @param currentPage
* 要翻到的目标页码
* @return 返回翻页对象
*/
public Pagination doPagination(int currentPage) {
gotoPage(currentPage);
return this;
}
// 设置起始数
public int getStart() {
start = rowsPerPage * (currentPage - 1);
return start;
}
// 得到起始数
public void setStart(int start) {
if (start < 0) {
this.start = 0;
} else if (start >= this.totalCount) {
this.start = this.totalCount - 1;
} else {
this.start = start;
}
}
// 设置当前页的最后一行的在总记录中的顺序(从0开始)
public void setEnd(int end) {
this.end = end;
}
// 得到当前页的最后一行的在总记录中的顺序(从0开始)
public int getEnd() {
if (rowsPerPage * currentPage > this.totalCount) {
end = this.totalCount - 1;
} else {
end = rowsPerPage * currentPage - 1;
}
return end;
}
// 以下4个方法供控制器(struts)调用
// 判断能否到第一页;只要能到上一页,肯定就有第一页
public boolean firstEnable() {
return previousEnable();
}
// 判断能否到上一页
public boolean previousEnable() {
return currentPage > 1;// 只要不是第一页,就能到上一页
}
// 判断能否到下一页
public boolean nextEnable() {
return currentPage * rowsPerPage < this.totalCount;
}
// 判断能否到最后一页;只要有下一页,就肯定有最后一页.
public boolean lastEnable() {
return nextEnable();
}
// 跳到第一页
public void firstPage() {
currentPage = 1;
}
// 跳到上一页
public void previousPage(int cPage) {
currentPage = (cPage - 1) > 0 ? (cPage - 1) : 1;
}
// 跳到下一页
public void nextPage(int cPage) {
currentPage = cPage + 1;
if (currentPage * rowsPerPage > this.totalCount) {
lastPage();
}
}
// 跳到最后一页
public void lastPage() {
if (this.totalCount % rowsPerPage == 0) {
currentPage = this.totalCount / rowsPerPage;
} else {
currentPage = this.totalCount / rowsPerPage + 1;
}
}
// 跳到指定的某一页
public void gotoPage(int pageNumber) {
if (pageNumber <= 1) {
currentPage = 1;
} else if (getTotalCount() < this.getRowsPerPage()) {
currentPage = 1;
} else if (pageNumber * rowsPerPage >= this.totalCount) {
lastPage();
} else {
currentPage = pageNumber;
}
}
// 设置总行数
public void setTotalCount(int totalCount) {
this.totalCount = totalCount;
}
// 得到总行数
public int getTotalCount() {
return totalCount;
}
// 设置每页行数
public void setRowsPerPage(int rowsPerPage) {
this.rowsPerPage = rowsPerPage;
}
// 得到每页行数
public int getRowsPerPage() {
return rowsPerPage;
}
// 得到总页数
public int getPages() {
if (this.totalCount % rowsPerPage == 0)
return this.totalCount / rowsPerPage;
else
return this.totalCount / rowsPerPage + 1;
}
// 得到当前页数
public int getCurrentPage() {
return currentPage;
}
// 设置当前页数
public void setCurrentPage(int currentPage) {
this.currentPage = currentPage;
}
public int getPageListSize() {
return pageListSize;
}
// 设置页码列表大小
public void setPageListSize(int pageListSize) {
this.pageListSize = pageListSize;
}
// 得到页面列表
public List<Integer> getPageNumList() {
this.pageNumList.removeAll(this.pageNumList);// 设置之前先清空
int totalPage = getPages();
if (totalPage > this.pageListSize) {
int halfSize = this.pageListSize / 2;
int first = 1;
int end = 1;
if (this.currentPage - halfSize < 1) { // 当前页靠近最小数1
first = 1;
end = this.pageListSize;
} else if (totalPage - this.currentPage < halfSize) { // 当前页靠近最大数
first = totalPage - this.pageListSize + 1;
end = totalPage;
} else {
first = this.currentPage - halfSize;
end = this.currentPage + halfSize;
}
for (int i = first; i <= end; i++) {
this.pageNumList.add(i);
}
} else {
for (int i = 0; i < totalPage; i++) {
this.pageNumList.add(i + 1);
}
}
return pageNumList;
}
}
分页样式和js文件(page.css/page.js)
page.js
$(function(){
$(document).on("click",".page_num,.next_page,.prev_page,.first_page,.last_page",function(){
var $self = $(this);
if($self.parent().attr("class") == 'disabled'){
return false;
}
$("#page_current").val($self.attr("data-pnum"));
$("#page_form").submit();
});
});
page.css
.pages{ width:100.5%; text-align:right; padding:10px 0; clear:both;}
.pages a,.pages b{ font-size:12px; font-family:Arial, Helvetica,
sans-serif; margin:0 2px;}
.pages a,.pages b{ border:1px solid #5FA623; background:#fff; padding:2px
6px; text-decoration:none}
.pages b,.pages a:hover{ background:#7AB63F; color:#fff;}
后台页面逻辑代码和数据库查询配置文件:
controller 文件
/*开发者查询列表*/
@RequestMapping(value="/select.action" )
public ModelAndView Select(HttpServletRequest request){
ModelAndView modelAndView = new ModelAndView();
int pageSize = Integer
.parseInt(request.getParameter("pageSize") == null ? "1"
: request.getParameter("pageSize"));
int pageNum = Integer
.parseInt(request.getParameter("pageNum") == null ? "1"
: request.getParameter("pageNum"));
Map<String, Object> maps = new HashMap<String, Object>();
maps.put("pageSize", pageSize);
maps.put("pageNum", (pageNum-1) * pageSize);
Developer developer = new Developer();
List<Developer> develpers = developerService.selectByDeveloper(maps);
int count = developerService.selectCountDevelopers();
Pagination page = new Pagination(count);
page.setCurrentPage(pageNum);
modelAndView.addObject("pnums", page.getPageNumList());
modelAndView.addObject("currentPage", pageNum);
modelAndView.addObject("pnext_flag", page.nextEnable());
modelAndView.addObject("plast_flag", page.lastEnable());
page.lastPage();
modelAndView.addObject("last_page", page.getCurrentPage());
modelAndView.addObject("count", count);
modelAndView.addObject("pageCount", page.getPages());
if(develpers != null){
modelAndView.setViewName("backstage/home");
modelAndView.addObject("developers", develpers);
}
return modelAndView;
}
mybatis配置文件(service 文件名与mybatis 配置文件名一致,这是我们公司老大提供的)
<select id="selectByDeveloper" resultMap="extendResultMap">
select
*
from developer d,company_type c,s_province s,s_city city,s_district t
where 1=1 and d.company_type_id = c.company_type_id and s.pid=d.pid and city.cid=d.cid and t.did=d.did
limit #{maps.pageNum},#{maps.pageSize}
</select>
项目页面源码:
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>统一认证平台管理后台</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<link rel="stylesheet" type="text/css"
href="<%=basePath%>static/lib/bootstrap/css/bootstrap.css">
<link rel="stylesheet" type="text/css"
href="<%=basePath%>static/lib/bootstrap/css/page.css">
<link rel="stylesheet" type="text/css"
href="<%=basePath%>static/stylesheets/theme.css">
<link rel="stylesheet"
href="<%=basePath%>static/lib/font-awesome/css/font-awesome.css">
<script src="<%=basePath%>static/lib/jquery-1.7.2.min.js"
type="text/javascript"></script>
<script src="<%=basePath%>static/lib/page.js" type="text/javascript"></script>
<!-- Demo page code -->
<style type="text/css">
#line-chart {
height: 300px;
width: 800px;
margin: 0px auto;
margin-top: 1em;
}
.brand {
font-family: georgia, serif;
}
.brand .first {
color: #ccc;
font-style: italic;
}
.brand .second {
color: #fff;
font-weight: bold;
}
</style>
<style>
.pages{ width:100.5%; text-align:right; padding:10px 0; clear:both;}
.pages a,.pages b{ font-size:12px; font-family:Arial, Helvetica,
sans-serif; margin:0 2px;}
.pages a,.pages b{ border:1px solid #5FA623; background:#fff; padding:2px
6px; text-decoration:none}
.pages b,.pages a:hover{ background:#7AB63F; color:#fff;}
</style>
<!-- Le HTML5 shim, for IE6-8 support of HTML5 elements -->
<!--[if lt IE 9]>
<script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
<!-- Le fav and touch icons -->
<link rel="shortcut icon" href="../assets/ico/favicon.ico">
<link rel="apple-touch-icon-precomposed" sizes="144x144"
href="../assets/ico/apple-touch-icon-144-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="114x114"
href="../assets/ico/apple-touch-icon-114-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="72x72"
href="../assets/ico/apple-touch-icon-72-precomposed.png">
<link rel="apple-touch-icon-precomposed"
href="../assets/ico/apple-touch-icon-57-precomposed.png">
</head>
<!--[if lt IE 7 ]> <body class="ie ie6"> <![endif]-->
<!--[if IE 7 ]> <body class="ie ie7 "> <![endif]-->
<!--[if IE 8 ]> <body class="ie ie8 "> <![endif]-->
<!--[if IE 9 ]> <body class="ie ie9 "> <![endif]-->
<!--[if (gt IE 9)|!(IE)]><!-->
<body class="">
<jsp:include page="top.jsp" />
<jsp:include page="menu.jsp" />
<!--内容 -->
<div class="content">
<!--内容标题 -->
<div class="header">
<!--<div class="stats">
<p class="stat"><span class="number">53</span>tickets</p>
<p class="stat"><span class="number">27</span>tasks</p>
<p class="stat"><span class="number">15</span>waiting</p>
</div>-->
<!--当前功能模块 -->
<h1 class="page-title">开发者管理</h1>
</div>
<ul class="breadcrumb">
<li><a href="index.html">统一认证平台管理后台</a> <span class="divider">/</span>
</li>
<li class="active">开发者管理</li>
</ul>
<div class="container-fluid">
<div class="row-fluid">
<!--菜单项一 -->
<div class="row-fluid">
<div class="alert alert-info">
<!-- <button type="button" class="close" data-dismiss="alert">×</button> -->
<strong>新手指南:</strong> 新手指南手册点击此处!
</div>
<div class="block">
<a href="#page-stats" class="block-heading" data-toggle="collapse">近期开发者数据统计</a>
<div id="page-stats" class="block-body collapse in">
<div class="stat-widget-container">
<div class="stat-widget">
<div class="stat-button">
<p class="title">2,500</p>
<p class="detail">开发者总数</p>
</div>
</div>
<div class="stat-widget">
<div class="stat-button">
<p class="title">500</p>
<p class="detail">新增开发者</p>
</div>
</div>
<div class="stat-widget">
<div class="stat-button">
<p class="title">243</p>
<p class="detail">活跃开发者</p>
</div>
</div>
<div class="stat-widget">
<div class="stat-button">
<p class="title">100</p>
<p class="detail">新增企业数</p>
</div>
</div>
</div>
</div>
</div>
</div>
<!--菜单项二 -->
<div class="row-fluid">
<div class="block span12">
<a href="#tablewidget" class="block-heading"
data-toggle="collapse">开发者<span class="label label-warning">+10</span>
</a>
<div id="tablewidget" class="block-body collapse in">
<table class="table">
<thead>
<tr>
<th>开发者编号</th>
<th>账户</th>
<th>唯一编号</th>
<th>真实姓名</th>
<th>性别</th>
<th>QQ</th>
<th>联系电话</th>
<th>邮箱</th>
<th>用户类型</th>
<th>公司名称</th>
<th>公司类型</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach var="developer" items="${developers}">
<tr>
<td><c:out value="${developer.acctId}" />
</td>
<td><c:out value="${developer.username}" />
</td>
<td><c:out value="${developer.openId}" />
</td>
<td><c:out value="${developer.acctRealNm}" />
</td>
<td><c:choose>
<c:when test="${developer.acctSex == 0}">
<c:out value="男" />
</c:when>
<c:otherwise>
<c:out value="女" />
</c:otherwise>
</c:choose></td>
<td><c:out value="${developer.acctQq}" />
</td>
<td><c:out value="${developer.acctPhone}" />
</td>
<td><c:out value="${developer.acctEmail}" />
</td>
<td><c:out value="${developer.userType}" />
</td>
<td><c:out value="${developer.companyName}" />
</td>
<td><c:out
value="${developer.companyType.companyTypeName}" />
</td>
<td><a name="toUpdate" ids="${developer.openId }"
href="javascript:;"><input type="submit" value="修改"
class="button" /> </a> <a name="toDisable"
ids="${developer.openId }" href="javascript:;"><input
type="submit" value="禁用" class="button" /> </a> <a
name="toCount" ids="${developer.openId }"
href="javascript:;"><input type="submit" value="统计"
class="button" /> </a></td>
</tr>
</c:forEach>
</tbody>
</table>
<form action="${pageContext.request.contextPath }/developer/select.action" method="get" id="page_form">
<input type="hidden" id="page_current" name="pageNum" value="${currentPage }">
</form>
<div class="pages">
<span style="font-size: 12px;color: #666;">共<font
style="color: #09c;font-size: 20px;">${count }</font>条数据,<font
style="color: #09c;font-size: 20px;">${pageCount }</font>页</span>
<c:if test="${currentPage != 1 }">
<b href="javascript:void(0)" class="first_page" data-pnum="1">首页<span class="sr-only"></span></b
<a aria-label="Previous" href="javascript:void(0)" class="prev_page" data-pnum="${currentPage -1 }"><span aria-hidden="true">«</span></a>
</c:if>
<c:if test="${currentPage == 1 }">
<b href="javascript:void(0)" class="first_page" >首页<span class="sr-only"></span></b>
<a aria-label="Previous" href="javascript:void(0)"><span aria-hidden="true">«</span></a>
</c:if>
<c:forEach items="${pnums }" var="pa">
<c:if test="${pa == currentPage }">
<b href="javascript:void(0)"
class="page_num" data-pnum="${pa }">${pa }<span
class="sr-only"></span>
</b>
</c:if>
<c:if test="${pa != currentPage }">
<a href="javascript:void(0)" class="page_num"
data-pnum="${pa }">${pa }<span class="sr-only"></span>
</a>
</c:if>
</c:forEach>
<c:if test="${pnext_flag }">
<a href="javascript:void(0)" aria-label="Next"
class="next_page" data-pnum="${currentPage + 1 }"><span
aria-hidden="true">»</span>
</a>
</c:if>
<c:if test="${!pnext_flag }">
<a href="javascript:void(0)"
aria-label="Next"><span aria-hidden="true">»</span>
</a>
</c:if>
<c:if test="${plast_flag }">
<a href="javascript:void(0)" class="last_page"
data-pnum="${last_page }">尾页<span class="sr-only"></span>
</a>
</c:if>
<c:if test="${!plast_flag }">
<a href="javascript:void(0)" data-pnum="${last_page }"
class="last_page">尾页<span class="sr-only"></span>
</a>
</c:if>
</div>
</div>
</div>
</div>
<!--底部菜单栏 -->
<footer>
<hr>
<!-- Purchase a site license to remove this link from the footer: http://www.portnine.com/bootstrap-themes -->
<p class="pull-right">
<a href="#" target="_blank">粤ICP备08106584号</a>
</p>
<p>
© 2015-2016 <a href="#" target="_blank">深圳未来社区有限公司</a>
</p>
</footer>
</div>
</div>
</div>
<script type="text/javascript"
src="<%=basePath%>static/lib/bootstrap/js/bootstrap.js"></script>
<script type="text/javascript">
/* $("[rel=tooltip]").tooltip();
$(function() {
$('.demo-cancel-click').click(function(){return false;});
}); */
$(function() {
//用户禁用操作
$("[name='toDisable']").click(function() {
if (confirm("确定要禁用该用户吗?")) {
var id = $(this).attr("ids");
//动态用户禁用
$.ajax({
url : "developer/update.action",
data : "open_id=" + id + "&open_type=update",
dataType : "json",
type : "POST",
async : true,
success : function(data) {
var ajaxobj = eval(data);
if (ajaxobj.error_code == "108") {
//刷新当前页面
window.location.reload();
} else {
alert("用户数据禁用失败!")
}
},
error : function() {
alert("用户数据禁用失败!")
}
});
}
});
//用户统计
$("[name='toCount']")
.click(
function() {
var id = $(this).attr("ids");
window.location.href = "/admin_back/toAddAgentBank.action?id="
+ id;
});
//更新操作
$("[name='toUpdate']")
.click(
function() {
var id = $(this).attr("ids");
window.location.href = "developer/develop_update.action?openId="
+ id;
});
})
</script>
</body>
</html>