目录
前言
一、lucky-canvas介绍
二、lucky-canvas使用(Vue3)
1. 安装
2. 注册
3. 使用
三、九宫格为例的具体使用
1. 组件使用
2. 常用配置
① blocks 背景
② prizes 奖品
③ buttons 抽奖按钮
3. 回调函数
① start 开始抽奖前
② end 结束抽奖后
4. 组件方法
① init() 初始化
② play() 开始抽奖
③ stop() 缓慢停止
四、三种抽奖完整代码示例
1. 转盘
2.九宫格
3.老虎机
前言
平时做项目时常会遇到抽奖需求。如果一个一个原生手敲的话,很费时间。所以找到了一款抽奖插件——lucky-canvas,一个基于 JavaScript 的跨平台 ( 大转盘 / 九宫格 / 老虎机 ) 抽奖插件。可以满足绝大部分的项目需求。(高定制并且细节较多的话仍需原生手写)。
本文将介绍该插件的使用方法,并以vue3项目举例。
一、lucky-canvas介绍
该插件基于 JS + Canvas 实现的【大转盘 & 九宫格 & 老虎机】抽奖,致力于为 WEB 前端提供一个功能强大且专业可靠的营销组件,只需要通过简单配置即可实现自由化定制,帮助你快速的完成产品需求。
该插件可以在跨平台使用,比如:
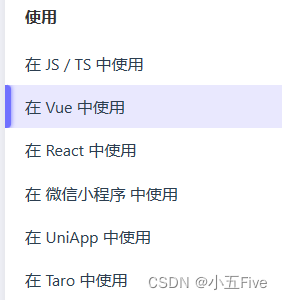
该插件也提供了各种示例,有基础示例和完整示例,比如:
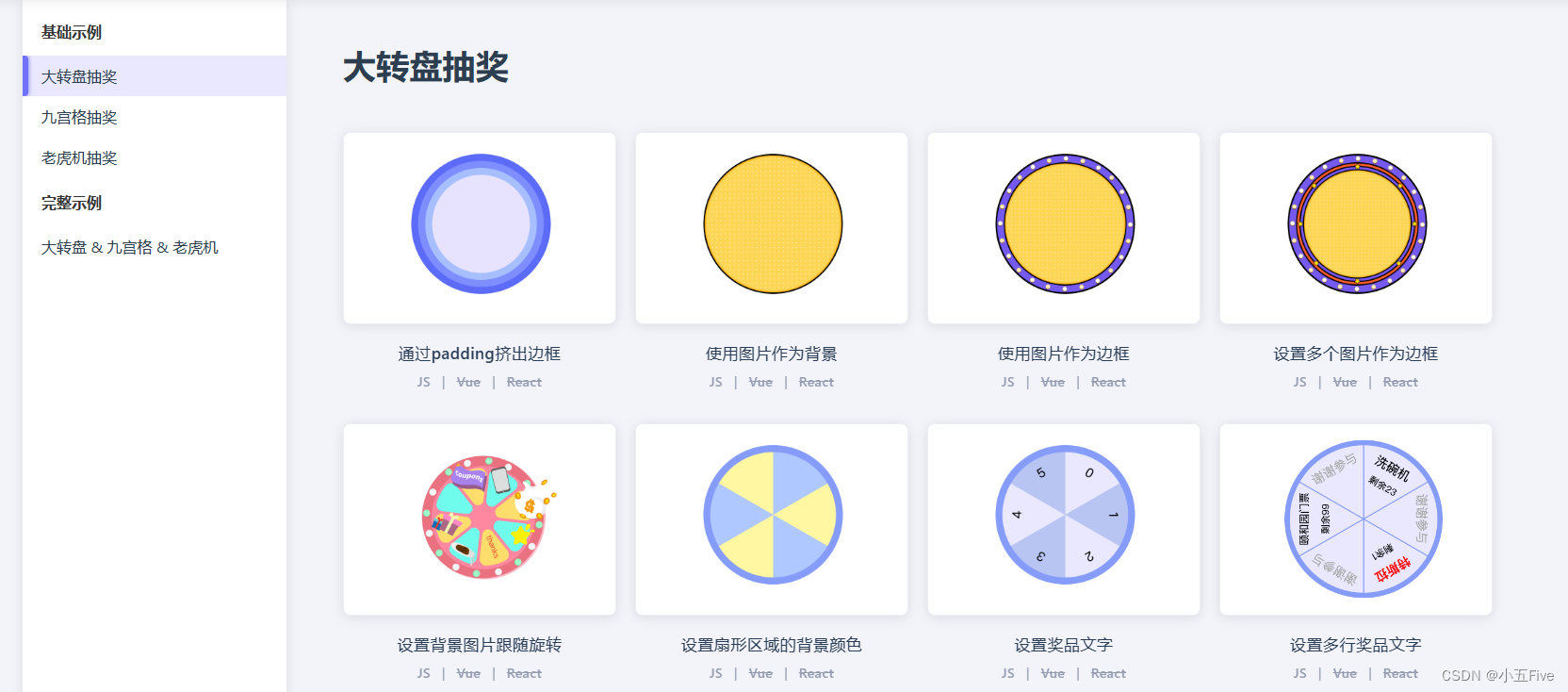
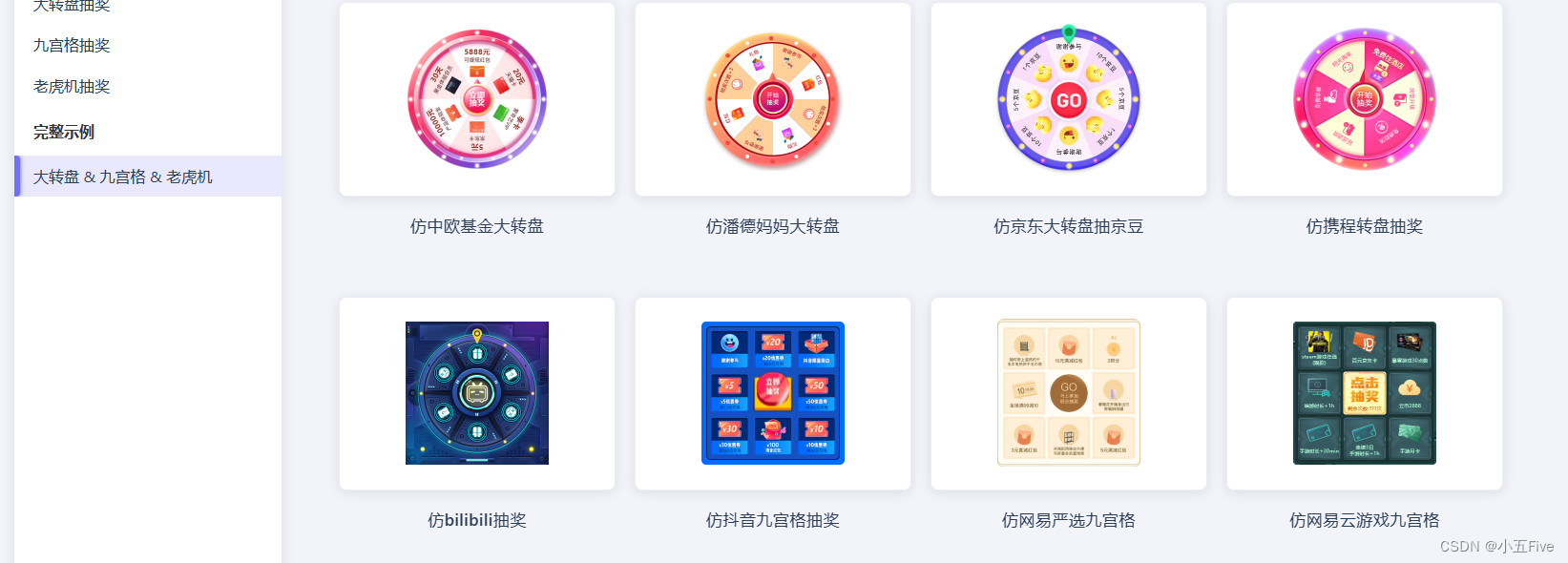
并且提供了api文档:
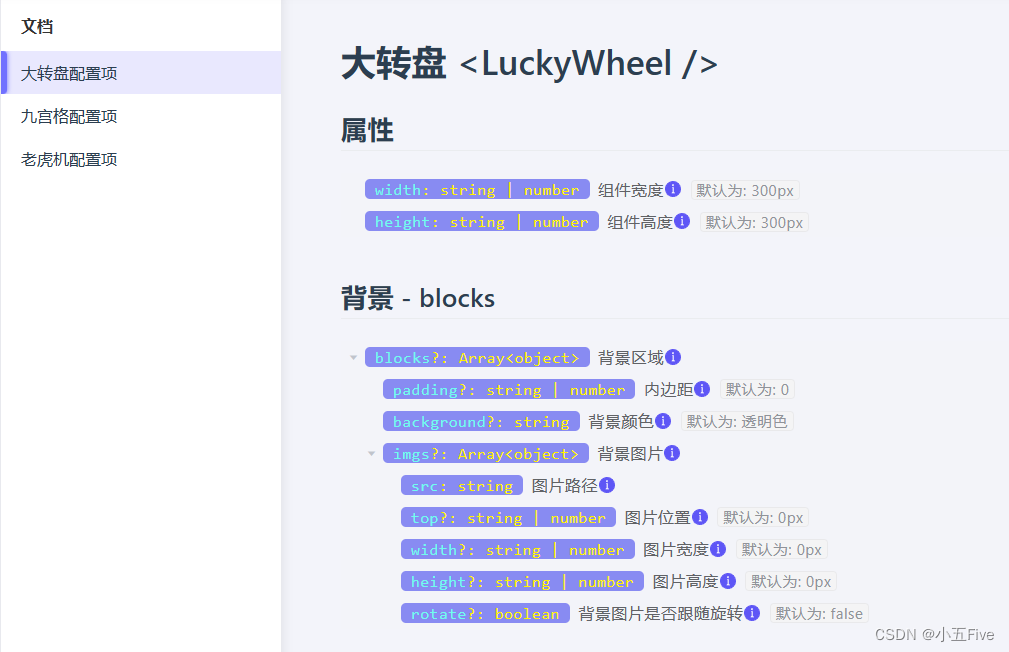
二、lucky-canvas使用(Vue3)
1. 安装
这里是以Vue为例,所以下载的是 @lucky-canvas/vue,如果是其他框架,比如是react,就是下载@lucky-canvas/vue/react,依此类推。
# npm 安装
npm install @lucky-canvas/vue@latest
# 或者 yarn 安装
yarn add @lucky-canvas/vue@latest
# 或者 pnpm 安装
pnpm install @lucky-canvas/vue@latest
2. 注册
main.ts:
// 完整加载
import VueLuckyCanvas from '@lucky-canvas/vue'
const app = createApp(App)
app.use(VueLuckyCanvas)
app.mount('#app')
3. 使用
直接在template里面以组件的形式进行使用。
官网的Vue3示例只提供了转盘,名称如下:
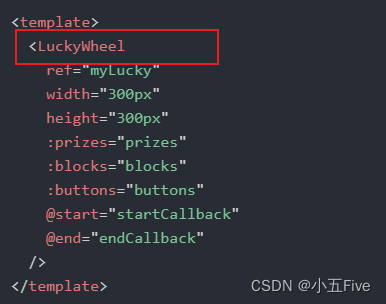
其余的,九宫格对应为LuckyGrid,老虎机对应为SlotMachine。
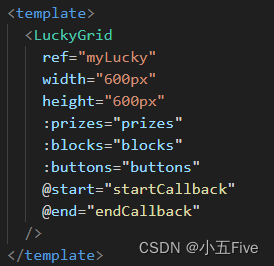
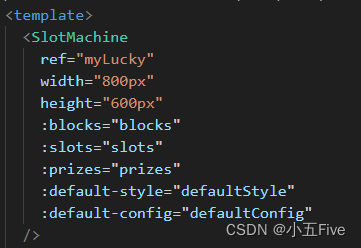
三、九宫格为例的具体使用
1. 组件使用
使用组件,并且给定默认宽高和背景。
<template>
<LuckyGrid
ref="myLucky"
width="600px"
height="600px"
:blocks="blocks"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const blocks = [{ padding: '13px', background: '#617df2' }]
</script>
会出现:
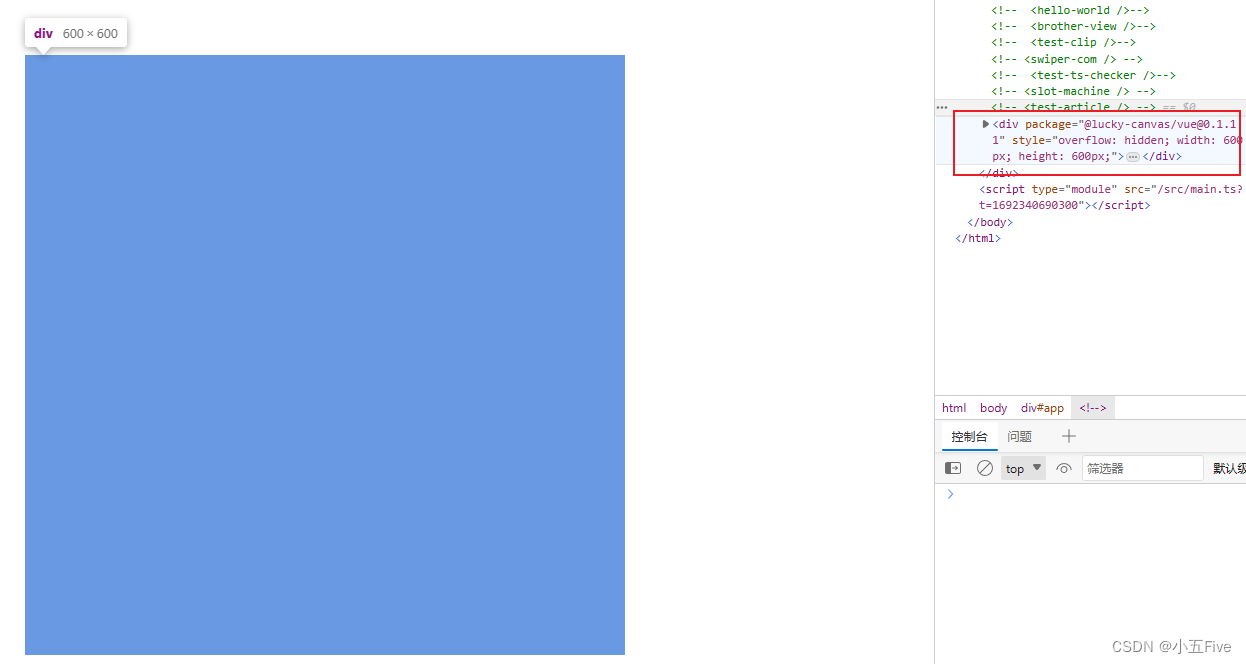
其中的blocks,就是九宫格盒子的背景。也是我们接触到的第一个配置。
2. 常用配置
① blocks 背景
用数组表示。
② prizes 奖品
用数组表示。
x和y是相对坐标,x表示第几行,y表示第几列。
font是文字,并且可以通过top设置距离顶部的距离。还有很多其他字段,可以设置字体颜色大小等等。
background是背景,背景还可以通过imgs字段替换成图片。
<template>
<LuckyGrid
ref="myLucky"
width="600px"
height="600px"
:blocks="blocks"
:prizes="prizes"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const prizes = [
{ x: 0, y: 0, fonts: [{ text: '0', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 0, fonts: [{ text: '1', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 0, fonts: [{ text: '2', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 1, fonts: [{ text: '3', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 2, fonts: [{ text: '4', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 2, fonts: [{ text: '5', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 2, fonts: [{ text: '6', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 1, fonts: [{ text: '7', top: '25%' }], background: '#b3c8fa' }
]
</script>
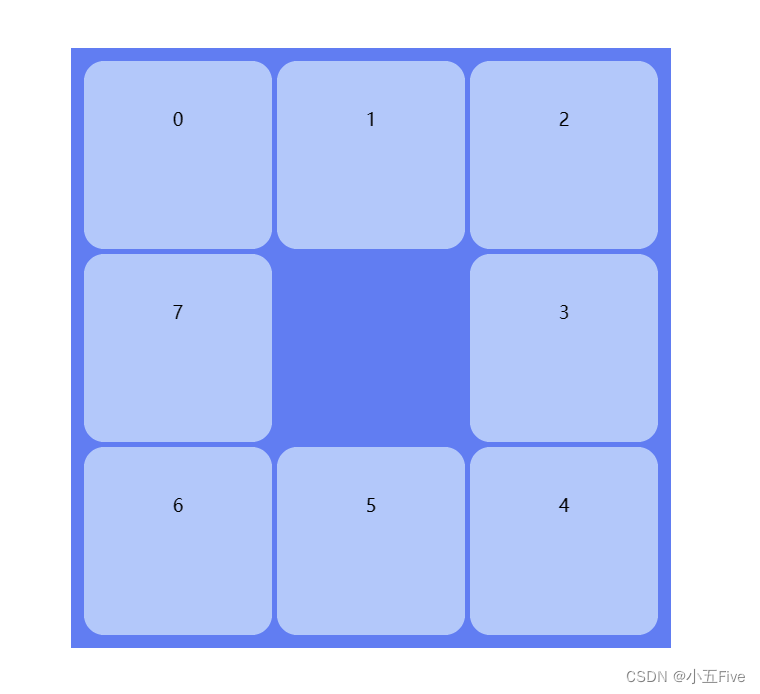
加了抽奖按钮后,中间的空就填好了。
x和y是相对坐标,x表示第几行,y表示第几列。
font是文字,并且可以通过top设置距离顶部的距离。还有很多其他字段,可以设置字体颜色大小等等。
background是背景,背景还可以通过imgs字段替换成图片。
<template>
<LuckyGrid
ref="myLucky"
width="600px"
height="600px"
:blocks="blocks"
:prizes="prizes"
:buttons="buttons"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const prizes = [
{ x: 0, y: 0, fonts: [{ text: '0', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 0, fonts: [{ text: '1', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 0, fonts: [{ text: '2', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 1, fonts: [{ text: '3', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 2, fonts: [{ text: '4', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 2, fonts: [{ text: '5', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 2, fonts: [{ text: '6', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 1, fonts: [{ text: '7', top: '25%' }], background: '#b3c8fa' }
]
const blocks = [{ padding: '13px', background: '#617df2' }]
const buttons = [
{
x: 1,
y: 1,
background: '#7f95d1',
fonts: [{ text: '开始', top: '25%' }]
}
]
</script>
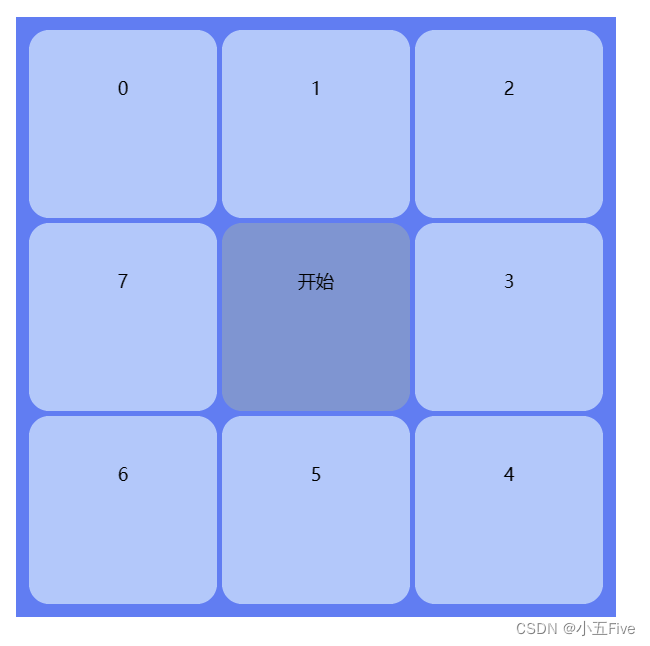
3. 回调函数
<template>
<LuckyGrid
ref="myLucky"
width="600px"
height="600px"
:prizes="prizes"
:blocks="blocks"
:buttons="buttons"
@start="startCallback"
@end="endCallback"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const prizes = [
{ x: 0, y: 0, fonts: [{ text: '0', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 0, fonts: [{ text: '1', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 0, fonts: [{ text: '2', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 1, fonts: [{ text: '3', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 2, fonts: [{ text: '4', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 2, fonts: [{ text: '5', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 2, fonts: [{ text: '6', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 1, fonts: [{ text: '7', top: '25%' }], background: '#b3c8fa' }
]
const blocks = [{ padding: '13px', background: '#617df2' }]
const buttons = [
{
x: 1,
y: 1,
background: '#7f95d1',
fonts: [{ text: '开始', top: '25%' }]
}
]
// 点击抽奖按钮会触发star回调
function startCallback() {
console.log(myLucky?.value)
// 调用抽奖组件的play方法开始游戏
myLucky?.value?.play()
// 模拟调用接口异步抽奖
setTimeout(() => {
// 假设后端返回的中奖索引是0
const index = 0
// 调用stop停止旋转并传递中奖索引
myLucky?.value?.stop(index)
}, 3000)
}
// 抽奖结束会触发end回调
function endCallback(prize: any) {
console.log(prize)
}
</script>
<style scoped lang="scss"></style>
① ① start 开始抽奖前
当点击抽奖按钮时, 触发该回调, 此时你可以决定是否要开始游戏。如果你是多按钮, 则可以通过第二个参数知道是哪个按钮触发的抽奖
② end 结束抽奖后
当游戏完全结束后, 触发该回调, 你可以在此时弹窗恭喜用户。参数则是中奖的奖品。
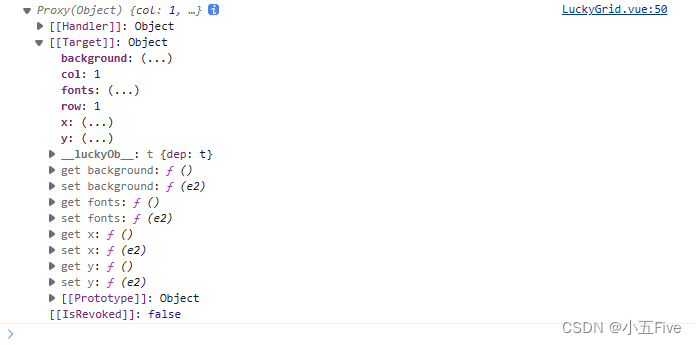
4. 组件方法
① init() 初始化
调用该方法时, 会立刻停止游戏, 回到最初始的状态。
② play() 开始抽奖
调用该方法时, 游戏才会开始。
③ stop() 缓慢停止
调用该方法时, 才会缓慢停止, 参数就是中奖奖品的索引。
四、三种抽奖完整代码示例
1. 转盘
<template>
<LuckyWheel
ref="myLucky"
width="300px"
height="300px"
:prizes="prizes"
:blocks="blocks"
:buttons="buttons"
@start="startCallback"
@end="endCallback"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const prizes = [
{ background: '#e9e8fe', fonts: [{ text: '0' }] },
{ background: '#b8c5f2', fonts: [{ text: '1' }] },
{ background: '#e9e8fe', fonts: [{ text: '2' }] },
{ background: '#b8c5f2', fonts: [{ text: '3' }] },
{ background: '#e9e8fe', fonts: [{ text: '4' }] },
{ background: '#b8c5f2', fonts: [{ text: '5' }] }
]
const blocks = [{ padding: '10px', background: '#869cfa' }]
const buttons = [
{ radius: '40%', background: '#617df2' },
{ radius: '35%', background: '#afc8ff' },
{
radius: '30%',
background: '#869cfa',
pointer: true,
fonts: [{ text: '开始', top: '-10px' }]
}
]
// 点击抽奖按钮会触发star回调
function startCallback() {
console.log(myLucky?.value)
// 调用抽奖组件的play方法开始游戏
myLucky?.value?.play()
// 模拟调用接口异步抽奖
setTimeout(() => {
// 假设后端返回的中奖索引是0
const index = 0
// 调用stop停止旋转并传递中奖索引
myLucky?.value?.stop(index)
}, 3000)
}
// 抽奖结束会触发end回调
function endCallback(prize: any) {
console.log(prize)
}
</script>
<style scoped lang="scss"></style>
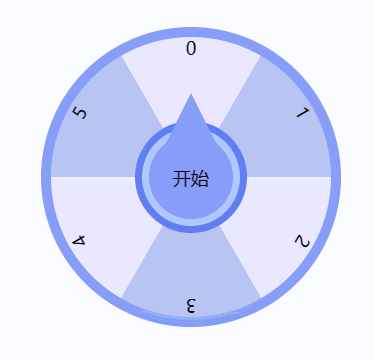
2.九宫格
<template>
<LuckyGrid
ref="myLucky"
width="600px"
height="600px"
:prizes="prizes"
:blocks="blocks"
:buttons="buttons"
@start="startCallback"
@end="endCallback"
/>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const prizes = [
{ x: 0, y: 0, fonts: [{ text: '0', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 0, fonts: [{ text: '1', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 0, fonts: [{ text: '2', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 1, fonts: [{ text: '3', top: '25%' }], background: '#b3c8fa' },
{ x: 2, y: 2, fonts: [{ text: '4', top: '25%' }], background: '#b3c8fa' },
{ x: 1, y: 2, fonts: [{ text: '5', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 2, fonts: [{ text: '6', top: '25%' }], background: '#b3c8fa' },
{ x: 0, y: 1, fonts: [{ text: '7', top: '25%' }], background: '#b3c8fa' }
]
const blocks = [{ padding: '13px', background: '#617df2' }]
const buttons = [
{
x: 1,
y: 1,
background: '#7f95d1',
fonts: [{ text: '开始', top: '25%' }]
}
]
// 点击抽奖按钮会触发star回调
function startCallback() {
console.log(myLucky?.value)
// 调用抽奖组件的play方法开始游戏
myLucky?.value?.play()
// 模拟调用接口异步抽奖
setTimeout(() => {
// 假设后端返回的中奖索引是0
const index = 0
// 调用stop停止旋转并传递中奖索引
myLucky?.value?.stop(index)
}, 3000)
}
// 抽奖结束会触发end回调
function endCallback(prize: any) {
console.log(prize)
}
</script>
<style scoped lang="scss"></style>
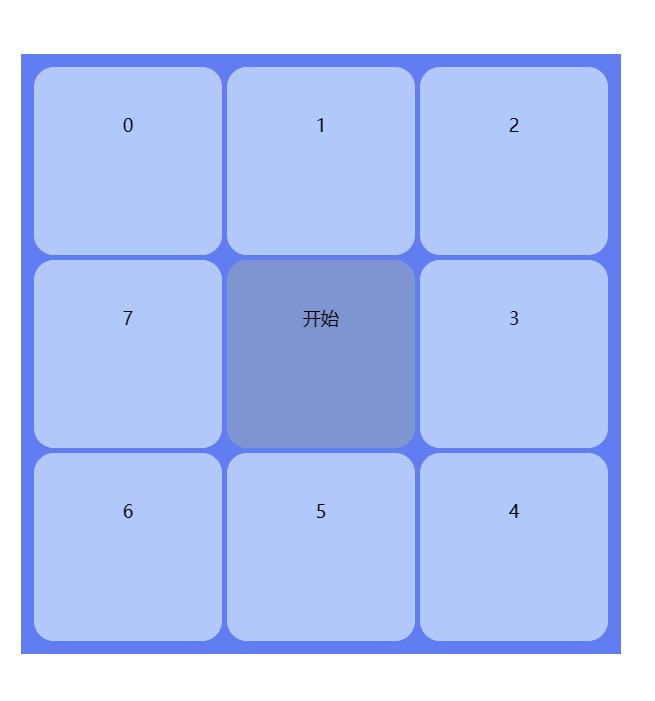
3.老虎机
<template>
<SlotMachine
ref="myLucky"
width="800px"
height="600px"
:blocks="blocks"
:slots="slots"
:prizes="prizes"
:default-style="defaultStyle"
:default-config="defaultConfig"
/>
<button @click="handleStart">开始</button>
</template>
<script setup lang="ts">
const myLucky = ref<any>(null)
const slots = [{ order: [0, 1, 2] }, { order: [2, 0, 1] }, { order: [1, 2, 0] }] // 一开始的排序
const prizes = [
{
background: 'red',
borderRadius: '10px'
},
{
background: 'blue',
borderRadius: '10px'
},
{
background: 'green',
borderRadius: '10px'
}
]
const defaultStyle = {
borderRadius: Infinity,
background: '#bac5ee',
fontSize: '32px',
fontColor: '#333'
}
const defaultConfig = {
rowSpacing: '25px',
colSpacing: '10px'
}
const blocks = [
{ padding: '10px', background: '#869cfa' },
{ padding: '10px', background: '#e9e8fe' }
]
// 点击抽奖按钮会触发star回调
function handleStart() {
myLucky.value.play()
setTimeout(() => {
const prizeIndex = 1 // prizes的索引,蓝色
myLucky.value.stop(prizeIndex)
}, 2000)
}
</script>
<style scoped lang="scss"></style>
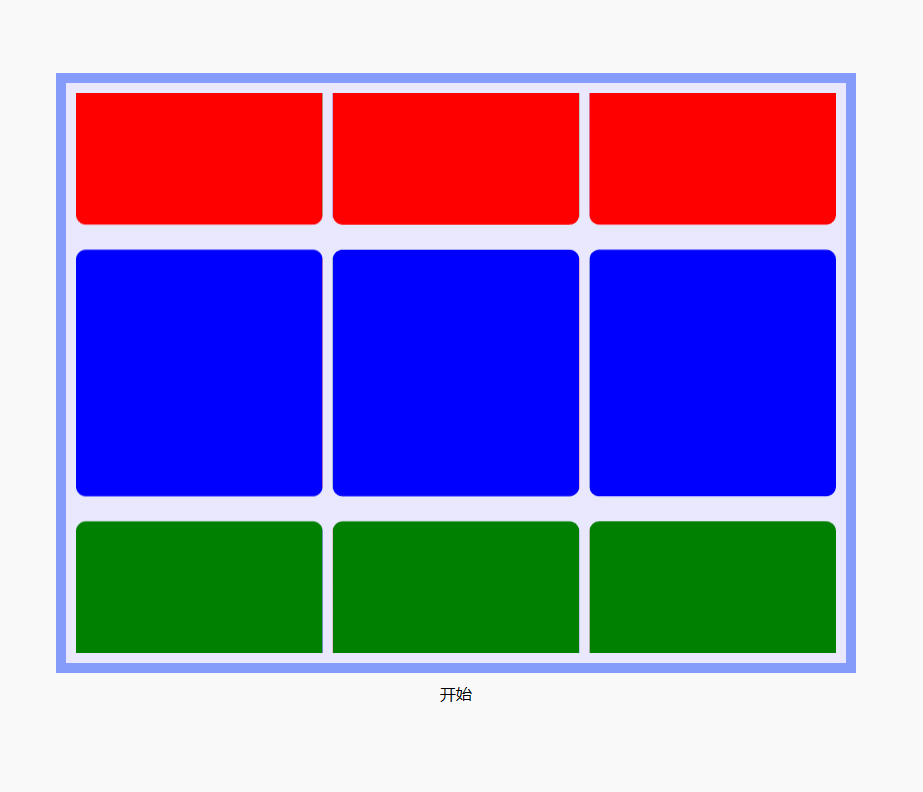
更多可见官网:100px.net | 基于 Js / TS / Vue / React / 微信小程序 / uni-app / Taro 的【大转盘 & 九宫格 & 老虎机】抽奖插件
https://100px.net/