项目描述 : 以SpringBoot为项目框架开发的二手交易网站,主要用作个人学习,网站的功能模块有:
买家模块、卖家模块、购物车 模块、订单模块、内容管理模块。通过这一系列模块能基本满足二手商品的线上交易,基本功能也全部实现
技术架构 : SpringBoot+MyBatis-plus+Thymeleaf+Redis+swagger
技术描述 :
package com.jzh.xx.transaction.controller;
import com.alipay.api.AlipayApiException;
import com.alipay.api.AlipayClient;
import com.alipay.api.DefaultAlipayClient;
import com.alipay.api.internal.util.AlipaySignature;
import com.alipay.api.request.AlipayTradePagePayRequest;
import com.jzh.xx.transaction.config.AlipayConfig;
import com.jzh.xx.transaction.domain.*;
import com.jzh.xx.transaction.service.*;
import com.jzh.xx.transaction.vo.OrderVO;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.util.*;
@Controller
@RequestMapping("checkout")
public class CheckoutController {
@Resource
private CategoryService categoryService;
@Resource
private CategoryTwoService categoryTwoService;
@Resource
private CartService cartService;
@Resource
private ExpressService expressService;
@Resource
private OrderService orderService;
@Resource
private UserService userService;
/**
* 订单信息页
* @param id
* @param model
* @param session
* @return
*/
@GetMapping("{id}")
public String toCheckout(@PathVariable Long id, Model model, HttpSession session){
// 获取用户 ID
XxUser user = (XxUser) session.getAttribute("user");
// 查询订单详情
OrderVO orderVO = orderService.getById(id);
// 购物车
List<Cart> cartGoods = new ArrayList<>();
if (user != null){
cartGoods = cartService.getByUserId(user.getId());
}
Double total = 0.0;
int goodsCount = cartGoods.size();
if (cartGoods.size() >0){
for (int i = 0; i< cartGoods.size(); i++){
total += cartGoods.get(i).getBuyCount() * cartGoods.get(i).getGoodsPrice();
}
}
// 父分类
List<Category> categories = categoryService.categoryList();
// 查询所有子分类
List<CategoryTwo> categoryTwos = categoryTwoService.getAll();
//查询所有快递
List<Express> expresses = expressService.getAll();
model.addAttribute("user",user);
model.addAttribute("orderVO",orderVO);
model.addAttribute("goodsCount",goodsCount);
model.addAttribute("cartGoods",cartGoods);
model.addAttribute("total",total);
model.addAttribute("categories",categories);
model.addAttribute("categoryTwos",categoryTwos);
model.addAttribute("expresses",expresses);
return "checkout";
}
@GetMapping("completed")
public String toCompleted(Model model, HttpSession session){
// 获取用户 ID
XxUser user = (XxUser) session.getAttribute("user");
// 购物车
List<Cart> cartGoods = new ArrayList<>();
if (user != null){
cartGoods = cartService.getByUserId(user.getId());
}
Double total = 0.0;
int goodsCount = cartGoods.size();
if (cartGoods.size() >0){
for (int i = 0; i< cartGoods.size(); i++){
total += cartGoods.get(i).getBuyCount() * cartGoods.get(i).getGoodsPrice();
}
}
// 父分类
List<Category> categories = categoryService.categoryList();
// 查询所有子分类
List<CategoryTwo> categoryTwos = categoryTwoService.getAll();
model.addAttribute("goodsCount",goodsCount);
model.addAttribute("cartGoods",cartGoods);
model.addAttribute("total",total);
model.addAttribute("categories",categories);
model.addAttribute("categoryTwos",categoryTwos);
return "completed";
}
@GetMapping("failPay")
public String toFailPay(Model model, HttpSession session){
// 获取用户 ID
XxUser user = (XxUser) session.getAttribute("user");
// 购物车
List<Cart> cartGoods = new ArrayList<>();
if (user != null){
cartGoods = cartService.getByUserId(user.getId());
}
Double total = 0.0;
int goodsCount = cartGoods.size();
if (cartGoods.size() >0){
for (int i = 0; i< cartGoods.size(); i++){
total += cartGoods.get(i).getBuyCount() * cartGoods.get(i).getGoodsPrice();
}
}
// 父分类
List<Category> categories = categoryService.categoryList();
// 查询所有子分类
List<CategoryTwo> categoryTwos = categoryTwoService.getAll();
model.addAttribute("goodsCount",goodsCount);
model.addAttribute("cartGoods",cartGoods);
model.addAttribute("total",total);
model.addAttribute("categories",categories);
model.addAttribute("categoryTwos",categoryTwos);
return "failtopay";
}
@PostMapping("/AliPay")
public void goPay(Long orderId, HttpServletResponse response) throws IOException {
//查询出订单信息
OrderVO order = orderService.getById(orderId);
//获得初始化的AlipayClient
AlipayClient alipayClient = new DefaultAlipayClient(AlipayConfig.gatewayUrl, AlipayConfig.app_id, AlipayConfig.merchant_private_key, "json", AlipayConfig.charset, AlipayConfig.alipay_public_key, AlipayConfig.sign_type);
AlipayTradePagePayRequest alipayRequest = new AlipayTradePagePayRequest();
alipayRequest.setReturnUrl(AlipayConfig.return_url);
//alipayRequest.setNotifyUrl(AlipayConfig.notify_url);
//商户订单号,商户网站订单系统中唯一订单号,必填
String out_trade_no = Long.toString(orderId);
//付款金额,必填
String total_amount = String.valueOf(order.getTotal());
//订单名称,必填
String subject = "闲闲网二手物品交易";
//商品描述,可空
String body = "";
// 该笔订单允许的最晚付款时间,逾期将关闭交易。取值范围:1m~15d。m-分钟,h-小时,d-天,1c-当天(1c-当天的情况下,无论交易何时创建,都在0点关闭)。 该参数数值不接受小数点, 如 1.5h,可转换为 90m。
String timeout_express = "5m";
alipayRequest.setBizContent("{\"out_trade_no\":\""+ out_trade_no +"\","
+ "\"total_amount\":\""+ total_amount +"\","
+ "\"subject\":\""+ subject +"\","
+ "\"body\":\""+ body +"\","
+ "\"timeout_express\":\""+ timeout_express +"\","
+ "\"product_code\":\"FAST_INSTANT_TRADE_PAY\"}");
//请求
String form="";
try {
form = alipayClient.pageExecute(alipayRequest).getBody(); //调用SDK生成表单
} catch (AlipayApiException e) {
e.printStackTrace();
}
response.setContentType("text/html;charset=" + AlipayConfig.charset);
response.getWriter().write(form);//直接将完整的表单html输出到页面
response.getWriter().flush();
response.getWriter().close();
}
/**
* 回调页
* @param request
* @param response
* @return
* @throws IOException
* @throws AlipayApiException
*/
@GetMapping("returnUrl")
public String returnUrl(HttpServletRequest request, HttpServletResponse response, HttpSession session)
throws IOException, AlipayApiException {
// 获取支付宝GET过来反馈信息
Map<String, String> params = new HashMap<String, String>();
Map<String, String[]> requestParams = request.getParameterMap();
for (Iterator<String> iter = requestParams.keySet().iterator(); iter.hasNext();) {
String name = (String) iter.next();
String[] values = (String[]) requestParams.get(name);
String valueStr = "";
for (int i = 0; i < values.length; i++) {
valueStr = (i == values.length - 1) ? valueStr + values[i] : valueStr + values[i] + ",";
}
// 乱码解决,这段代码在出现乱码时使用
valueStr = new String(valueStr.getBytes("utf-8"), "utf-8");
params.put(name, valueStr);
}
boolean signVerified = AlipaySignature.rsaCheckV1(params, AlipayConfig.alipay_public_key, AlipayConfig.charset, AlipayConfig.sign_type); // 调用SDK验证签名
//验证签名通过
if(signVerified){
// 商户订单号
String out_trade_no = new String(request.getParameter("out_trade_no").getBytes("ISO-8859-1"), "UTF-8");
// 支付宝交易号
String trade_no = new String(request.getParameter("trade_no").getBytes("ISO-8859-1"), "UTF-8");
// 付款金额
String total_amount = new String(request.getParameter("total_amount").getBytes("ISO-8859-1"), "UTF-8");
//支付成功,更新订单支付状态
Long orderId = Long.valueOf(out_trade_no);
ShopOrder order =new ShopOrder();
order.setId(orderId);
order.setStatus((byte) 1);
order.setOverTime(new Date());
orderService.updateOrder(order);
//将用户信息放入session
Long userId = orderService.getUserId(orderId);
//清除该用户的购物车
cartService.deleteByUserId(userId);
XxUser user = userService.getById(userId);
session.setAttribute("user",user);
return "redirect:/checkout/completed";//跳转付款成功页面
}else{
return "redirect:/checkout/failPay";//跳转付款失败页面
}
}
}
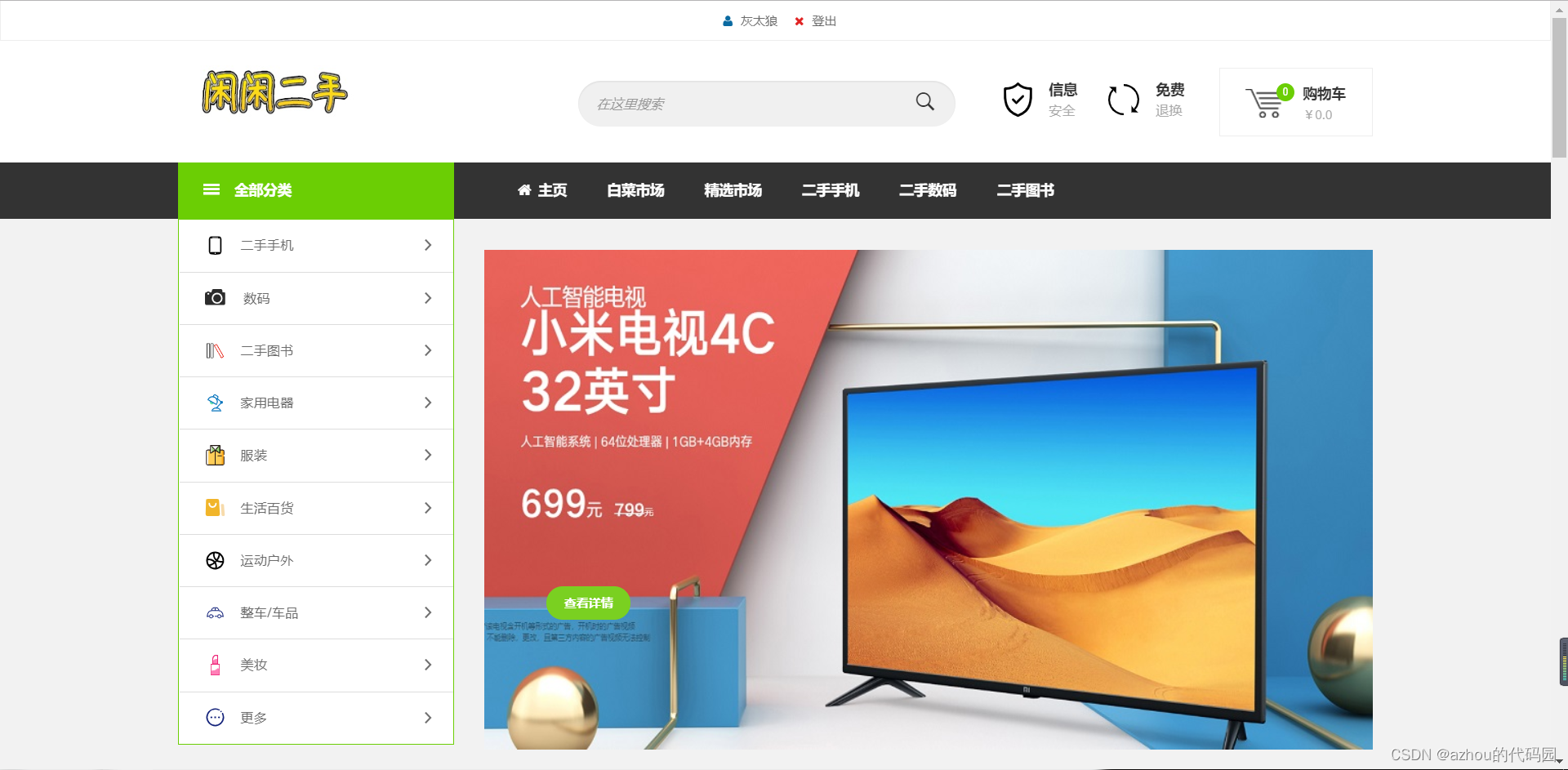
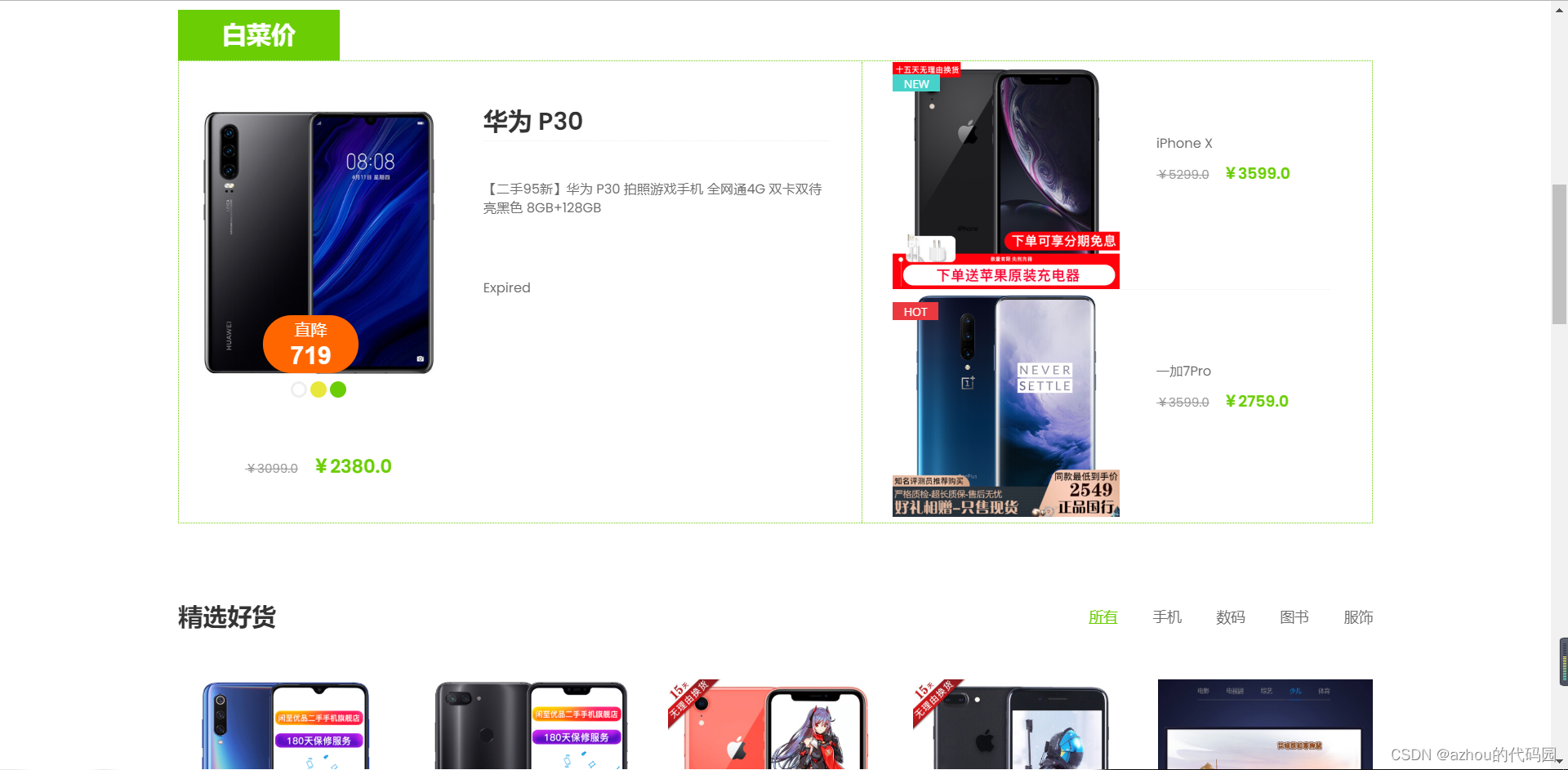
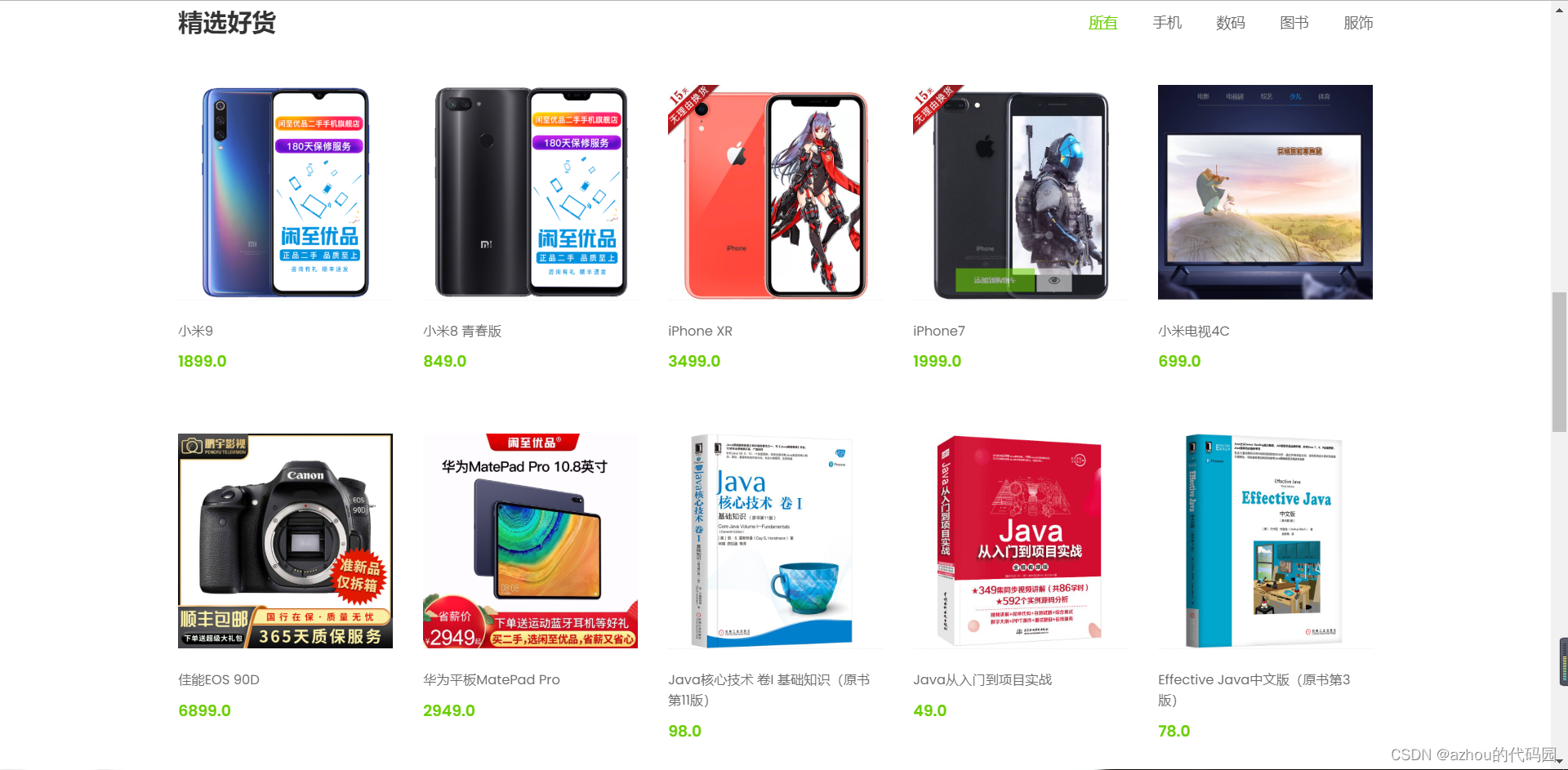
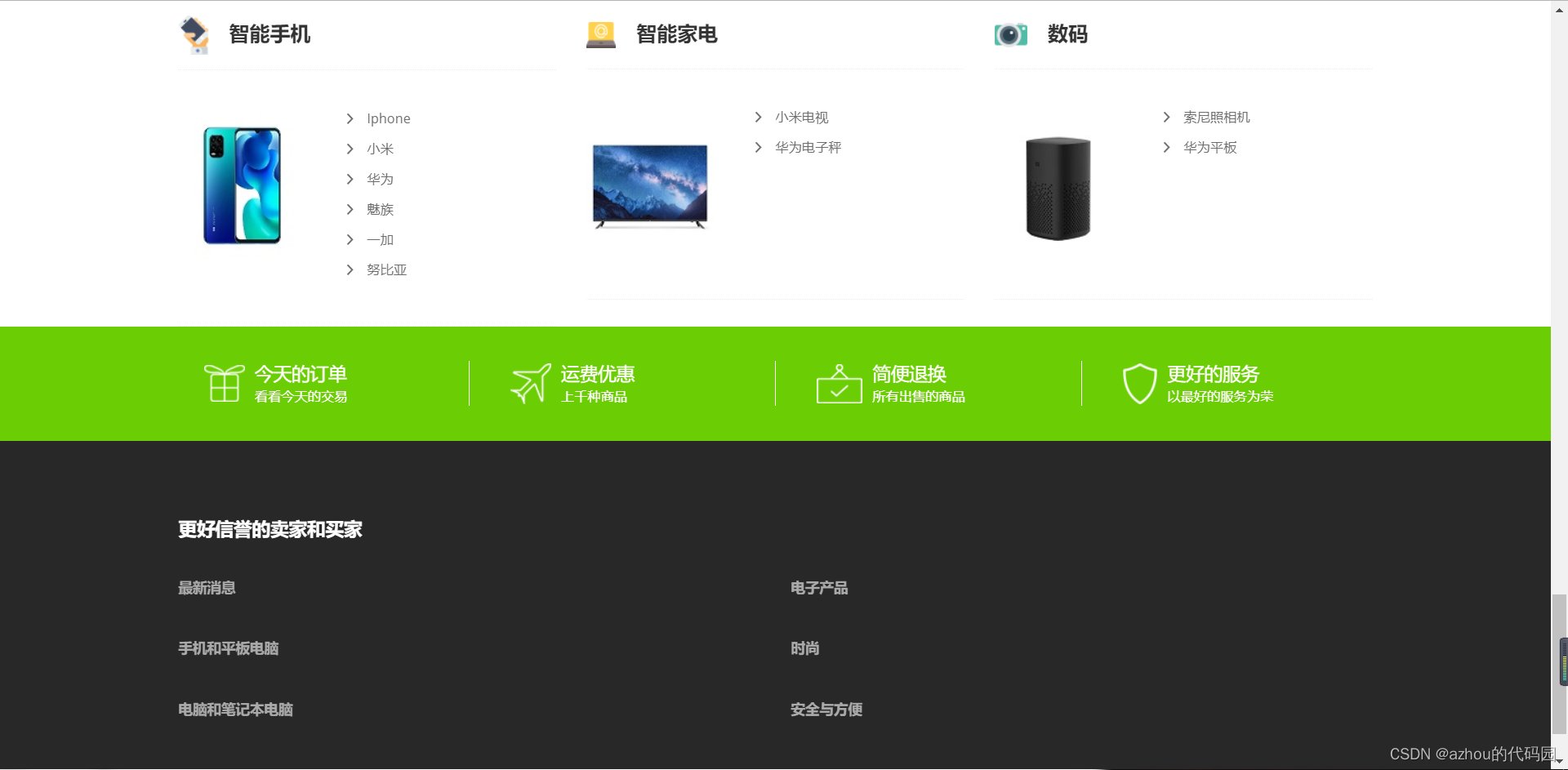
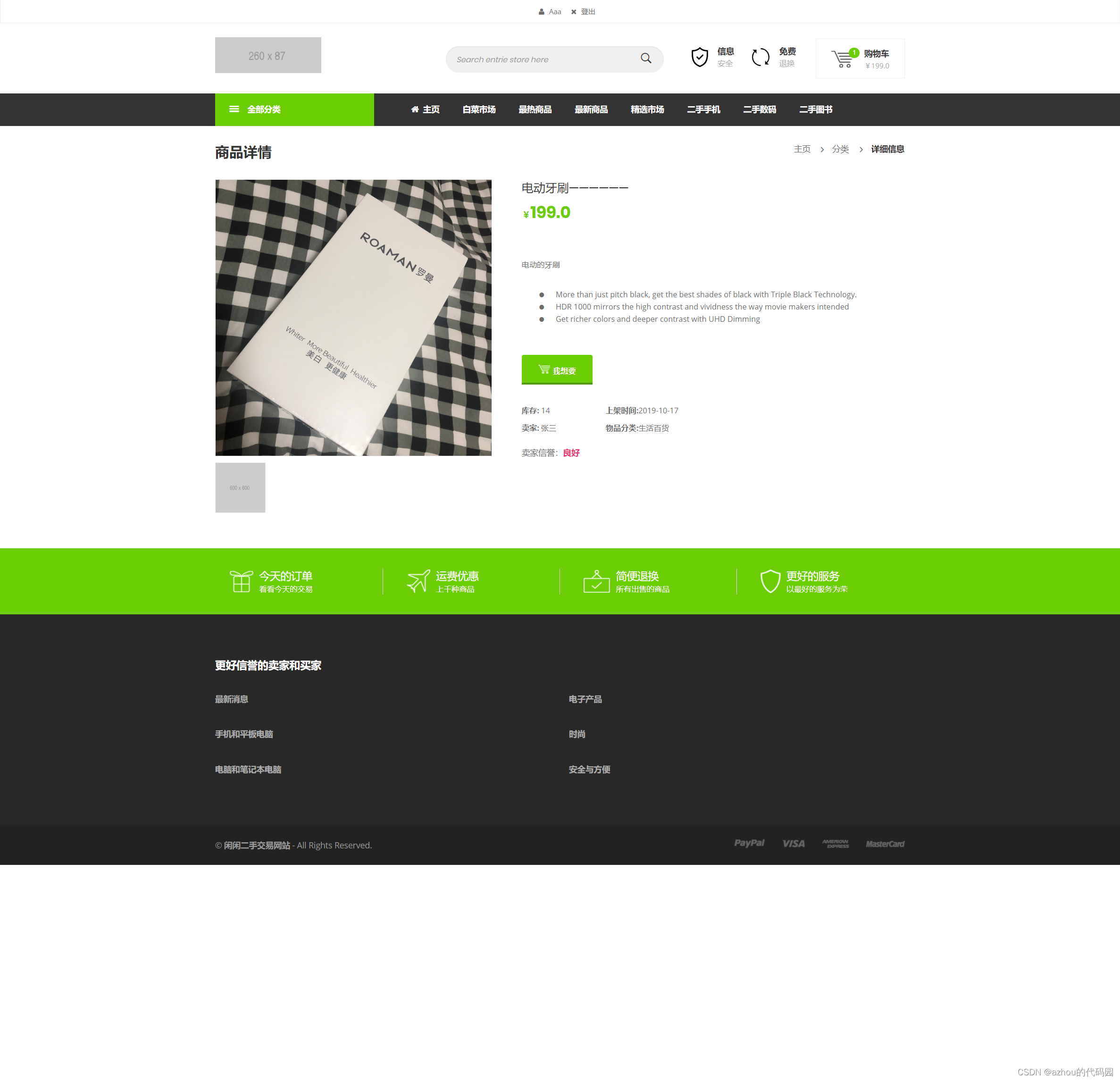
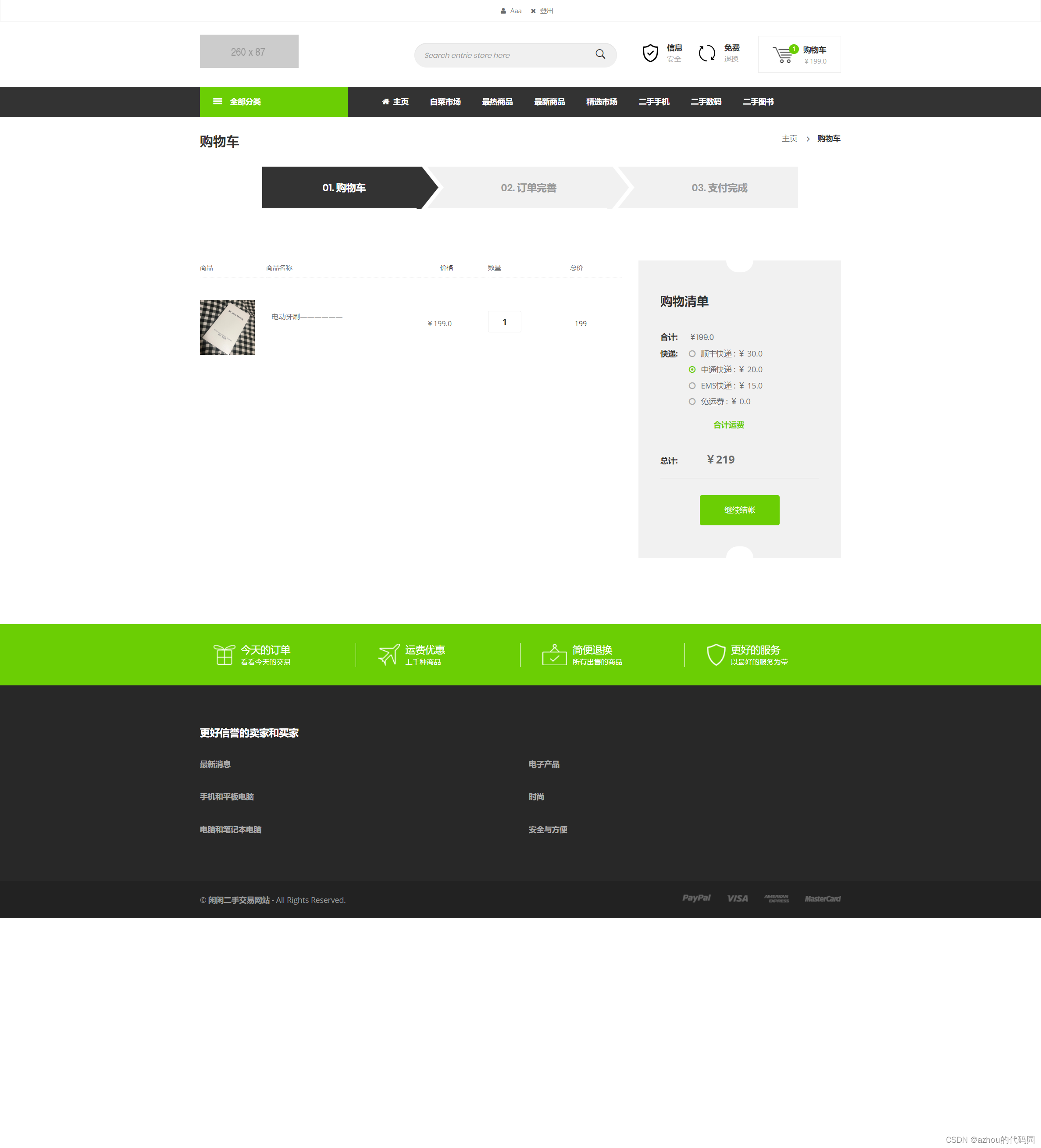
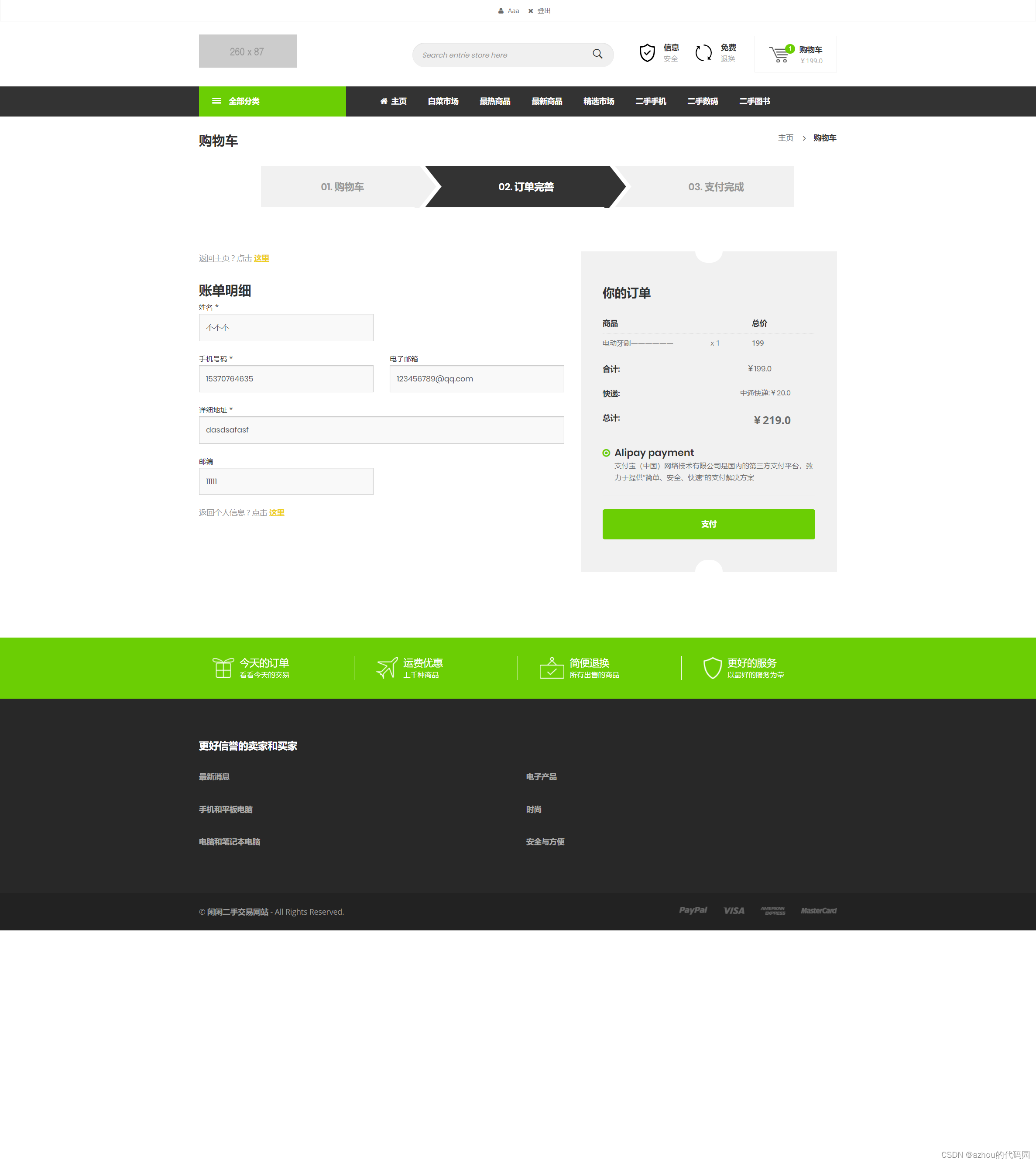
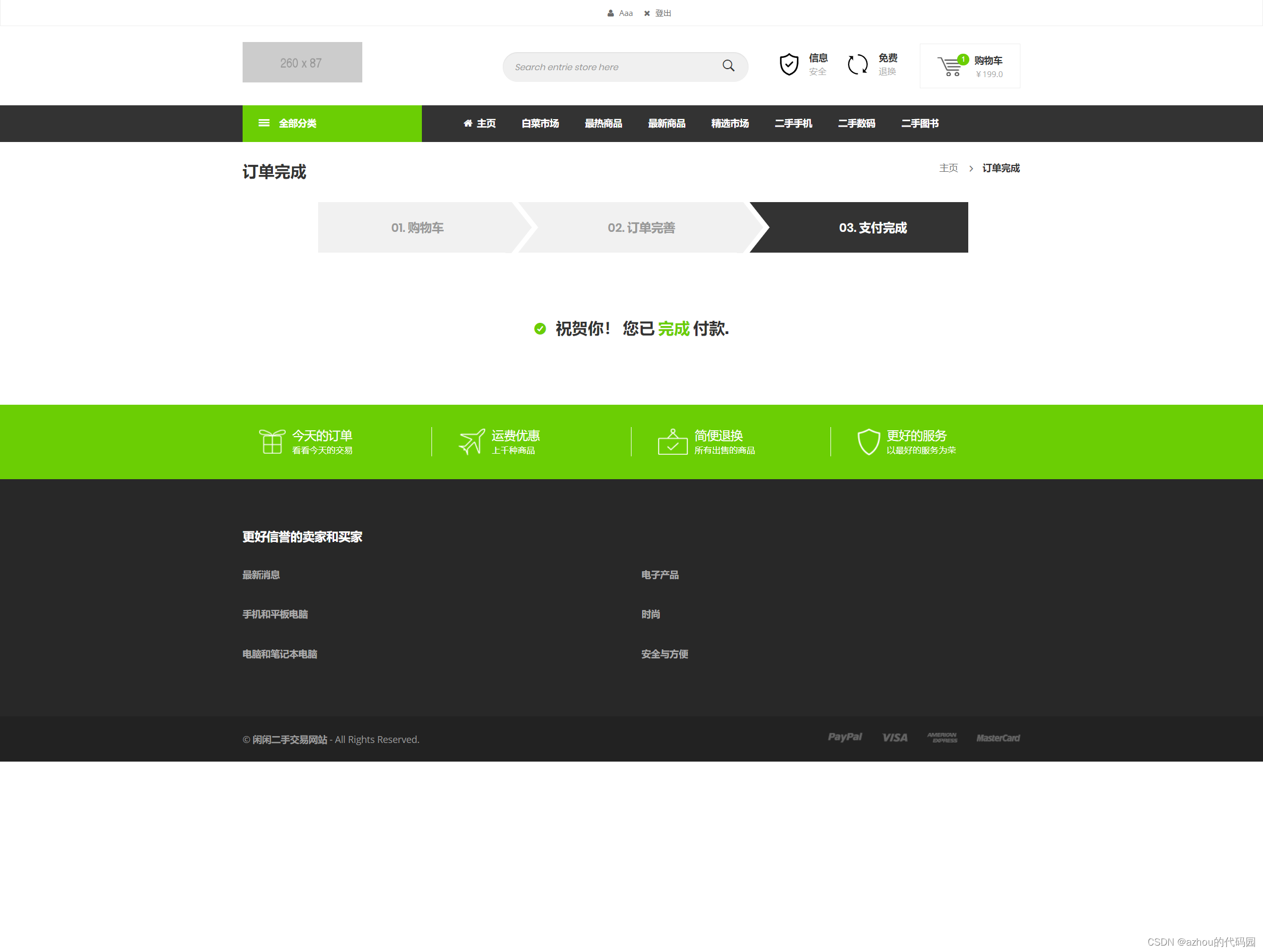
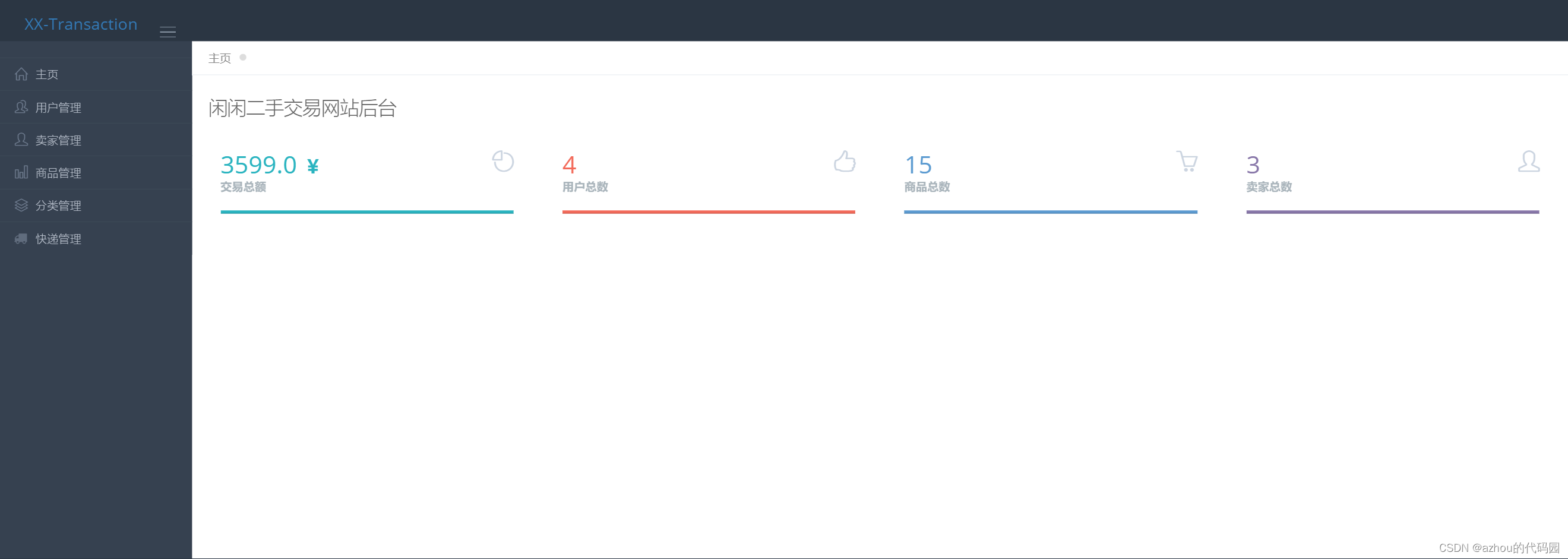
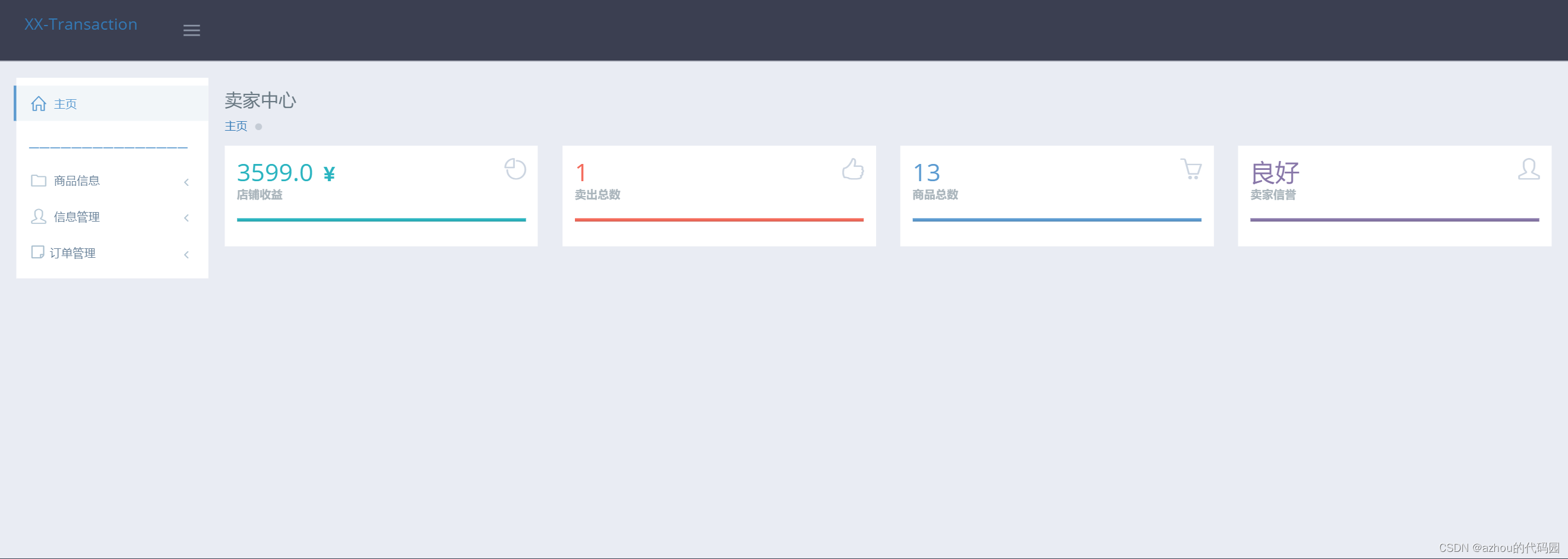
二手交易平台源码链接
https://gitee.com/wuyanzua/blog-applet