参考链接: class torch.device
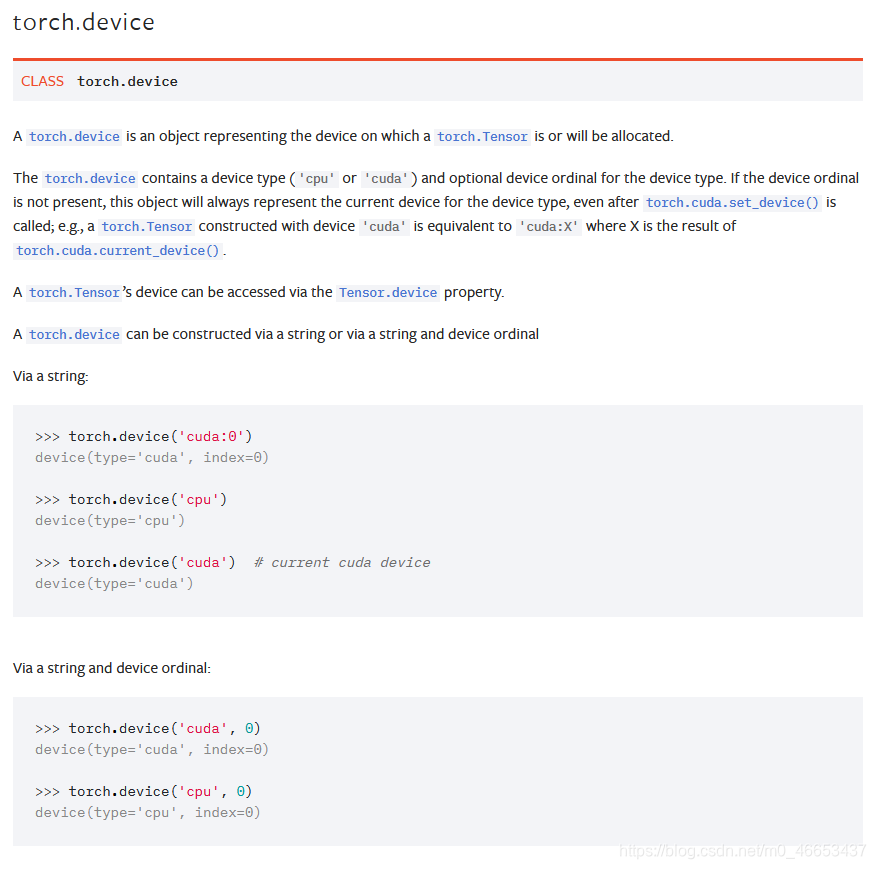
原文及翻译:
torch.device
torch.device栏目
class torch.device
torch.device 类型
A torch.device is an object representing the device on which
a torch.Tensor is or will be allocated.
torch.device的一个实例是一个对象,该对象代表的是张量torch.Tensor所在
的设备或将要分配到的设备.
The torch.device contains a device type ('cpu' or 'cuda') and optional
device ordinal for the device type. If the device ordinal is not
present, this object will always represent the current device for the
device type, even after torch.cuda.set_device() is called; e.g., a
torch.Tensor constructed with device 'cuda' is equivalent to 'cuda:X'
where X is the result of torch.cuda.current_device().
torch.device包含了一个设备类型('cpu' 或者 'cuda'),以及该设备类型的可选设备序数.如果该设备序数不存在,那么这个对象所代表的将总是该设备类型的当前设备,尽管
已经调用了torch.cuda.set_device()函数;例如,使用设备'cuda'来创建一个
torch.Tensor 对象等效于使用设备'cuda:X'来创建torch.Tensor对象,其中X是函数
torch.cuda.current_device()的返回结果.
A torch.Tensor’s device can be accessed via the Tensor.device property.
通过使用张量torch.Tensor的属性Tensor.device 就可以访问该torch.Tensor张量
所在的设备.
A torch.device can be constructed via a string or via a string and device ordinal
通过一个字符串或者一个字符串和设备序数就可以创建一个torch.device对象.
Via a string:
通过一个字符串来创建:
>>> torch.device('cuda:0')
device(type='cuda', index=0)
>>> torch.device('cpu')
device(type='cpu')
>>> torch.device('cuda') # current cuda device # 当前cuda设备
device(type='cuda')
Via a string and device ordinal:
通过一个字符串和设备序数来创建一个设备对象:
>>> torch.device('cuda', 0)
device(type='cuda', index=0)
>>> torch.device('cpu', 0)
device(type='cpu', index=0)
代码实验:
Microsoft Windows [版本 10.0.18363.1316]
(c) 2019 Microsoft Corporation。保留所有权利。
C:\Users\chenxuqi>conda activate ssd4pytorch1_2_0
(ssd4pytorch1_2_0) C:\Users\chenxuqi>python
Python 3.7.7 (default, May 6 2020, 11:45:54) [MSC v.1916 64 bit (AMD64)] :: Anaconda, Inc. on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import torch
>>> # 通过一个字符串来创建:
>>> torch.device('cuda:0')
device(type='cuda', index=0)
>>>
>>> d = torch.device('cuda:0')
>>> d
device(type='cuda', index=0)
>>>
>>> torch.device('cpu')
device(type='cpu')
>>> d = torch.device('cpu')
>>> d
device(type='cpu')
>>>
>>> torch.device('cuda') # current cuda device
device(type='cuda')
>>> d = torch.device('cuda') # current cuda device
>>> d
device(type='cuda')
>>>
>>> # 通过一个字符串和设备序数来创建一个设备对象:
>>> torch.device('cuda', 0)
device(type='cuda', index=0)
>>> d = torch.device('cuda', 0)
>>> d
device(type='cuda', index=0)
>>>
>>> torch.device('cpu', 0)
device(type='cpu', index=0)
>>> d = torch.device('cpu', 0)
>>>
>>>
>>>
>>> torch.device('cpu', 1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CPU device index must be -1 or zero, got 1
>>> torch.device('cpu', -1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Device index must not be negative
>>> torch.device('cuda', 0)
device(type='cuda', index=0)
>>> torch.device('cuda', 1)
device(type='cuda', index=1)
>>> torch.device('cuda', 3)
device(type='cuda', index=3)
>>>
>>>
>>>
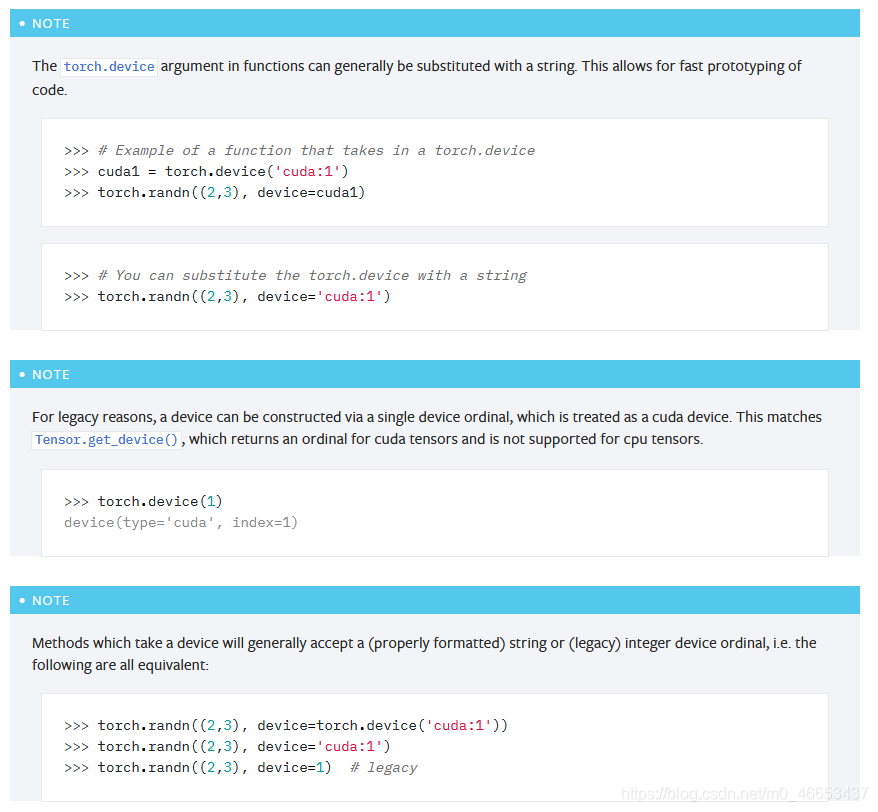
原文及翻译:
Note 注意:
The torch.device argument in functions can generally be substituted
with a string. This allows for fast prototyping of code.
函数的torch.device参数通常可以用一个字符串来替代.这有利于更快的代码原型.
>>> # Example of a function that takes in a torch.device
>>> # 函数接收torch.device作为参数的例子.
>>> cuda1 = torch.device('cuda:1')
>>> torch.randn((2,3), device=cuda1)
>>> # You can substitute the torch.device with a string
>>> # 你可以用一个字符串来替代 torch.device
>>> torch.randn((2,3), device='cuda:1')
Note 注意:
For legacy reasons, a device can be constructed via a single device
ordinal, which is treated as a cuda device. This matches
Tensor.get_device(), which returns an ordinal for cuda tensors and is
not supported for cpu tensors.
由于遗留的历史原因,我们可以通过单个设备序数来创建一个设备,这个序数被用来作为
一个cuda设备的序数.这个序数和Tensor.get_device()匹配,Tensor.get_device()
函数返回cuda张量的序数,并且这个序数不支持cpu张量.
>>> torch.device(1)
device(type='cuda', index=1)
Note 注意:
Methods which take a device will generally accept a (properly
formatted) string or (legacy) integer device ordinal, i.e. the
following are all equivalent:
接收一个device对象的方法通常也可以接收一个(正确格式化的)字符串或者
一个表示设备序数的(遗留的方式)整数,即以下的方式是等效的:
>>> torch.randn((2,3), device=torch.device('cuda:1'))
>>> torch.randn((2,3), device='cuda:1')
>>> torch.randn((2,3), device=1) # legacy # 遗留的使用方式
代码实验展示:
Microsoft Windows [版本 10.0.18363.1316]
(c) 2019 Microsoft Corporation。保留所有权利。
C:\Users\chenxuqi>conda activate ssd4pytorch1_2_0
(ssd4pytorch1_2_0) C:\Users\chenxuqi>python
Python 3.7.7 (default, May 6 2020, 11:45:54) [MSC v.1916 64 bit (AMD64)] :: Anaconda, Inc. on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import torch
>>> torch.manual_seed(seed=20200910)
<torch._C.Generator object at 0x0000024D1851D330>
>>>
>>> torch.device('cuda:1')
device(type='cuda', index=1)
>>> cuda0 = torch.device('cuda:0')
>>> cuda0
device(type='cuda', index=0)
>>> cuda1 = torch.device('cuda:1')
>>> cuda1
device(type='cuda', index=1)
>>> torch.randn((2,3), device=cuda0)
tensor([[-0.9908, 0.5920, -0.0895],
[ 0.7582, 0.0068, -3.2594]], device='cuda:0')
>>> torch.randn((2,3), device=cuda1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CUDA error: invalid device ordinal
>>> # 报错的原因是当前机器上只有一个GPU
>>> torch.randn((2,3), device='cuda:1')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CUDA error: invalid device ordinal
>>> torch.randn((2,3), device='cuda:0')
tensor([[ 1.2275, -0.4505, 0.2995],
[ 0.6775, 0.2833, 1.3016]], device='cuda:0')
>>>
>>> torch.device(1)
device(type='cuda', index=1)
>>> torch.device(0)
device(type='cuda', index=0)
>>>
>>> data = torch.randn((2,3), device='cuda:0')
>>> data
tensor([[ 0.8317, -0.8718, -1.9394],
[-0.7548, 0.9575, 0.0592]], device='cuda:0')
>>> data.get_device()
0
>>> data = torch.randn((2,3))
>>> data
tensor([[ 0.2824, -0.3715, 0.9088],
[-1.7601, -0.1806, 2.0937]])
>>> data.get_device()
-1
>>> data = torch.randn((2,3), device='cpu')
>>> data
tensor([[ 1.0406, -1.7651, 1.1216],
[ 0.8440, 0.1783, 0.6859]])
>>> data.get_device()
-1
>>> torch.device(1)
device(type='cuda', index=1)
>>> torch.device(0)
device(type='cuda', index=0)
>>> torch.device(-1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Device index must not be negative
>>> torch.randn((2,3), device=torch.device('cuda:1'))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CUDA error: invalid device ordinal
>>> torch.randn((2,3), device=torch.device('cuda:0'))
tensor([[ 0.9059, 1.5271, -0.0798],
[ 0.0769, -0.3586, -1.9597]], device='cuda:0')
>>> torch.randn((2,3), device='cuda:1')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CUDA error: invalid device ordinal
>>> torch.randn((2,3), device='cuda:0')
tensor([[ 0.3807, 0.7194, 1.1678],
[-1.1230, 0.6926, 0.0385]], device='cuda:0')
>>> torch.randn((2,3), device=1) # legacy
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: CUDA error: invalid device ordinal
>>> torch.randn((2,3), device=0) # legacy
tensor([[ 0.6609, -1.1344, 0.2916],
[-0.6726, -0.4537, -0.9022]], device='cuda:0')
>>> torch.randn((2,3), device=-1) # legacy
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Device index must not be negative
>>>
>>>
>>>
代码实验:
Microsoft Windows [版本 10.0.18363.1316]
(c) 2019 Microsoft Corporation。保留所有权利。
C:\Users\chenxuqi>conda activate ssd4pytorch1_2_0
(ssd4pytorch1_2_0) C:\Users\chenxuqi>python
Python 3.7.7 (default, May 6 2020, 11:45:54) [MSC v.1916 64 bit (AMD64)] :: Anaconda, Inc. on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import torch
>>> torch.cuda.is_available()
True
>>> print(torch.cuda.is_available())
True
>>> print(torch.__version__)
1.2.0+cu92
>>> torch.manual_seed(seed=20200910)
<torch._C.Generator object at 0x000001CA8034D330>
>>>
>>> device = (torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu'))
>>> device
device(type='cuda')
>>> device_1 = torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu')
>>> device_1
device(type='cuda')
>>> torch.device('cpu')
device(type='cpu')
>>> torch.device('cuda')
device(type='cuda')
>>> torch.device('cuda0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Expected one of cpu, cuda, mkldnn, opengl, opencl, ideep, hip, msnpu device type at start of device string: cuda0
>>> torch.device('cuda:0')
device(type='cuda', index=0)
>>> torch.device('cuda:1')
device(type='cuda', index=1)
>>> torch.device('cuda:-1')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Device index must be non-negative, got -1
>>> torch.device('cuda:3')
device(type='cuda', index=3)
>>>
>>>
>>>
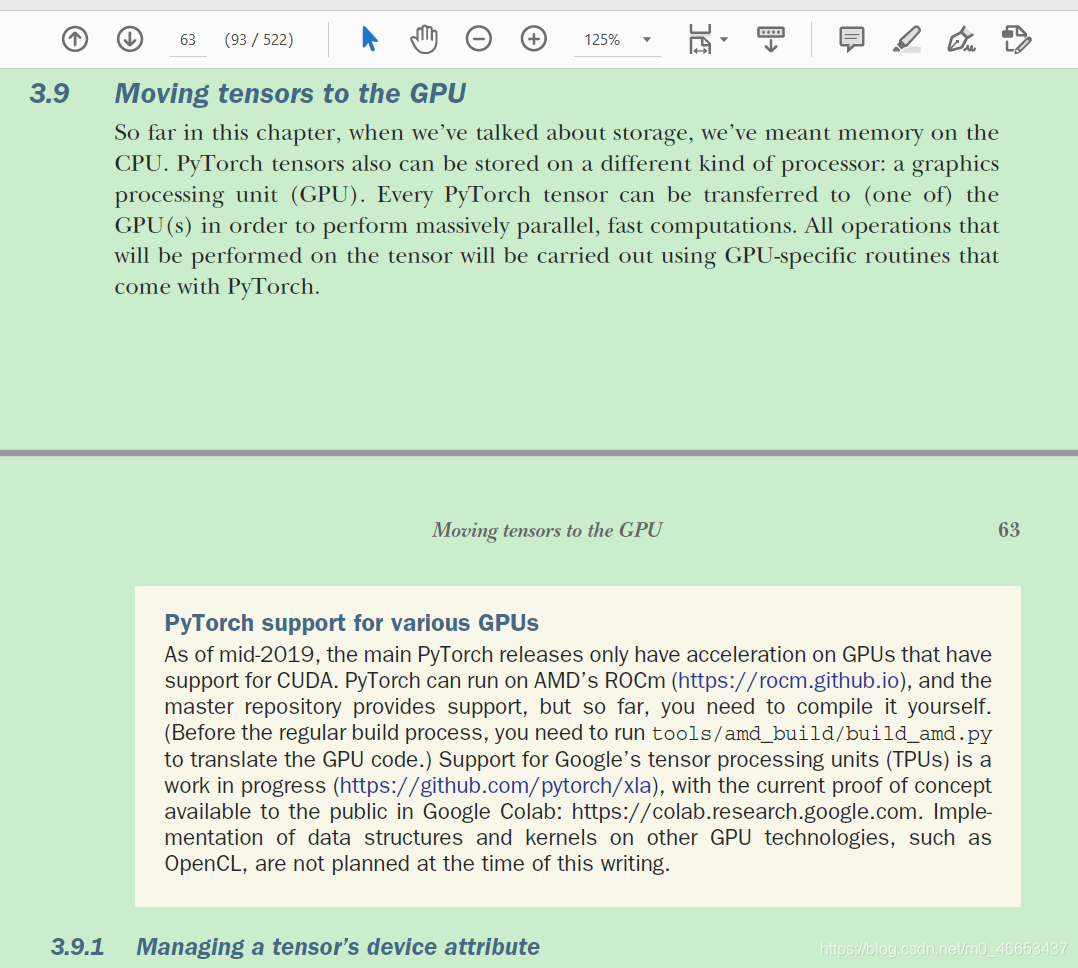
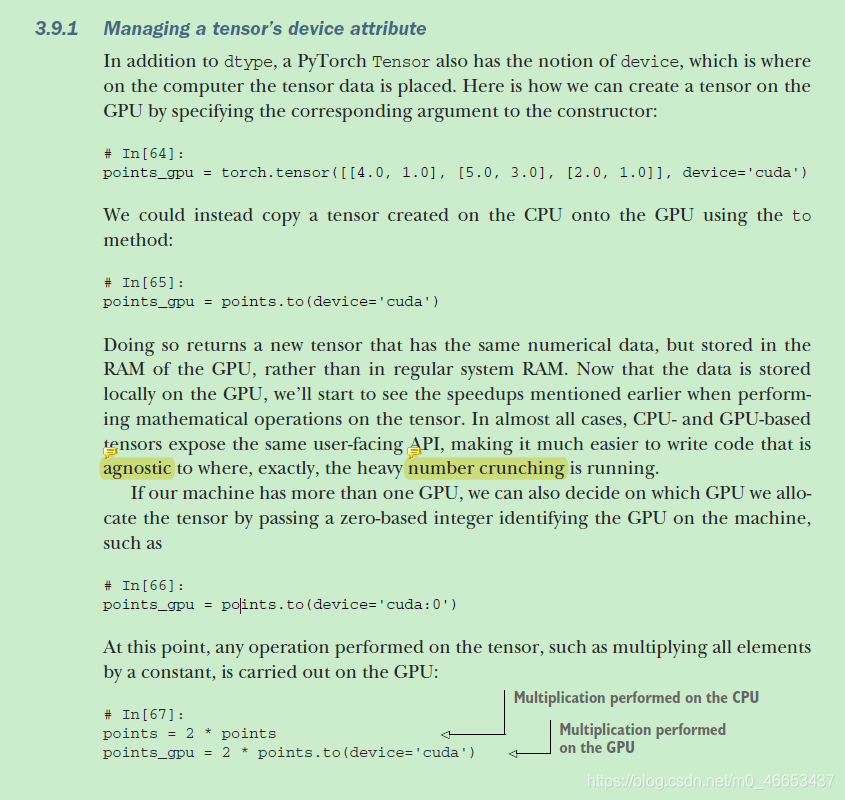
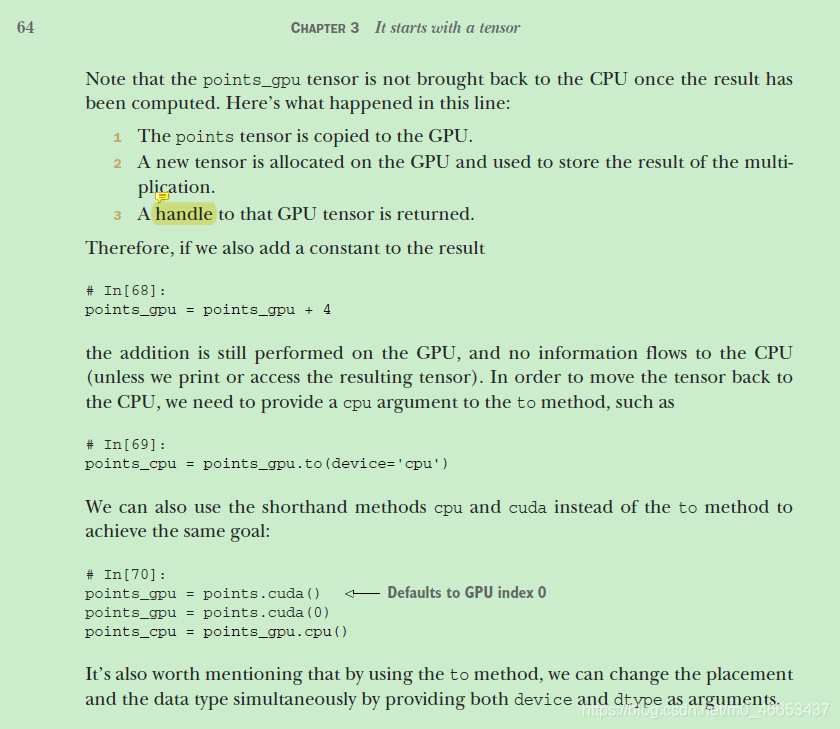
代码实验:
Microsoft Windows [版本 10.0.18363.1316]
(c) 2019 Microsoft Corporation。保留所有权利。
C:\Users\chenxuqi>conda activate ssd4pytorch1_2_0
(ssd4pytorch1_2_0) C:\Users\chenxuqi>python
Python 3.7.7 (default, May 6 2020, 11:45:54) [MSC v.1916 64 bit (AMD64)] :: Anaconda, Inc. on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import torch
>>> torch.manual_seed(seed=20200910)
<torch._C.Generator object at 0x000001AF9D28D330>
>>>
>>> torch.randn((2,3), device='cuda')
tensor([[-0.9908, 0.5920, -0.0895],
[ 0.7582, 0.0068, -3.2594]], device='cuda:0')
>>> torch.randn((2,3), device='cuda0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
RuntimeError: Expected one of cpu, cuda, mkldnn, opengl, opencl, ideep, hip, msnpu device type at start of device string: cuda0
>>> torch.randn((2,3), device='cuda:0')
tensor([[ 1.2275, -0.4505, 0.2995],
[ 0.6775, 0.2833, 1.3016]], device='cuda:0')
>>> torch.randn((2,3), device='cpu')
tensor([[ 0.2824, -0.3715, 0.9088],
[-1.7601, -0.1806, 2.0937]])
>>> torch.randn((2,3))
tensor([[ 1.0406, -1.7651, 1.1216],
[ 0.8440, 0.1783, 0.6859]])
>>>
>>>
>>>