1. 工具类 AsposeUtil
@Component
@Slf4j
public class AsposeUtil {
private static final String[] WORD = {"doc", "docx", "wps", "wpt", "txt"};
private static final String[] EXCEL = {"xls", "xlsx", "et", "xlsm"};
private static final String[] PPT = {"ppt", "pptx"};
private static final String[] PDF = {"pdf"};
private static final String[] IMG = {"bmp", "jpg", "png", "tif", "gif", "pcx", "tga", "exif", "fpx", "svg", "psd", "cdr", "pcd", "dxf", "ufo", "eps", "ai", "raw", "WMF", "webp", "avif", "apng"};
private static final String TYPE_UNSUPPORT = "不支持的格式";
private static final String TYPE_WORD = "TYPE_WORD";
private static final String TYPE_EXCEL = "TYPE_EXCEL";
private static final String TYPE_PPT = "TYPE_PPT";
private static final String TYPE_PDF = "TYPE_PDF";
private static final String TYPE_IMG = "TYPE_IMG";
@Autowired
OssUtil ossUtil;
private boolean judgeLicense() {
boolean result = false;
try {
// InputStream is = AsposeUtil.class.getClassLoader().getResourceAsStream("license.xml");
String license =
"<License>\n" +
" <Data>\n" +
" <Products>\n" +
" <Product>Aspose.Total for Java</Product>\n" +
" <Product>Aspose.Words for Java</Product>\n" +
" </Products>\n" +
" <EditionType>Enterprise</EditionType>\n" +
" <SubscriptionExpiry>20991231</SubscriptionExpiry>\n" +
" <LicenseExpiry>20991231</LicenseExpiry>\n" +
" <SerialNumber>8bfe198c-7f0c-4ef8-8ff0-acc3237bf0d7</SerialNumber>\n" +
" </Data>\n" +
" <Signature>sNLLKGMUdF0r8O1kKilWAGdgfs2BvJb/2Xp8p5iuDVfZXmhppo+d0Ran1P9TKdjV4ABwAgKXxJ3jcQTqE/2IRfqwnPf8itN8aFZlV3TJPYeD3yWE7IT55Gz6EijUpC7aKeoohTb4w2fpox58wWoF3SNp6sK6jDfiAUGEHYJ9pjU=</Signature>\n" +
"</License>";
InputStream is = new ByteArrayInputStream(license.getBytes("UTF-8"));
License aposeLic = new License();
aposeLic.setLicense(is);
result = true;
} catch (Exception e) {
log.error("Aspose License Error,error:{}",e);
}
return result;
}
/**
* 根据文件名判断文件类型
*
* @return
*/
private String getType(String fileName) {
String suffix = fileName.substring(fileName.lastIndexOf(".") + 1); // 后缀
if (CollUtil.contains(Arrays.asList(WORD), suffix)) {
return TYPE_WORD;
} else if (CollUtil.contains(Arrays.asList(EXCEL), suffix)) {
return TYPE_EXCEL;
} else if (CollUtil.contains(Arrays.asList(PPT), suffix)) {
return TYPE_PPT;
} else if (CollUtil.contains(Arrays.asList(PDF), suffix)) {
return TYPE_PDF;
} else if (CollUtil.contains(Arrays.asList(IMG), suffix)) {
return TYPE_IMG;
} else {
return TYPE_UNSUPPORT;
}
}
/**
* 文件转化pdf
*
* @param fileName 传入的文件名称
* @param addr 传入的文件地址
* @return 转换后的pdf地址 或 格式不支持预览
*/
public String toPdf(String fileName, String addr) throws Exception {
if (!judgeLicense()) {
throw new BizException(BuErrorEnum.ASPOSE_LISENSE_PROBLEM.getErrCode(), BuErrorEnum.ASPOSE_LISENSE_PROBLEM.getErrMsg());
}
String type = getType(fileName);
switch (type) {
case TYPE_UNSUPPORT:
return TYPE_UNSUPPORT;
case TYPE_PDF:
return addr;
case TYPE_IMG:
return addr;
default:
break;
}
InputStream in = URLUtil.getStream(URLUtil.url(addr)); // 下载文件
ByteArrayOutputStream tmp = new ByteArrayOutputStream();
switch (type) {
case TYPE_WORD:
tmp = wordToPdfStream(in);
break;
case TYPE_EXCEL:
tmp = excelToPdfStream(in);
break;
case TYPE_PPT:
tmp = pptToPdfStream(in);
break;
default:
break;
}
PdfMultipartFile pdfMultipartFile = new PdfMultipartFile(fileName, new ByteArrayInputStream(tmp.toByteArray()));
return ossUtil.uploadFile(pdfMultipartFile);
}
private ByteArrayOutputStream wordToPdfStream(InputStream in) throws Exception {
Document doc = new Document(in);
ByteArrayOutputStream dstStream = new ByteArrayOutputStream();
doc.save(dstStream, com.aspose.words.SaveFormat.PDF);
return dstStream;
}
private ByteArrayOutputStream excelToPdfStream(InputStream in) throws Exception {
Workbook excel = new Workbook (in);
ByteArrayOutputStream dstStream = new ByteArrayOutputStream();
excel.save(dstStream, com.aspose.cells.SaveFormat.PDF);
return dstStream;
}
private ByteArrayOutputStream pptToPdfStream(InputStream in) throws Exception {
Presentation ppt = new Presentation (in);
ByteArrayOutputStream dstStream = new ByteArrayOutputStream();
ppt.save(dstStream, SaveFormat.Pdf);
return dstStream;
}
}
public class PdfMultipartFile implements MultipartFile {
private final String name;
private final String originalFilename;
@Nullable
private final String contentType;
private final byte[] content;
public PdfMultipartFile(String name, @Nullable byte[] content) {
this(name, "", null, content);
}
public PdfMultipartFile(String name, InputStream contentStream) throws IOException {
this(name, "", null, FileCopyUtils.copyToByteArray(contentStream));
}
public PdfMultipartFile(
String name, @Nullable String originalFilename, @Nullable String contentType, @Nullable byte[] content) {
Assert.hasLength(converName(name), "Name must not be empty");
this.name = converName(name);
this.originalFilename = (originalFilename != null ? originalFilename : "");
this.contentType = contentType;
this.content = (content != null ? content : new byte[0]);
}
public PdfMultipartFile(
String name, @Nullable String originalFilename, @Nullable String contentType, InputStream contentStream)
throws IOException {
this(name, originalFilename, contentType, FileCopyUtils.copyToByteArray(contentStream));
}
private String converName(String name) {
int index = name.lastIndexOf(".");
if (index > 0) {
String suffix = name.substring(index).toLowerCase();
return name.replace(suffix, ".pdf");
}
return name + ".pdf";
}
@Override
public String getName() {
return this.name;
}
@Override
@NonNull
public String getOriginalFilename() {
return this.originalFilename == null || this.originalFilename.equals("") ? this.name : this.originalFilename;
}
@Override
@Nullable
public String getContentType() {
return this.contentType;
}
@Override
public boolean isEmpty() {
return (this.content.length == 0);
}
@Override
public long getSize() {
return this.content.length;
}
@Override
public byte[] getBytes() throws IOException {
return this.content;
}
@Override
public InputStream getInputStream() throws IOException {
return new ByteArrayInputStream(this.content);
}
@Override
public void transferTo(File dest) throws IOException, IllegalStateException {
FileCopyUtils.copy(this.content, dest);
}
}
2. license文件
<License>
<Data>
<Products>
<Product>Aspose.Total for Java</Product>
<Product>Aspose.Words for Java</Product>
</Products>
<EditionType>Enterprise</EditionType>
<SubscriptionExpiry>20991231</SubscriptionExpiry>
<LicenseExpiry>20991231</LicenseExpiry>
<SerialNumber>8bfe198c-7f0c-4ef8-8ff0-acc3237bf0d7</SerialNumber>
</Data>
<Signature>
sNLLKGMUdF0r8O1kKilWAGdgfs2BvJb/2Xp8p5iuDVfZXmhppo+d0Ran1P9TKdjV4ABwAgKXxJ3jcQTqE/2IRfqwnPf8itN8aFZlV3TJPYeD3yWE7IT55Gz6EijUpC7aKeoohTb4w2fpox58wWoF3SNp6sK6jDfiAUGEHYJ9pjU=
</Signature>
</License>
3. jar包
4. 本地pom引入
<!--aspose start-->
<dependency>
<groupId>com.aspose</groupId>
<artifactId>slides</artifactId>
<version>15.9.0</version>
<scope>system</scope>
<systemPath>${project.basedir}/lib/aspose.slides-15.9.0.jar</systemPath>
</dependency>
<dependency>
<groupId>aspose</groupId>
<artifactId>cells</artifactId>
<version>8.5.2</version>
<scope>system</scope>
<systemPath>${project.basedir}/lib/aspose-cells-8.5.2.jar</systemPath>
</dependency>
<dependency>
<groupId>aspose</groupId>
<artifactId>words</artifactId>
<version>16.8.0</version>
<scope>system</scope>
<systemPath>${project.basedir}/lib/aspose-words-16.8.0-jdk16.jar</systemPath>
</dependency>
<!--aspose end-->
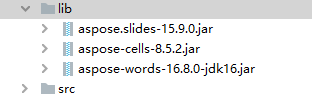