1、求最大值
题目描述:输入两个整数,比较大小并输出“max=”,之后输出较大的值。
代码实现:
#include <iostream>
using namespace std;
int main()
{
int num1, num2;
cin>>num1>>num2;
num1 > num2 ? cout<<num1 : cout<<num2;
return 0;
}
执行结果: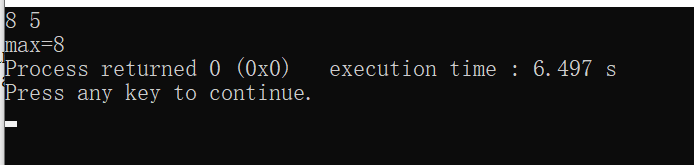
2、求圆面积
题目描述:定义一个circle圆类,有浮点型的半径属性和计算面积行为。PI值定为常量3.14。从键盘接收一个半径值并创建相应的circle对象,输出这个圆的面积。
代码实现:
#include <iostream>
using namespace std;
const float PI = 3.14;
class circle{
public:
circle(float a)
{
r = a;
}
void area()
{
cout<<PI*r*r<<endl;
}
private:
float r;
};
int main()
{
float r;
cin>>r;
circle crl(r);
crl.area();
return 0;
}
执行结果:
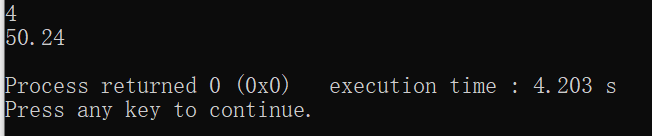
3、编写重载函数来打印字符串
题目描述:编写函数 print_spaced 来打印字符串,要求打印出的字符串每个字母之间都有一个空格。要求编写两个同名函数,一个支持字符数组输入,另一个支持string类型输入。然后编写main函数测试这两个函数,第一个使用字符数组输入,第二个使用string类型输入。
函数原型为:void print_spaced(char s[]) ; void print_spaced(string s) ;
代码实现:
#include <iostream>
#include <string.h>
using namespace std;
void print_spaced(char s[]);
void print_spaced(string s);
void print_spaced(char s[])
{
int i = 0;
while(s[i] != '\0')
{
cout<<s[i];
if((i != strlen(s)-1))
{
cout<<" ";
}
i++;
}
cout<<endl;
}
void print_spaced(string s)
{
for(int i = 0; i < s.length(); i++)
{
cout<<s[i];
if((i != s.length()-1))
{
cout<<" ";
}
}
}
int main()
{
char s1[101];
string str;
cin>>s1;
print_spaced(s1);
cin>>str;
print_spaced(str);
return 0;
}
运行结果:
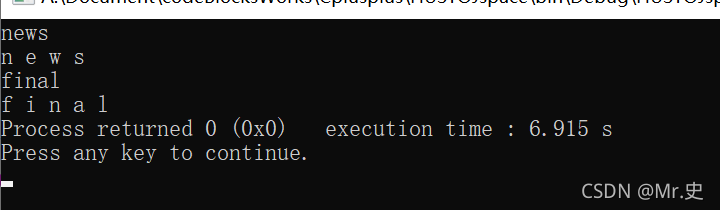
4、设计带构造函数的Dog类
题目描述:设计一个Dog类,包含name、age、sex和weight等属性,在有参数的构造函数中对数据成员进行初始化。
公有成员函数有:GetName()、GetAge()、GetSex()和GetWeight()可获取名字、年龄、性别和体重。编写成员函数speak() 显示狗的叫声(Arf!Arf!)。编写主函数,输入狗的名字、年龄、性别和体重;声明Dog对象并用输入的数据通过构造函数初始化对象,通过成员函数获取狗的属性并显示出来。
代码实现:
#include <iostream>
using namespace std;
class Dog{
public:
Dog(string names, int ages, string sexs, float weights)
{
name = names;
age = ages;
sex = sexs;
weight = weights;
}
string GetName();
int GetAge();
string GetSex();
float GetWeight();
void speak();
private:
string name;
int age;
string sex;
float weight;
};
string Dog::GetName()
{
return name;
}
int Dog::GetAge()
{
return age;
}
string Dog::GetSex()
{
return sex;
}
float Dog::GetWeight()
{
return weight;
}
void Dog::speak()
{
cout<<"Arf!Arf!"<<endl;
}
int main()
{
string name;
int age;
float weight;
string sex;
cin>>name>>age>>sex>>weight;
Dog dog(name, age, sex, weight);
cout<<dog.GetName()<<endl;
cout<<dog.GetAge()<<endl;
cout<<dog.GetSex()<<endl;
cout<<dog.GetWeight()<<endl;
dog.speak();
return 0;
}
运行结果:
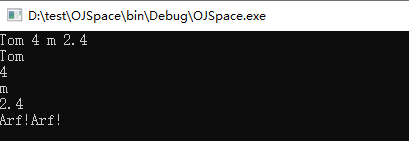
5、定义一个带重载构造函数的日期类
题目描述:定义一个带重载构造函数的日期类Date,数据成员有年、月、日;成员函数包括:一个带参数的构造函数Date(int,int,int),一个不带参数的构造函数(设置默认值为1900-1-1),一个按“年-月-日”格式显示日期的函数void show(),一个对数据成员赋值的函数void init(int,int,int)。
主函数中对类的测试要求:
- 分别使用两个不同的重载构造函数创建两个日期类对象(必须为d1,d2,d2初始值为2100-12-12);
- 按“年-月-日”格式分别显示两个对象的值;
- 输入数据,用init函数为d1赋值;
2.按“年-月-日”格式显示对象d1的值;
代码实现:
#include <iostream>
using namespace std;
class Date{
public:
Date(int, int, int);
Date();
void show();
void init(int,int,int);
private:
int year;
int mouth;
int day;
};
Date::Date()
{
year = 1900;
mouth = 1;
day = 1;
}
Date::Date(int years, int mouths, int days)
{
year = years;
mouth = mouths;
day = days;
}
void Date::show()
{
cout<<year<<"-"<<mouth<<"-"<<day<<endl;
}
void Date::init(int years, int mouths, int days)
{
year = years;
mouth = mouths;
day = days;
}
int main()
{
int y;
int m;
int d;
Date d1;
Date d2(2100, 12, 12);
d1.show();
d2.show();
cin>>y>>m>>d;
d1.init(y, m, d);
d1.show();
return 0;
}
运行结果:
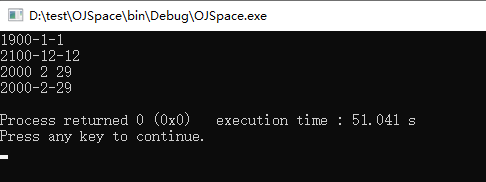
6、 C++第三章——指针,动态生成Person类的对象
题目描述:编写Person类,数据成员为姓名(20字符长度)、年龄(int)和性别(char)。
编写无参数的构造函数,其中姓名赋值为“XXX”,年龄0,性别m;
编写析构函数,在其中输出字符串“Now destroying the instance of Person”;
编写Register成员函数,为数据成员赋值;
编写showme成员函数,显示姓名、年龄和性别。
编写主函数:
用Person类创建2个指针,p1和 p2;
用new创建两个Person对象,分别将指针赋值给p1,p2;
用showme成员函数显示p1,p2所指对象的值;
再输入一组“姓名、年龄和性别”值,用成员函数Register为p1的成员赋值;
将p1所指对象的值赋值给p2所指对象;
用showme显示p1、p2所指对象的值。
删除动态对象。
代码实现:
#include <iostream>
#include <string.h>
using namespace std;
class Person{
public:
Person();
~Person();
void Register(char names[20], int ages, char sexs);
void showme();
private:
char name[20];
int age;
char sex;
};
Person::Person()
{
strcpy(name, "XXX");
age = 0;
sex = 'm';
}
Person::~Person()
{
cout<<"Now destroying the instance of Person"<<endl;
}
void Person::Register(char names[20], int ages, char sexs)
{
//将字符串names 赋值给name
memcpy(name, names, strlen(names));
age = ages;
sex = sexs;
}
void Person::showme()
{
cout<<name<<" "<<age<<" "<<sex<<endl;
}
int main()
{
char name[20];
int ages;
char sexs;
Person *p1, *p2;
p1 = new Person();
p2 = new Person();
cout<<"person1:";
p1->showme();
cout<<"person2:";
p2->showme();
cin>> name >> ages >> sexs;
p1->Register(name, ages, sexs);
cout<<"person1:";
p1->showme();
*p2 = *p1;
cout<<"person2:";
p2->showme();
delete p1;
delete p2;
return 0;
}
运行结果:
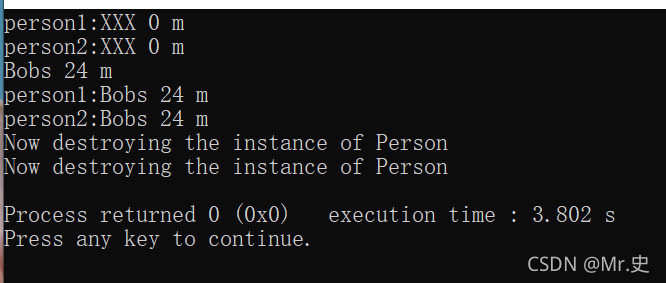
7、编写一个小小的计算器,能够计算两个浮点数的加、减、乘、除。
每行是一个测试用例,每行的内容为:操作数1 运算符 操作数2,操作数为浮点数,运算符为+、-、*、/,操作数和运算符之间有一个空格隔开。
对每个测试用例,输出一行计算结果,保留两位小数的精度。如果除法遇到操作数2为0,输出“Divide by zero."。
代码实现:
#include <iostream>
#include <iomanip>
//需导入iomanip库 才能使用cout<< fixed <<setprecision(2);
using namespace std;
class Calculator{
public:
Calculator(double inum1, char ioper, double inum2)
{
num1 = inum1;
oper = ioper;
num2 = inum2;
}
void calculate()
{
cout<< fixed <<setprecision(2);
switch(oper)
{
case '+' :
cout<< num1 + num2 <<endl;
break;
case '-':
cout<< num1 - num2 <<endl;
break;
case '*':
cout<< num1 * num2 <<endl;
break;
case '/':
if(num2 == 0)
{
cout<< "Divide by zero." <<endl;
break;
}
cout<< num1 / num2 <<endl;
break;
default:
cout<<"你输入有误" <<endl;
}
}
private:
double num1, num2;
char oper;
};
int main()
{
double num1, num2;
char oper;
cin>>num1>>oper>>num2;
Calculator cal(num1, oper, num2);
cal.calculate();
cin>>num1>>oper>>num2;
Calculator cal2(num1, oper, num2);
cal2.calculate();
cin>>num1>>oper>>num2;
Calculator cal3(num1, oper, num2);
cal3.calculate();
cin>>num1>>oper>>num2;
Calculator cal4(num1, oper, num2);
cal4.calculate();
return 0;
}
运行结果:
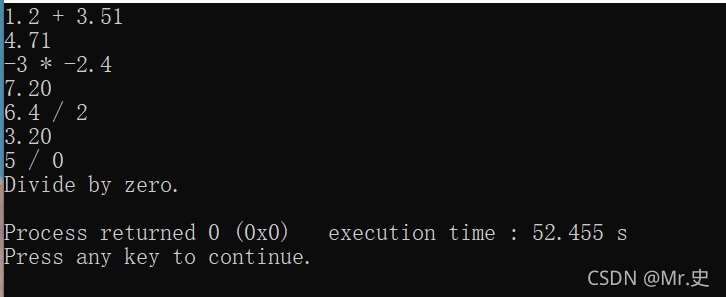
8、设计一个多功能的MyTime类
题目描述:设计一个多功能的MyTime类,设计多个重载的构造函数,可以设置时间,进行时间的加减运算,按各种可能的格式(24小时制、12小时制)输出时间。
注意:
(1)请考虑设置的时间的合理性(时0-23; 分0-59;秒0-59)。
(2)12小时制中,12:00:00前为AM, 12:00:00及以后为PM
(3)加减运算的加数、减数是一个时间的长度,单位为“时、分、秒”
(4)构造函数:没参数时,设置时间为0时 0分 0秒;有参数时,设置成给定的时、分、秒。
在主函数中
(1)声明两个对象t1,t2,并通过构造函数初始化它们(t2初始化为为8:10:30)
(2)显示按12、14小时制显示t1、t2的时间。
(3)再设置t1的时间,数据由用户输入。
(4)再输入待加减的时间。
(5)t1加输入的时间,并按12小时和24小时制显示。
(6)t2减输入的时间,并按12小时和24小时制显示。
输入格式:
第一行为t1的时间,第二行为待加减的时间
输出格式:
显示按12、14小时制显示t1、t2的初始时间
t1加输入的待加减的时间按12小时和24小时制显示。
t2减输入的待加减的时间按12小时和24小时制显示
输入样例:
11 30 30
5 15 20
输出样例:
00:00:00 AM
00:00:00
08:10:30 AM
08:10:30
04:45:50 PM
16:45:50
02:55:10 AM
02:55:10
代码实现:
#include<iostream>
using namespace std;
class MyTime
{
private:
int hour;
int minute;
int second;
public:
MyTime()
{
hour = 0;
minute = 0;
second = 0;
}
MyTime(int _hour, int _minute, int _second)
{
hour = _hour;
minute = _minute;
second = _second;
}
void SetTime(int _hour, int _minute, int _second)
{
hour = _hour;
minute = _minute;
second = _second;
}
void addTime(int h,int m,int s)
{
hour += h;
minute += m;
second += s;
if(second>=60)
{
second = second -60;
minute = minute + 1;
}
if(minute>=60)
{
minute = minute - 60;
hour = hour + 1;
}
if(hour>=24)
{
hour = hour -24;
}
}
void reduceTime(int _h,int _m,int _s)
{
if(second<_s)
{
second += 60;
minute --;
}
if(minute<_m)
{
minute += 60;
hour --;
}
if(hour<_h)
{
hour = hour + 24;
}
hour -= _h;
minute -= _m;
second -= _s;
}
void print_12()
{
int tempHour;
hour>=12?tempHour=hour-12:tempHour=hour;
if(tempHour<10){
cout<<'0';
}
cout<<tempHour<<':';
if(minute<10){
cout<<'0';
}
cout<<minute<<':';
if(second<10){
cout<<'0';
}
cout<<second;
if(hour>12||((hour==12)&&((minute>0)||(second>0)))){
cout<<" "<<"PM"<<endl;
}else{
cout<<" "<<"AM"<<endl;
}
}
void print_24()
{
if(hour<10){
cout<<'0';
}
cout<<hour<<':';
if(minute<10){
cout<<'0';
}
cout<<minute<<':';
if(second<10){
cout<<'0';
}
cout<<second<<endl;
}
};
int main()
{
MyTime t1;
MyTime t2(8,10,30);
t1.print_12();
t1.print_24();
t2.print_12();
t2.print_24();
int h1,m1,s1,h2,m2,s2;
cin>>h1>>m1>>s1;
t1.SetTime(h1, m1, s1);
cin>>h2>>m2>>s2;
t1.addTime(h2,m2,s2);
t2.reduceTime(h2, m2, s2);
t1.print_12();
t1.print_24();
t2.print_12();
t2.print_24();
return 0;
}
运行结果:
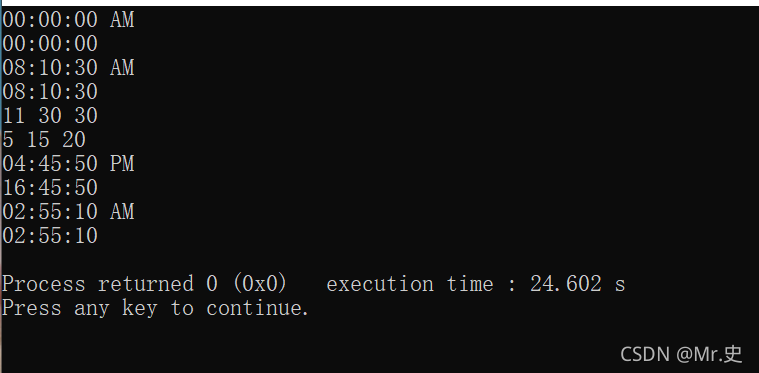
9、面向对象之存折类
题目描述:请定义“存折”类,及其相关的客户端代码。请注意编写正确的构造函数。存折类的基本情况如下:
Type name:AccountType
Domain:
Id,name,balance;
Operations:
Deposit, 存钱操作;
Withdraw,取钱操作;
getBalance,获取余额操作;
WriteAccount,打印帐户信息;
Input:
13001 peter 0
1200 //存的钱
500 //取的钱
Output:
13001 peter 700
代码实现:
#include <iostream>
#include <string>
using namespace std;
class AccountType{
public:
AccountType(int, string, int );
//存钱操作
void Deposit(int ibalance);
//取钱操作
int Withdraw(int ibalance);
//获取余额操作
int getBalance();
//打印帐户信息
void WriteAccount();
private:
int id;
string name;
int balance;
};
AccountType::AccountType(int iid, string iname, int ibalance)
{
id = iid;
name = iname;
balance = ibalance;
}
//存钱操作
void AccountType::Deposit(int ibalance)
{
balance += ibalance;
}
//取钱操作
int AccountType::Withdraw(int ibalance)
{
balance -= ibalance;
return balance;
}
//获取余额操作
int AccountType::getBalance()
{
return balance;
}
//打印帐户信息
void AccountType::WriteAccount()
{
cout<< id <<" "<< name <<" "<< balance;
}
int main(int argc, char* argv[]){
int id;
string name;
int balance;
cin>> id >> name >> balance;
AccountType accountType(id, name, balance);
cin>>balance;
accountType.Deposit(balance);
cin>>balance;
accountType.Withdraw(balance);
accountType.getBalance();
accountType.WriteAccount();
return 0;
}
运行结果: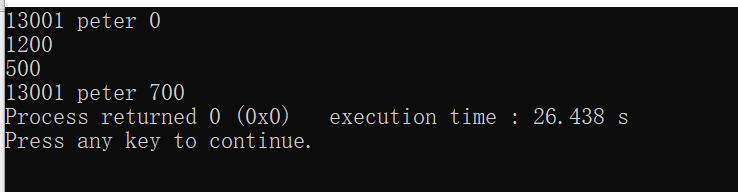
10.User类
题目描述:
设计并实现一个用户类User,并在主函数中使用和测试这个类。每一个用户有用户名(name),密码(passwd),联系邮箱(email)三个属性。 支持设置用户信息setInfo()。允许设置信息时密码默认为6个1,联系邮箱默认为空串。 支持打印用户信息printInfo()。打印用户名、密码、联系邮箱。其中,密码以6个*方式显示。 支持修改邮箱changeEmail()。支持修改密码changePassword()。在修改密码前,要求先输入旧密码,验证无误后,才允许修改。 如果输入旧密码时,连续三次输入错误,则提示用户稍后再试,暂时退出修改密码程序。 在main()函数中创建User类对象,测试User类的各项操作(设置用户信息,修改邮箱,修改密码,打印用户信息)。
代码实现:
#include <iostream>
#include <string>
using namespace std;
class User{
public:
//设置用户信息
//允许设置信息时密码默认为6个1,联系邮箱默认为空串。
void setInfo(string name, string passwd = "111111", string email = "");
//打印用户名、密码、联系邮箱。
//其中,密码以6个*方式显示
void printInfo();
//支持修改邮箱
void changeEmail(string email);
//支持修改密码
//在修改密码前,要求先输入旧密码,验证无误后,才允许修改。
//如果输入旧密码时,连续三次输入错误,则提示用户稍后再试,暂时退出修改密码程序。
bool changePassword(string new_passwd, string old_passwd);
private:
string m_name;
string m_passwd;
string m_email;
};
//设置用户信息
//允许设置信息时密码默认为6个1,联系邮箱默认为空串。
void User::setInfo(string name, string passwd , string email)
{
m_name = name;
m_passwd = passwd;
m_email = email;
}
//打印用户名、密码、联系邮箱。
//其中,密码以6个*方式显示
void User::printInfo()
{
cout<<"用户名:"<< m_name << " 密码:" << "******" <<" 邮箱:" << m_email<<endl;
}
//支持修改邮箱
void User::changeEmail(string email)
{
m_email = email;
}
//支持修改密码
//在修改密码前,要求先输入旧密码,验证无误后,才允许修改。
//如果输入旧密码时,连续三次输入错误,则提示用户稍后再试,暂时退出修改密码程序。
bool User::changePassword(string new_passwd, string old_passwd)
{
if(old_passwd != m_passwd)
{
for(int i = 2; i > 0; i--)
{
cout<<"请重新输入原密码你还有"<< i <<"次机会"<<endl;
cin>>old_passwd;
if(old_passwd == m_passwd)
{
m_passwd = new_passwd;
return true;
}
}
return false;
}
m_passwd = new_passwd;
return true;
}
int main()
{
User user;
user.setInfo("Tom");
user.printInfo();
user.setInfo("Tom", "111111", "tom@163.com");
user.printInfo();
user.changeEmail("js@163.com");
user.printInfo();
if(!user.changePassword("222222", "000000"))
{
cout<<"修改密码失败!";
}
return 0;
}
运行结果1:
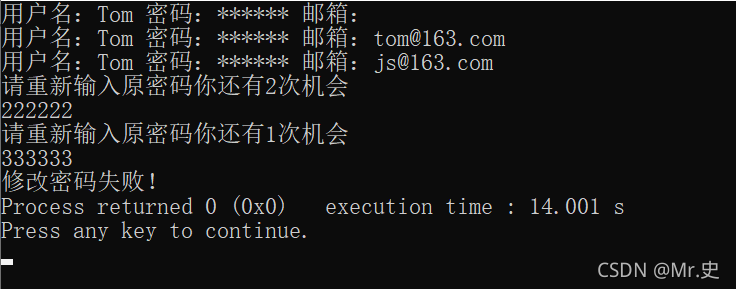
运行结果2:
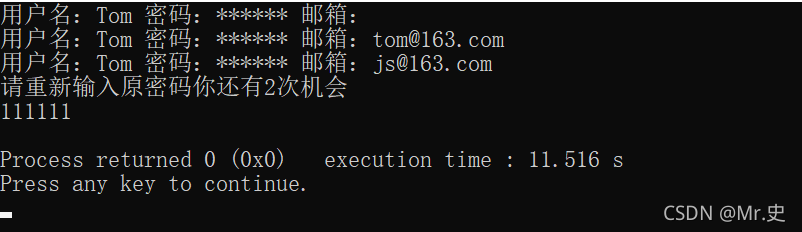
11.定义一个图书类Book 对象数组应用
题目描述:
定义一个图书类Book,
数据成员包含name(书名)、author(作者)、price(定价),
为该类定义构造函数,
并提供各数据成员的getXXX()和setXXX()函数。
在main函数中创建图书对象数组,
将对应输入值依次输出。
输入:
5
谭浩强 C语言教程 55.55
谭浩强 C++教程 33.44
李春葆 数据结构 35.80
李春版 数据结构java版本 45.7
李春版 数据结构python版本 39.9
输出:
name=谭浩强,author=C语言教程,price=55.55
name=谭浩强,author=C++教程,price=33.44
name=李春葆,author=数据结构,price=35.8
name=李春版,author=数据结构java版本,price=45.7
name=李春版,author=数据结构python版本,price=39.9
代码实现:
#include <iostream>
#include <string>
using namespace std;
class Book{
public:
Book()
{
}
Book(string _name, string _author, float _price);
string getName();
string getAuthor();
float getPrice();
void setName(string _name);
void setAuthor(string _author);
void setPrice(float _price);
private:
string name;
string author;
float price;
};
Book::Book(string _name, string _author, float _price)
{
name = _name;
author = _author;
price = _price;
}
string Book::getName()
{
return name;
}
string Book::getAuthor()
{
return author;
}
float Book::getPrice()
{
return price;
}
void Book::setName(string _name)
{
name = _name;
}
void Book::setAuthor(string _author)
{
author = _author;
}
void Book::setPrice(float _price)
{
price = _price;
}
int main()
{
int i;
string name;
string author;
float price;
cin>>i;
Book book[i];
for(int j = 0; j<i; j++)
{
cin>>name>>author>>price;
book[j].setName(name);
book[j].setAuthor(author);
book[j].setPrice(price);
}
for(int j = 0; j<i; j++)
{
cout<<"name="<< book[j].getName() <<",author="<< book[j].getAuthor()<<",price="<< book[j].getPrice()<<endl;
}
return 0;
}
运行结果:
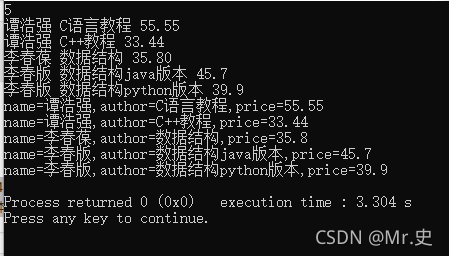
12.定义一个交通工具的类
题目描述:
定义一个交通工具(Vehicle)的类,
其中有数据成员:
速度(speed),体积(volume)等等,
其中有成员函数:
构造函数
析构函数
显示速度和体积值(show()),
设置速度(setSpeed(int speed)),
加速speedUp(int upspeed),减速speedDown(int downspeed)等等。
在main函数中创建一个交通工具对象,通过成员函数设置speed, volume的值,并且通过成员函数打印出来。
另外,调用加速,减速的方法对速度进行改变。
输入:
5 //循环次数
33 //加速度
22 //减速度
输出:
Vehicle is running!
speed = 11 volume = 1
~Vehicle is running!
Vehicle is running!
speed = 12 volume = 2
~Vehicle is running!
Vehicle is running!
speed = 13 volume = 3
~Vehicle is running!
Vehicle is running!
speed = 14 volume = 4
~Vehicle is running!
Vehicle is running!
speed = 15 volume = 5
~Vehicle is running!
代码实现:
#include <iostream>
#include <string>
using namespace std;
class Vehicle{
public:
Vehicle();
~Vehicle();
void show();
void setSpeed(int speed);
void setVolume(int volume);
void speedUp(int upspeed);
void speedDown(int downspeed);
private:
int m_speed;
int m_volume;
};
Vehicle::Vehicle()
{
m_speed = 0;
m_volume = 0;
}
Vehicle::~Vehicle()
{
}
void Vehicle::show()
{
cout<<"Vehicle is running!"<<endl;
cout<<"speed = "<< m_speed <<" volume = "<< m_volume <<endl;
cout<<"~Vehicle is running!"<<endl;
}
void Vehicle::setSpeed(int speed)
{
m_speed = speed;
}
void Vehicle::setVolume(int volume)
{
m_volume = volume;
}
void Vehicle::speedUp(int upspeed)
{
m_speed += upspeed;
}
void Vehicle::speedDown(int downspeed)
{
m_speed -= downspeed;
}
int main()
{
int j;
int upspeed;
int downspeed;
int speed;
cin>> j >> upspeed >> downspeed;
Vehicle vehicle[j];
for (int i = 0; i < j; i++)
{
vehicle[i].setSpeed(i);
vehicle[i].setVolume(i+1);
vehicle[i].speedUp(upspeed);
vehicle[i].speedDown(downspeed);
vehicle[i].show();
}
return 0;
}
运行结果:
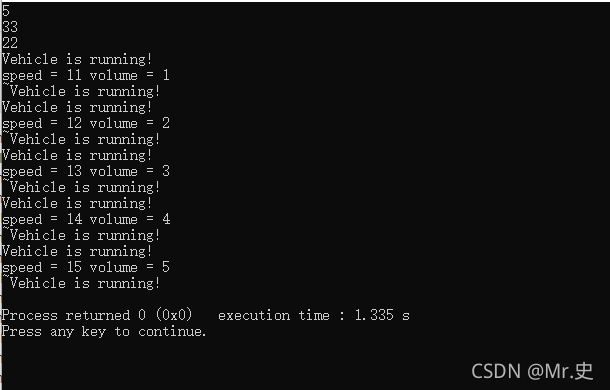
13、统计学生总成绩和平均成绩(静态成员变量)
问题描述:
编写一个程序,已有若干学生的数据,包括学号、姓名、成绩。
要求输入这些学生的基础信息(学号、姓名、成绩),
输出学生的:总人数、总成绩、平均成绩。
要求:学生总人数、总成绩、平均成绩使用静态成员变量定义。
输入:
3 //总人数
1001 user01 11.11 //学号、姓名、成绩
1002 user02 22.33
1003 user03 55.55
输出:
count = 3,total_score = 88.99,s_avg_score = 29.6633
代码实现:
#include <iostream>
using namespace std;
//编写一个程序,已有若干学生的数据,包括学号、姓名、成绩。
//要求输入这些学生的基础信息(学号、姓名、成绩),
//输出学生的:总人数、总成绩、平均成绩。
//
//
//要求:学生总人数、总成绩、平均成绩使用静态成员变量定义。
class School
{
public:
School();
School(int, string , float);
void show();
static int total_people;
static float total_score;
static float avg;
private:
int m_sno;
string m_sname;
float m_score;
};
School::School()
{
}
School::School(int sno, string sname, float score)
{
m_sno = sno;
m_sname = sname;
m_score = score;
++total_people;
total_score = total_score + m_score;
avg = total_score/total_people;
}
void School::show()
{
cout<<"count = "<< total_people <<",total_score = "<< total_score << ",s_avg_score = " << avg <<endl;
}
int School::total_people = 0;
float School::total_score = 0.0;
float School::avg = 0.0;
int main()
{ int m_sno;
string m_sname;
float m_score;
int n = 0;
int sno;
string sname;
float score;
cin>>n;
School school[n];
for(int i = 0; i < n; i++)
{
cin>>sno>>sname>>score;
school[i] = {sno, sname, score};
if(i == (n-1))
{
school[i].show();
}
}
return 0;
}
运行结果:
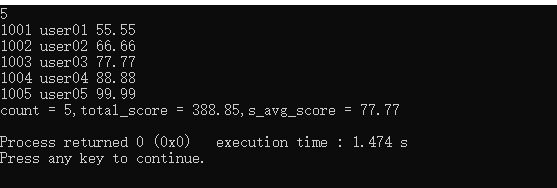
14、按要求构建stock类(this应用)
问题描述:
构建一个类Stock。
Stock 包含三个成员:
(1)字符数组 stockcode[]
(2)整形数据类型 quan
(3)双精度数据成员 price
构造函数包含三个参数:
(1)字符数组 na[]
(2) q
(3)p
当定义 Stock 对象时,对象的第一个字符串参数复制给数据成员 stockcode
第2、3个参数复制给:quan、price。
未设置第2、3个参数时,quan的值是:1000,price值:8.98
成员函数print 没有形参,需要使用 this 指针,显示对象成员的内容。
要求输入、输出如下样例所示。
输入:
3
yang 0 0.00 //如果输入0和0.00 调用默认值
wang 1111 11.11
zhou 3333 33.33
输出:
yang,1000,8.98
wang,1111,11.11
zhou,3333,33.33
代码实现:
#include <iostream>
#include <string.h>
using namespace std;
//
//构建一个类Stock。
//Stock 包含三个成员:
//(1)字符数组 stockcode[]
//(2)整形数据类型 quan
//(3)双精度数据成员 price
//构造函数包含三个参数:
//(1)字符数组 na[]
//(2) q
//(3)p
//当定义 Stock 对象时,对象的第一个字符串参数复制给数据成员 stockcode
//第2、3个参数复制给:quan、price。
//未设置第2、3个参数时,quan的值是:1000,price值:8.98
//
//成员函数print 没有形参,需要使用 this 指针,显示对象成员的内容。
//
//要求输入、输出如下样例所示。
class Stock
{
public:
Stock();
Stock(char na[], int q = 1000, double p = 8.98);
void prinf();
private:
char stockcode[20];
int quan;
double price;
};
Stock::Stock()
{
}
Stock::Stock(char na[20], int q, double p)
{
strcpy(stockcode, na);
quan = q;
price = p;
}
void Stock::prinf()
{
cout<<this->stockcode<<","<<this->quan<<","<<this->price<<endl;
}
int main()
{
int n = 0;
cin>>n;
char stockcode[20];
int quan;
double price;
Stock stock[n];
for(int i = 0; i < n; i++)
{
cin>> stockcode >> quan >> price;
if(quan == 0 && price == 0.00)
{
stock[i] = {stockcode};
continue;
}
stock[i] = {stockcode, quan, price};
}
for(int i = 0; i < n; i++)
{
stock[i].prinf();
}
return 0;
}
运行结果:
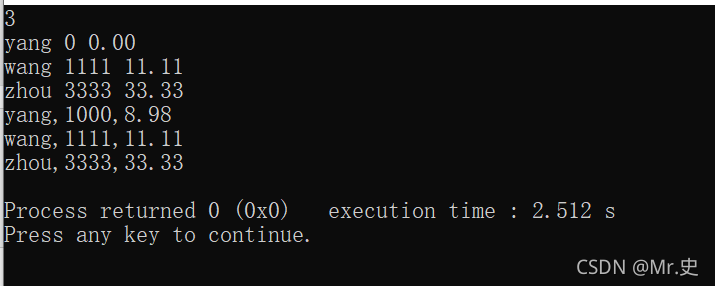
15、友元函数的应用
问题描述:
1、定义名为:Time 的时间类,
共有三个数据成员:时、分、秒。
通过构造函数实现初始化。
通过打印函数display实现成员打印。
2、定义名为:Date的日期类。
共有三个数据成员:年、月、日。
定义:Time 时间类的成员函数display 为Date日期类的友元函数。
在该友元函数中实现实现对Date数据成员的打印以及实现对原Time 自身数据成员的访问。
3、输入:分两批次。
第一次输入:时、分、秒。当时(0-24)、分(0-59)、秒(0-59)越界时,会提示“时间输入错误!!”,程序返回。
当正确后,则继续第二次输入。
第二次输入:月、天、年。当月(1-12)、天(1-31)越界时,会提示“日期输入错误!!”,程序返回。
当正确后,则继续调用display 打印输出信息。
输入:
11 59 59
10 20 2121
输出:
2121-10-20 11:59:59
输入:
25 59 59
输出:
时间输入错误!!
代码实现:
#include <iostream>
using namespace std;
class Date;
class Time
{
public:
Time(int, int, int );
void display(Date &dayD);
private:
int m_hour;
int m_minute;
int m_second;
};
Time::Time(int hour, int minute, int second)
{
m_hour = hour;
m_minute = minute;
m_second = second;
}
class Date
{
public:
Date(int year,int mouth, int day);
friend void Time::display(Date &dayD);
private:
int m_year;
int m_mouth;
int m_day;
};
Date::Date(int year,int mouth, int day)
{
m_year = year;
m_mouth = mouth;
m_day = day;
}
void Time::display(Date &dayD)
{
if(dayD.m_mouth <= 12 && dayD.m_mouth >= 0 && dayD.m_day <= 31 && dayD.m_day >= 1)
{
cout<<dayD.m_year<<"-"<<dayD.m_mouth<<"-"<<dayD.m_day<<" "<<m_hour<<":"<<m_minute<<":"<<m_second<<endl;
}
else
{
cout<<"日期输入错误!!"<<endl;
}
}
int main()
{
int hour;
int minute;
int second;
int year;
int mouth;
int day;
cin>>hour>>minute>>second;
Time time(hour, minute, second);
if(hour <24 && hour >=0 && minute <= 59 && minute >= 0 && second <= 59 && second >= 0)
{
cin>>mouth>>day>>year;
Date date(year, mouth, day);
time.display(date);
}
else
{
cout<<"时间输入错误!!"<<endl;
}
return 0;
}
运行结果1:
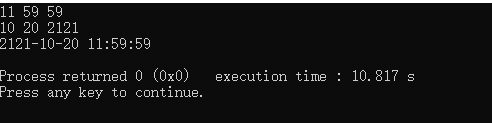
运行结果2:
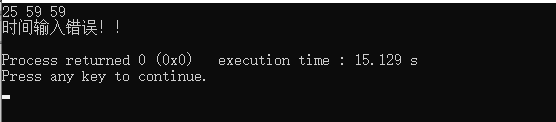
16、类的组合应用
问题描述:
1、定义一个ponit 类,有两个成员变量m_x,m_y;
有两个成员方法getx() gety()
通过构造函数实现成员变量的初始化。
-
定义一个line 线类,有三个成员变量,其中两个是point 类的对象 m_pp1, m_pp2. 另外一个是double 类型的m_len
通过构造函数的初始化成员列表的方式实现对 m_pp1, m_pp2 的初始化,
并且通过计算公式的方式,求解m_len , m_len 的求解方法= 根号下(两个类成员变量的x之差的平方+两个类成员变量的y之差的平方)
line 类有个成员函数 getLen(),用于返回 m_len
输入:
4 5 //point 类对象1的两个成员
5 6 //point 类对象2的两个成员
输出:
The result is:1.41421
代码实现:
#include <iostream>
#include <math.h>
using namespace std;
class Ponit
{
public:
Ponit(double x, double y);
double getX();
double getY();
private:
double m_x;
double m_y;
};
Ponit::Ponit(double x, double y)
{
m_x = x;
m_y = y;
}
double Ponit::getX()
{
return m_x;
}
double Ponit::getY()
{
return m_y;
}
class line
{
public:
line(double x1, double y1, double x2, double y2);
double getLine();
private:
Ponit m_pp1;
Ponit m_pp2;
double m_len;
};
line::line(double x1, double y1, double x2, double y2):m_pp1(x1, y1), m_pp2(x2, y2)
{
m_len = sqrt(pow((m_pp1.getX() - m_pp2.getX()), 2) + pow((m_pp1.getY() - m_pp2.getY()), 2) );
}
double line::getLine()
{
return m_len;
}
int main()
{
double a, b, c, d;
cin>>a>>b;
cin>>c>>d;
line lin(a, b, c, d);
cout<<"The result is:"<<lin.getLine();
return 0;
}
运行结果:

17、派生与继承之联想电脑
问题描述:
1、定义一个电脑 Computer 类,
一个成员变量:m_name
两个成员函数:setName(string name)、getName()
2、定义一个联想电脑类:LenovoComputer
一个成员变量:m_price
三个成员函数:setPrice(string price)、getPrice()、printInfo()
输入:电脑台数,比如:10台。
输出:电脑信息如下
name = Thinkpad-000, price = 1000
name = Thinkpad-001, price = 1001
name = Thinkpad-002, price = 1002
name = Thinkpad-003, price = 1003
name = Thinkpad-004, price = 1004
name = Thinkpad-005, price = 1005
name = Thinkpad-006, price = 1006
name = Thinkpad-007, price = 1007
name = Thinkpad-008, price = 1008
name = Thinkpad-009, price = 1009
price 计算方法:第1台,1000, 第2台:1000+1,依次类推。
name 名称组合生成方式:前缀”Thinkpad-“,中间0需要结合循环实现,最后是循环自增id。
输入:
15
输出:
name = Thinkpad-000, price = 1000
name = Thinkpad-001, price = 1001
name = Thinkpad-002, price = 1002
name = Thinkpad-003, price = 1003
name = Thinkpad-004, price = 1004
name = Thinkpad-005, price = 1005
name = Thinkpad-006, price = 1006
name = Thinkpad-007, price = 1007
name = Thinkpad-008, price = 1008
name = Thinkpad-009, price = 1009
name = Thinkpad-010, price = 1010
name = Thinkpad-011, price = 1011
name = Thinkpad-012, price = 1012
name = Thinkpad-013, price = 1013
name = Thinkpad-014, price = 1014
代码实现:
#include <iostream>
#include <string>
#define max 100
using namespace std;
class Computer{
public:
void setName(string name);
string getName();
private:
string m_name;
};
string Computer::getName()
{
return m_name;
}
void Computer::setName(string name)
{
m_name = name;
}
class LenovoComputer:public Computer{
public:
void setPrice(string price);
string getPrice();
void printInfo();
private:
string m_price;
};
void LenovoComputer::setPrice(string price)
{
m_price = price;
}
string LenovoComputer::getPrice()
{
return m_price;
}
void LenovoComputer::printInfo()
{
cout<<"name = Thinkpad-"<< getName() <<", price = "<<m_price<<endl;
}
//int ---> string
string to_Strings(int n)
{
int m = n;
char s[max];
char ss[max];
int i=0,j=0;
if (n < 0)// 处理负数
{
m = 0 - m;
j = 1;
ss[0] = '-';
}
while (m>0)
{
s[i++] = m % 10 + '0';
m /= 10;
}
s[i] = '\0';
i = i - 1;
while (i >= 0)
{
ss[j++] = s[i--];
}
ss[j] = '\0';
return ss;
}
//输出001 002 003
string addzero(int x)
{
if(x == 0)
{
return "000";
}
if (x<10)
{
return "00"+to_Strings(x);
}
else if (x<100)
{
return "0"+to_Strings(x);
}
}
int main()
{
int n;
cin>>n;
LenovoComputer lc;
for(int i = 0; i < n; i++)
{
lc.setName(addzero(i));
lc.setPrice(to_Strings(1000+i));
lc.printInfo();
}
return 0;
}
运行结果:
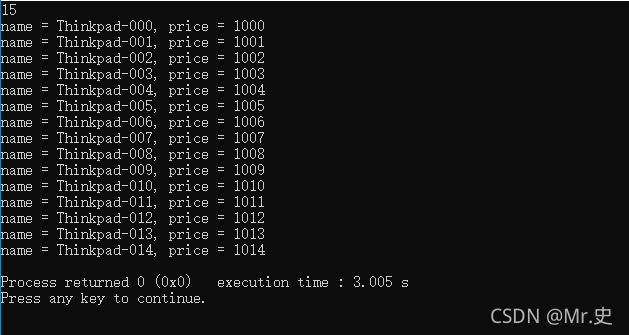
18、矩形类继承实现
问题描述:
1、定义一个Point类:
包含:两个私有成员:m_x, m_y;
包含:三个成员函数:Move(float xOff, float yOff),GetX(),GetY()
2、定义一个Rectangle继承自Point类
包含:两个私有成员 m_width, m_height;
包含:三个成员函数:GetHeight()、GetWidth()、printInfo()
要求:通过构造函数实现初始化。
输入:矩形个数。如 5
输出:矩形信息。举例如下:
X: 5,Y: 5, GetWidth: 20, GetHeight: 10
X: 6,Y: 6, GetWidth: 21, GetHeight: 11
X: 7,Y: 7, GetWidth: 22, GetHeight: 12
X: 8,Y: 8, GetWidth: 23, GetHeight: 13
X: 9,Y: 9, GetWidth: 24, GetHeight: 14
注意:X,Y width,height的初始值分别为:2,3, 20,10
后面值的变化是根据循环变量i递增的。
需要先执行 Move 函数使得X,Y变成:2+3, 3+2
输入:
5
输出:
X: 5,Y: 5, GetWidth: 20, GetHeight: 10
X: 6,Y: 6, GetWidth: 21, GetHeight: 11
X: 7,Y: 7, GetWidth: 22, GetHeight: 12
X: 8,Y: 8, GetWidth: 23, GetHeight: 13
X: 9,Y: 9, GetWidth: 24, GetHeight: 14
代码实现:
#include <iostream>
#include <string>
using namespace std;
class Point{
public:
Point(float x, float y);
void Move(float xOff, float yOff);
float GetX();
float GetY();
private:
float m_x = 2;
float m_y = 3;
};
Point::Point(float x, float y)
{
m_x = x;
m_y = y;
}
void Point::Move(float xOff, float yOff)
{
m_x = xOff + 3;
m_y = yOff + 2;
}
float Point::GetX()
{
return m_x;
}
float Point::GetY()
{
return m_y;
}
class Rectangle:public Point{
public:
Rectangle(float x, float y, float width, float height);
float GetWidth();
float GetHeight();
void printInfo();
private:
float m_width;
float m_height;
};
//父类构造函数必须在子类中初始化
Rectangle::Rectangle(float x = 2, float y = 3,float width = 20, float height = 10):Point(x, y)
{
m_width = width;
m_height = height;
}
float Rectangle::GetWidth()
{
return m_width;
}
float Rectangle::GetHeight()
{
return m_height;
}
void Rectangle::printInfo()
{
cout<<"X: "<< GetX()<<",Y: "<< GetY() <<", GetWidth: "<< GetWidth() <<", GetHeight: "<< GetHeight() <<endl;
}
int main()
{
int n;
cin>>n;
for(int i = 0; i < n; i++)
{
Rectangle rectangle(2+i, 3+i, 20+i, 10+i);
rectangle.Move(rectangle.GetX(), rectangle.GetY());
rectangle.printInfo();
}
return 0;
}
运行结果:
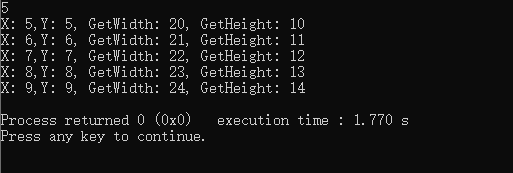
19、第四章-派生类及继承实现之多重继承
问题描述:
1、设计时间类:MyTime
包含数据成员:时、分、秒
包含成员函数:display()
2、设计日期类:MyDate
包含数据成员:年、月、日
包含成员函数:display()
3、设计childName 类派生自:MyTime、MyDate类,
其也有类:display()
要求1:定义childName 对象,实现对象的输出操作。
要求2:初始化借助 调用基类初始化成员列表实现。
输入:
薛之谦 2000 1 1 12 05 05
输出:
MyTime is running!
MyDate is running!
Birthtime is running!
姓名:薛之谦 , 出生日期和时间为:
出生年月:2000年1月1日
出生时间:12时5分5秒
~Birthtime is running!
~MyDate is running!
~MyTime is running!
代码实现:
#include<iostream>
#include <string>
using namespace std;
//时间类
class MyTime
{
public:
MyTime(int, int , int);
void display();
~MyTime();
private:
int m_hour;
int m_minute;
int m_second;
};
MyTime::MyTime(int hour, int minute, int second)
{
cout<<"MyTime is running!"<<endl;
m_hour = hour;
m_minute = minute;
m_second = second;
}
void MyTime::display()
{
//出生时间:12时18分25秒
cout<<"出生时间:"<< m_hour << "时" << m_minute << "分" << m_second << "秒"<<endl;
}
MyTime::~MyTime()
{
cout<<"~MyTime is running!"<<endl;
}
//日期类
class Date{
public:
Date(int, int, int);
void display();
~Date();
private:
int m_year;
int m_mouth;
int m_day;
};
Date::Date(int year, int mouth, int day)
{
cout<<"MyDate is running!"<<endl;
m_year = year;
m_mouth = mouth;
m_day = day;
}
void Date::display()
{
//出生年月:1981年11月15日
cout<<"出生年月:"<< m_year <<"年"<< m_mouth <<"月"<< m_day <<"日"<<endl;
}
Date::~Date()
{
cout<<"~MyDate is running!"<<endl;
}
class ChildName:public MyTime, public Date
{
public:
ChildName(int hour, int minute, int second, int year, int mouth, int day, string);
void display();
~ChildName();
private:
string m_name;
};
ChildName::ChildName(int hour, int minute, int second, int year, int mouth, int day, string name):MyTime(hour, minute, second), Date(year, mouth, day)
{
cout<<"Birthtime is running!"<<endl;
m_name = name;
}
ChildName::~ChildName()
{
cout<<"~Birthtime is running!"<<endl;
}
void ChildName::display()
{
cout<<"姓名:"<<m_name<<", 出生日期和时间为:"<<endl;
Date::display();
MyTime::display();
}
int main()
{
int hour;
int minute;
int second;
int year;
int mouth;
int day;
string name;
//输入姓名 出生日期 出生时间
cin>> name >> year >> mouth >> day >> hour >> minute >> second;
//初始化
ChildName childName(hour, minute, second, year, mouth, day, name);
//输出
childName.display();
return 0;
}
运行结果:
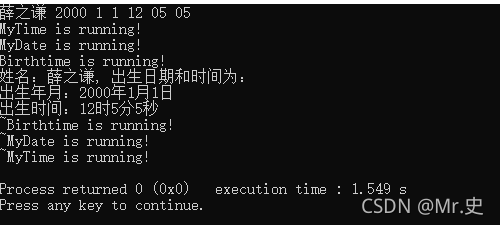
20、第四章-派生类及继承实现之虚函数
问题描述:
基类area_info 有两个派生类:rectangle 矩形类和三角形类 triangle,让每个派生类都包含函数:area()。
分别用来求解矩形的面积和三角形的面积。
要求:1、使用构造函数实现初始化。
2、输入值自己控制。
3、使用基类指针访问虚函数的方式(运行时多态,没有讲到,需要预习)求解矩形面积和三角形面积。
如下代码是开头部分,需要补充完善。
#include <iostream>
using namespace std;
#include <string.h>
class area_info
{
public:
area_info(double r, double s)
{
m_height = r;
m_width = s;
}
virtual double area() = 0;
protected:
double m_height;
double m_width;
};
输入:
11.5 22.3
输出:
正方形的面积为:256.45
三角形的面积为:128.225
代码实现:
#include<iostream>
using namespace std;
//基类area_info 有两个派生类:Rectangle 矩形类和三角形类 triangle,让每个派生类都包含函数:area()。
//分别用来求解矩形的面积和三角形的面积。
//要求:1、使用构造函数实现初始化。
//2、输入值自己控制。
//3、使用基类指针访问虚函数的方式(运行时多态,没有讲到,需要预习)求解矩形面积和三角形面积。
//如下代码是开头部分,需要补充完善
class Area_info
{
public:
Area_info(double height,double width)
{
m_height = height;
m_width = width;
}
virtual double area()=0;
protected:
double m_height;
double m_width;
};
//矩形类
class Rectangle:public Area_info
{
public:
Rectangle(double height,double width):Area_info(height, width)
{
}
double area()
{
return m_height * m_width;
}
};
//三角形类
class Triangle:public Area_info
{
public:
Triangle(double height, double width):Area_info(height, width)
{
}
double area()
{
return m_height*m_width/2;
}
};
int main()
{
double height;
double width;
Area_info *ai;
cin>>height>>width;
Rectangle rectangle(height, width);
Triangle triangle( height, width);
ai = &rectangle;
cout<<"正方形的面积为:"<<rectangle.area()<<endl;
ai = ▵
cout<<"三角形的面积为:"<<triangle.area()<<endl;
}
运行结果:
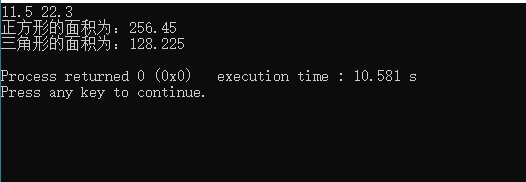
21、实现一维数组的函数重载:
问题描述:
实现一维数组的函数重载:
main 函数定义如下:
int main()
{
int icnt = 0;
cin >> icnt;
g_n = icnt;
int* pLeftArray = new int[icnt];
int* pRightArray = new int[icnt];
for(int i = 0; i < icnt; i++)
{
pLeftArray[i] = i;
pRightArray[i] = i+1;
cout << "pLeftArray[" << i << "] = " << pLeftArray[i];
cout << ", pRightArray[" << i << "] = " << pRightArray[i] <<endl;
}
MyArray bArray(pLeftArray);
MyArray cArray(pRightArray);
MyArray rstArray = bArray+cArray;
rstArray.printArray();
return 0;
}
补全类的定义和重载函数的说明。
输入:
10
输出:
pLeftArray[0] = 0, pRightArray[0] = 1
pLeftArray[1] = 1, pRightArray[1] = 2
pLeftArray[2] = 2, pRightArray[2] = 3
pLeftArray[3] = 3, pRightArray[3] = 4
pLeftArray[4] = 4, pRightArray[4] = 5
pLeftArray[5] = 5, pRightArray[5] = 6
pLeftArray[6] = 6, pRightArray[6] = 7
pLeftArray[7] = 7, pRightArray[7] = 8
pLeftArray[8] = 8, pRightArray[8] = 9
pLeftArray[9] = 9, pRightArray[9] = 10
i = 0, m_iarray[0]= 1
i = 1, m_iarray[1]= 3
i = 2, m_iarray[2]= 5
i = 3, m_iarray[3]= 7
i = 4, m_iarray[4]= 9
i = 5, m_iarray[5]= 11
i = 6, m_iarray[6]= 13
i = 7, m_iarray[7]= 15
i = 8, m_iarray[8]= 17
i = 9, m_iarray[9]= 19
代码实现:
#include <iostream>
using namespace std;
int g_n = 0;
class MyArray{
public:
MyArray(){};
MyArray(int* arry);
void printArray();
int* m_arry = new int[g_n];
};
MyArray::MyArray(int* arry)
{
for(int i = 0; i < g_n; i++)
{
m_arry = arry;
}
}
void MyArray::printArray()
{
for (int i = 0; i < g_n; i++)
{
cout << "i = " << i << ", m_iarray[" << i << "]= " <<m_arry[i]<<endl;
}
}
MyArray operator+(MyArray mR1, MyArray mR2)
{
MyArray temp;
for(int i = 0; i < g_n; i++)
{
temp.m_arry[i] = mR1.m_arry[i] + mR2.m_arry[i];
}
return temp;
}
int main()
{
int icnt = 0;
cin >> icnt;
g_n = icnt;
int* pLeftArray = new int[icnt];
int* pRightArray = new int[icnt];
for(int i = 0; i < icnt; i++)
{
pLeftArray[i] = i;
pRightArray[i] = i+1;
cout << "pLeftArray[" << i << "] = " << pLeftArray[i];
cout << ", pRightArray[" << i << "] = " << pRightArray[i] <<endl;
}
MyArray bArray(pLeftArray);
MyArray cArray(pRightArray);
MyArray rstArray = bArray + cArray;
rstArray.printArray();
return 0;
}
运行结果:
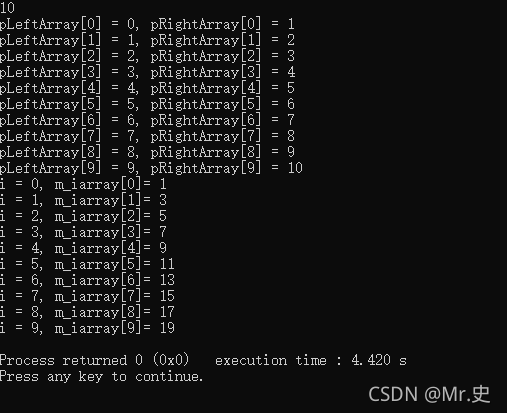
22、友元运算符重载
问题描述:
结合如下主函数,补全类的定义,要求:使用友元运算符重载函数实现复数的加法运算符重载、减法运算符重载。
int main()
{
int real_a = 0;
int imag_a = 0;
int real_b = 0;
int imag_b = 0;
cin >> real_a >> imag_a >> real_b >> imag_b;
MyComplex compx_a(real_a, imag_a);
MyComplex compx_b(real_b, imag_b);
MyComplex compx_sum = compx_a + compx_b;
compx_sum.getComplexRst();
MyComplex compx_sub = compx_a - compx_b;
compx_sub.getComplexRst();
return 0;
}
输入:
11 22 33 44
输出:
rst = 44+66i
rst = -22-22i
代码实现:
#include <iostream>
using namespace std;
class MyComplex
{
public:
MyComplex(){};
MyComplex(int ,int );
void getComplexRst();
int m_real = 0;
int m_imag = 0;
};
MyComplex::MyComplex(int real, int imag)
{
m_real = real;
m_imag = imag;
}
void MyComplex::getComplexRst()
{
if(m_imag > 0)
{
cout<<"rst = "<<m_real << "+" << m_imag <<"i"<<endl;
}
else
{
cout<<"rst = "<<m_real << m_imag <<"i"<<endl;
}
}
MyComplex operator+(MyComplex om1, MyComplex om2)
{
MyComplex temp;
temp.m_real = om1.m_real + om2.m_real;
temp.m_imag = om1.m_imag + om2.m_imag;
return temp;
}
MyComplex operator-(MyComplex om1, MyComplex om2)
{
MyComplex temp;
temp.m_real = om1.m_real - om2.m_real;
temp.m_imag = om1.m_imag - om2.m_imag;
return temp;
}
int main()
{
int real_a = 0;
int imag_a = 0;
int real_b = 0;
int imag_b = 0;
cin >> real_a >> imag_a >> real_b >> imag_b;
MyComplex compx_a(real_a, imag_a);
MyComplex compx_b(real_b, imag_b);
MyComplex compx_sum = compx_a + compx_b;
compx_sum.getComplexRst();
MyComplex compx_sub = compx_a - compx_b;
compx_sub.getComplexRst();
return 0;
}
运行结果:
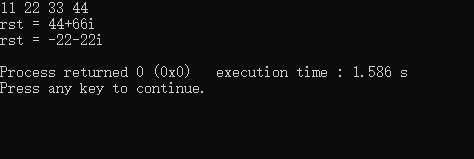
23、虚基类的实现
问题描述:
main()函数已经写好,需要完善类的定义。
基类:base,base的子类:base01(实现变量+10),base02(实现变量+20), Derived 继承自:base01,base02。
参考教材:P172 修改代码。
需要借助:虚基类实现。
int main()
{
int ival = 0;
cin >> ival;
Derived obj(ival);
return 0;
}
输入:
5
输出:
base=5
in base01(), m_a = 15
in base02(), m_a = 35
base01::m_a = 35
base02::m_a = 35
代码实现:
#include<iostream>
using namespace std;
class base
{
public:
base() {}
base(int ival)
{
a = ival;
cout<<"Base="<<a<<endl;
}
int a;
};
class base01:virtual public base
{
public:
base01() {}
base01(int ival):base(ival)
{
a = a+10;
cout<<"in Base01(), m_a = "<<a<<endl;
}
int b1;
};
class base02:virtual public base
{
public:
base02() {}
base02(int ival):base(ival)
{
a = a+20;
cout<<"in Base02(), m_a = "<<a<<endl;
}
~base02()
{
}
int b2;
};
class Derived:public base01,public base02
{
public:
Derived() {}
Derived(int ival):base(ival),base01(ival),base02(ival)
{
cout<<"Base01::m_a = "<<a<<endl;
cout<<"Base02::m_a = "<<a<<endl;
}
int d;
};
int main()
{
int ival = 0;
cin >> ival;
Derived obj(ival);
return 0;
}
运行结果:
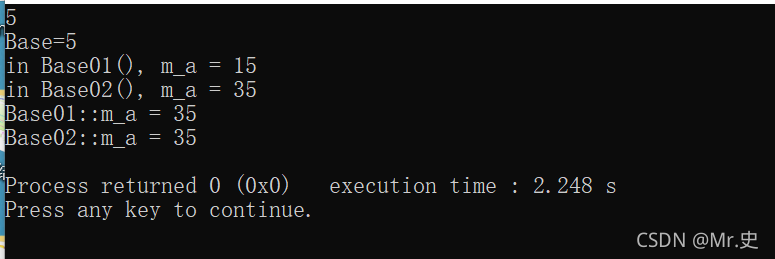
24、纯虚函数的应用
问题描述:
Shape是一个父类,他的子类有:
三角形类:Triangle
矩形类:Rectangle
圆形类:Circle
要求如下:
第一:Shape 类中定义纯虚函数 show 和 area;
第二:area 用途:求解图形面积。
show 用途:打印信息。
第三:要求根据输出完善 核心代码实现,要求实现运行时多态。
第四:main() 函数已经给出,需要写出剩余部分的实现。
int main()
{
Shape *s;
Circle mycircle(20);
Rectangle myrect(3,4);
Triangle mytriangle(6,8,10);
s=&mytriangle;//动态多态
s->show();
cout<<"Triangle:"<<s->area()<<endl;
s=&myrect;//动态多态
s->show();
cout<<"Rectangle Area:"<<s->area()<<endl;
mycircle.show();//静态多态
cout<<"Circle Area:"<<mycircle.area()<<endl;
return 0;
}
输入:
无
输出:
a=6, b=8, c=10
Triangle:24
length=3, width=4
Rectangle Area:12
radius=20
Circle Area:1256.64
代码实现:
#include <iostream>
#include <cmath>
using namespace std;
class Shape
{
public:
Shape()
{
}
Shape(double radius)
{
m_radius = radius;
}
Shape(double length, double width)
{
m_width = width;
m_length = length;
}
Shape(double a, double b, double c)
{
m_a = a;
m_b = b;
m_c = c;
}
virtual double area() = 0;
virtual void show() = 0;
protected:
double m_length;
double m_width;
double m_a;
double m_b;
double m_c;
double m_radius;
double m_result;
};
//圆形
class Circle:public Shape
{
public:
Circle(double radius):Shape(radius)
{
}
double area();
void show();
};
double Circle::area()
{
return 3.14159 * m_radius * m_radius;
}
void Circle::show()
{
cout<<"radius="<<m_radius<<endl;
}
//矩形
class Rectangle:public Shape
{
public:
Rectangle(double length, double width):Shape(length, width)
{
}
virtual double area();
virtual void show();
};
double Rectangle::area()
{
return m_length * m_width;
}
void Rectangle::show()
{
cout<<"length="<< m_length <<", width="<< m_width <<endl;
}
//三角形
class Triangle:public Shape
{
public:
Triangle(double a, double b, double c):Shape(a, b, c)
{
}
double area();
void show();
};
double Triangle::area()
{
return (m_a * m_b)/2;
}
void Triangle::show()
{
cout<<"a="<< m_a <<", b="<< m_b <<", c="<< m_c <<endl;
}
int main()
{
Shape *s;
Circle mycircle(20);
Rectangle myrect(3,4);
Triangle mytriangle(6,8,10);
s=&mytriangle;//动态多态
s->show();
cout<<"Triangle:"<<s->area()<<endl;
s=&myrect;//动态多态
s->show();
cout<<"Rectangle Area:"<<s->area()<<endl;
mycircle.show();//静态多态
cout<<"Circle Area:"<<mycircle.area()<<endl;
return 0;
}
运行结果:
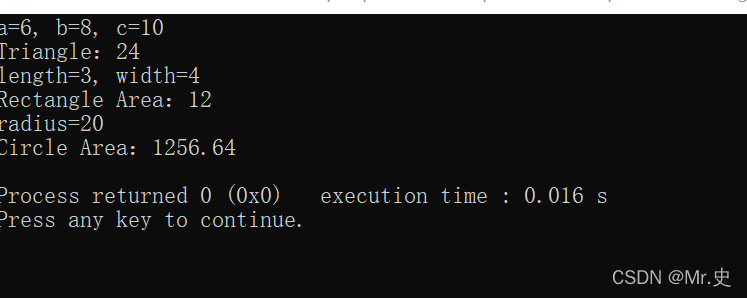
25、函数模块使用
问题描述:
编写一个对具有n个元素的数组array[ ]求最小值的程序,要求将求最小值的函数设计成函数模板。
main()函数参考如下:
int main()
{
int iArray[MAX_SIZE]= {0};
double dArray[MAX_SIZE] = {0.0};
int icnt = 0;
cin >> icnt;
for(int i = 0; i < icnt; i++)
{
cin >> iArray[i];
}
for(int j = 0; j < icnt; j++)
{
cin >> dArray[j];
}
cout<<"a数组的最小值为:"
<<GetMinVal(iArray,icnt)<< endl;
cout<<"b数组的最小值为:"
<<GetMinVal(dArray,icnt)<<endl;
return 0;
}
输入:
5
1 3 4 5 0
1.2 3.5 4.8 9.2 0.3
输出:
a数组的最小值为:0
b数组的最小值为:0.3
代码实现:
#include <iostream>
#include <string>
using namespace std;
const int MAX_SIZE = 10;
template<typename T>
T GetMinVal(T* arry, int arrySize)
{
int i = 0;
T minSum = arry[i];
for(i = 0; i < arrySize; i++)
{
if(arry[i] < minSum)
{
minSum = arry[i];
}
}
return minSum;
}
int main()
{
int iArray[MAX_SIZE]= {0};
double dArray[MAX_SIZE] = {0.0};
int icnt = 0;
cin >> icnt;
for(int i = 0; i < icnt; i++)
{
cin >> iArray[i];
}
for(int j = 0; j < icnt; j++)
{
cin >> dArray[j];
}
cout<<"a数组的最小值为:"
<<GetMinVal(iArray,icnt)<< endl;
cout<<"b数组的最小值为:"
<<GetMinVal(dArray,icnt)<<endl;
return 0;
}
运行结果:
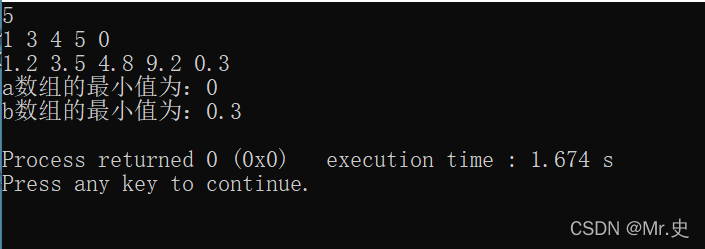
26、综合题
问题描述:
1、定义一个Person 类,
包含成员函数:show() 用于输出基础信息
成员变量:name,sex,age
其中age 整形,其他string类型。
2、定义一个Student类继承自Person 类,
新增了id,date,score 三个变量
其中score int 类型,其他string 类型。
输入:
第一行:人数 N
第二行:待查找的成绩。
第三行之后:N个学生的基础信息。
输出:
见下面。
输入:
3
480
Mary 男 19 20170101001 2017.09.01 580
Hunk 男 18 20210101002 2021.09.01 390
Lucy 女 18 20210101003 2021.09.01 630
输出:
第 1个同学的信息为:
student’s id: 20170101001
name: Mary
sex: 男
age: 19
enrollment date: 2017.09.01
enrollment score: 580
第 2个同学的信息为:
student’s id: 20210101002
name: Hunk
sex: 男
age: 18
enrollment date: 2021.09.01
enrollment score: 390
第 3个同学的信息为:
student’s id: 20210101003
name: Lucy
sex: 女
age: 18
enrollment date: 2021.09.01
enrollment score: 630
分数为:480 不存在
全班最高成绩为:630
成绩最高同学的姓名为:Lucy
代码实现:
#include <iostream>
#include <string>
using namespace std;
class Person
{
public:
Person()
{
}
Person(string, string, int );
virtual void show() = 0;
protected:
string m_name;
string m_sex;
int m_age;
};
Person::Person(string name, string sex, int age)
{
m_name = name;
m_sex = sex;
m_age = age;
}
class Student:public Person
{
public:
Student()
{
}
Student(string name, string sex, int age, string id, string date, int score);
void show();
int getScore()
{
return m_score;
}
string getName()
{
return m_name;
}
private:
string m_id;
string m_date;
int m_score;
};
Student::Student(string name, string sex, int age, string id, string date, int score):Person(name, sex, age)
{
m_id = id;
m_date = date;
m_score = score;
}
void Student::show()
{
cout<<" student's id: "<< m_id <<endl;
cout<<" name: "<< m_name <<endl;
cout<<" sex: "<< m_sex <<endl;
cout<<" age: "<< m_age <<endl;
cout<<" enrollment date: "<< m_date <<endl;
cout<<" enrollment score: "<< m_score <<endl;
}
int main()
{
string name;
string sex;
int age;
string id;
string date;
int score;
//学生人数
int n;
//待查询学生成绩
int search_score;
int flag = -1;
string flag_name;
int top_score = 0;
string top_name;
cin>>n>>search_score;
Student student[n];
for(int i = 0; i < n; i++)
{
cin>> name >> sex >> age >> id >> date >>score;
student[i] = {name, sex, age, id, date, score};
}
for(int i = 0; i < n; i++)
{
cout<<"第 "<< i+1 <<"个同学的信息为:"<<endl;
student[i].show();
//查找分数
if(student[i].getScore() == search_score)
{
flag = 0;
flag_name = student[i].getName();
}
//获取最高分
if(student[i].getScore() > top_score)
{
top_score = student[i].getScore();
top_name = student[i].getName();
}
if(i == n -1)
{
flag == 0 ? cout<<"分数为:"<< search_score <<" 存在!该学生姓名为:"<< flag_name <<endl : cout<<"分数为:"<< search_score <<" 不存在"<<endl;
cout<<"全班最高成绩为:"<< top_score <<endl;
cout<<"成绩最高同学的姓名为:"<< top_name <<endl;
}
}
return 0;
}
运行结果:
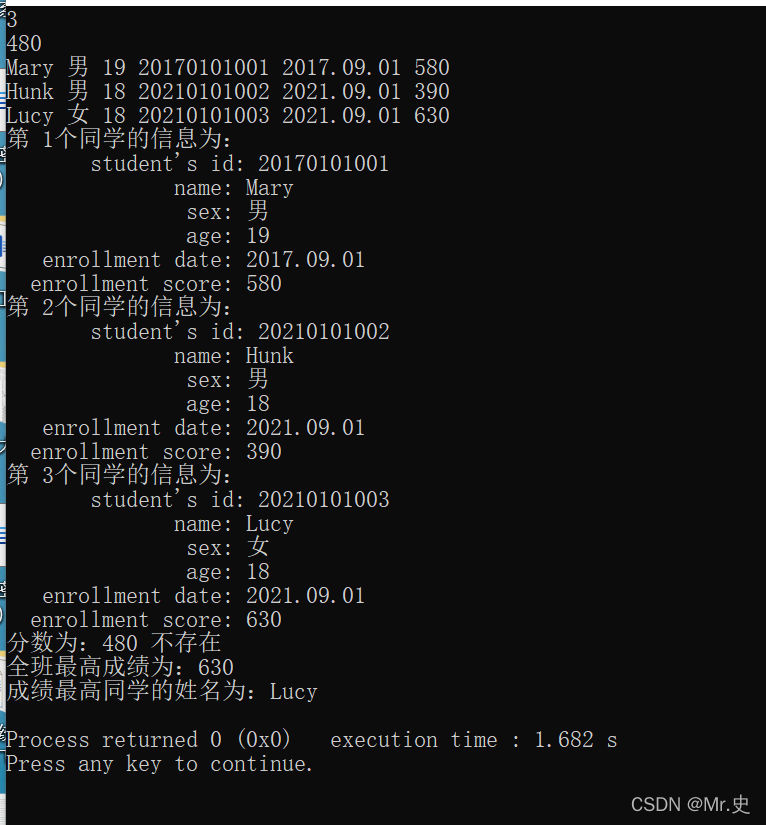
27、类模板实现
问题描述:
1、定义一个类模板,实现输出最大值、最小值。
输入:两个 int 类型值,或者两个 float,或者两个double类型值,类型自己定义。
输出:两者中的最大值,两者中的最小值。
输入:
33 55
33.33 55.55
33.3333 55.5555
输出:
Min is:33
Max is:55
Min is:33.33
Max is:55.55
Min is:33.3333
Max is:55.5555
代码实现:
#include <iostream>
using namespace std;
template<typename T>
class Compare
{
public:
Compare(T a, T b)
{
m_x = a;
m_y = b;
}
T Max()
{
return (m_x > m_y)? m_x : m_y;
}
T Min()
{
return (m_x < m_y)? m_x : m_y;
}
private:
T m_x;
T m_y;
};
int main()
{
int a, b;
double x, y;
double c, d;
cin>>a>>b;
cin>>x>>y;
cin>>c>>d;
Compare <int>compare(a, b);
cout<<"Min is:"<<compare.Min()<<endl;
cout<<"Max is:"<<compare.Max()<<endl;
Compare <double>compare1(x, y);
cout<<"Min is:"<<compare1.Min()<<endl;
cout<<"Max is:"<<compare1.Max()<<endl;
Compare <double>compare2(c, d);
cout<<"Min is:"<<compare2.Min()<<endl;
cout<<"Max is:"<<compare2.Max()<<endl;
return 0;
}
运行结果:
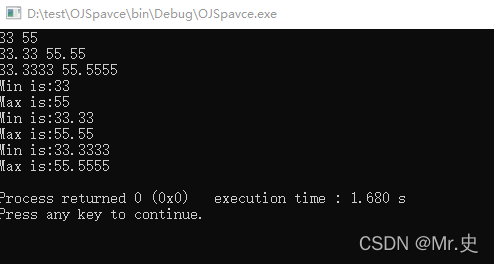
28、函数模板
问题描述:
用函数模板实现数据的从小到大排序。
要求:
1、使用刚讲过的 直接插入排序方法。
2、用模板实现,模板支持不同的类型。
主函数已经给出:
int main()
{
int iArray[MAX_SIZE] = {0};
float fArray[MAX_SIZE] = {0.0f};
initArray(iArray, MAX_SIZE);
initArray(fArray, MAX_SIZE);
insertSort(iArray, MAX_SIZE);
printArray(iArray, MAX_SIZE);
insertSort(fArray, MAX_SIZE);
printArray(fArray, MAX_SIZE);
return 0;
}
输入:
10 9 8 7 6 5 4 3 2 100
10.1 9.8 8.7 7.6 6.5 5.4 3.2 2.1 100.88 1.6
输出:
------------sort begin----------------
pArray[0] = 2
pArray[1] = 3
pArray[2] = 4
pArray[3] = 5
pArray[4] = 6
pArray[5] = 7
pArray[6] = 8
pArray[7] = 9
pArray[8] = 10
pArray[9] = 100
------------sort end-----------------
------------sort begin----------------
pArray[0] = 1.6
pArray[1] = 2.1
pArray[2] = 3.2
pArray[3] = 5.4
pArray[4] = 6.5
pArray[5] = 7.6
pArray[6] = 8.7
pArray[7] = 9.8
pArray[8] = 10.1
pArray[9] = 100.88
------------sort end-----------------
代码实现:
#include <iostream>
using namespace std;
const int MAX_SIZE = 10;
template<typename T>
void initArray(T* arry, int sizes)
{
for(int i = 0; i < sizes; i++)
{
cin>>arry[i];
}
}
template<typename T>
void insertSort(T* arry, int sizes)
{
int i, j;
T temp;
for(i = 1; i < sizes; i++)
{
if(arry[i] < arry[i-1])
{
temp = arry[i];
for(j = i; j > 0 ; j-- )
{
if(arry[j - 1] > temp)
{
arry[j] = arry[j - 1];
}
else
{
break;
}
}
arry[j] = temp;
}
}
}
template<typename T>
void printArray(T* arry, int sizes)
{
cout<<"------------sort begin----------------"<<endl;
for(int i = 0; i < sizes; i++)
{
cout<<"pArray["<<i<<"] = "<<arry[i]<<endl;
}
cout<<"------------sort end-----------------"<<endl;
}
int main()
{
int iArray[MAX_SIZE] = {0};
float fArray[MAX_SIZE] = {0.0f};
initArray(iArray, MAX_SIZE);
initArray(fArray, MAX_SIZE);
insertSort(iArray, MAX_SIZE);
printArray(iArray, MAX_SIZE);
insertSort(fArray, MAX_SIZE);
printArray(fArray, MAX_SIZE);
return 0;
}
运行结果:
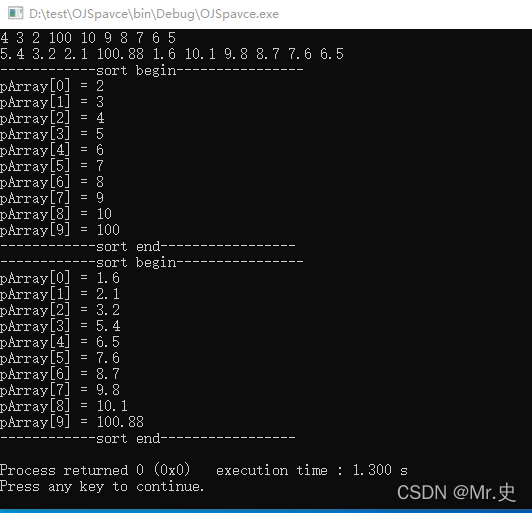
29、异常处理实现
问题描述:
根据main 函数和输出结果,写出缺失函数实现。
缺失函数功能为:加薪,若为正常值,则加薪15%。否则若为-1,则抛出异常。
int main()
{
int icnt = 0;
cin >> icnt;
float* pOldArray = new float[icnt];
for(int i = 0; i < icnt; i++)
{
cin >> pOldArray[i];
}
for(int i = 0; i < icnt; i++)
{
try
{
float newSalary = addSalary(pOldArray[i]);
cout << "newSalary = " << newSalary << endl;
}
catch(string s)
{
cout << s << endl;
}
cout << "i = " << i << " finished..." << endl << endl;
}
delete []pOldArray;
return 0;
}
输入:
3
1000.5 2000.5 -1
输出:
addSalary begin …
addSalary end…
newSalary = 1149
i = 0 finished…
addSalary begin …
addSalary end…
newSalary = 2299
i = 1 finished…
addSalary begin …
addSalary error : zero salary
zero salary
i = 2 finished…
代码实现:
#include <iostream>
#include <string>
using namespace std;
float addSalary(float salary)
{
cout<<"addSalary begin ..."<<endl;
if(salary == -1)
{
string s = "addSalary error : zero salary\nzero salary";
throw s;
}
cout<<"addSalary end..."<<endl;
return salary + salary*0.15;
}
int main()
{
int icnt = 0;
cin >> icnt;
float* pOldArray = new float[icnt];
for(int i = 0; i < icnt; i++)
{
cin >> pOldArray[i];
}
for(int i = 0; i < icnt; i++)
{
try
{
float newSalary = addSalary(pOldArray[i]);
cout << "newSalary = " << newSalary << endl;
}
catch(string s)
{
cout << s << endl;
}
cout << "i = " << i << " finished..." << endl << endl;
}
delete []pOldArray;
return 0;
}
运行结果:
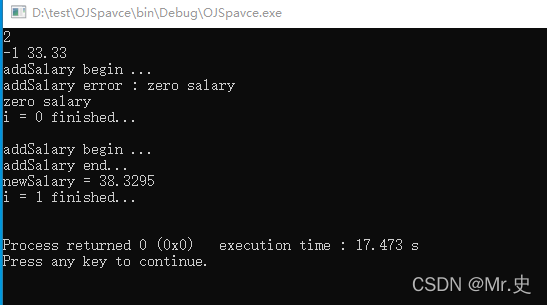
30、
问题描述:
输入:
输出:
代码实现:
运行结果: