一、问题描述
线上一个查询服务,偶尔会报一次查询出来的结果集合包含null。
二、问题排查
在多线程查询过程中,使用了ArrayList,多线程查询出来后执行ArrayList.add()。然而ArrayList并不是线程安全的集合,会导致null值出现。可以考虑使用SynchronizedList。
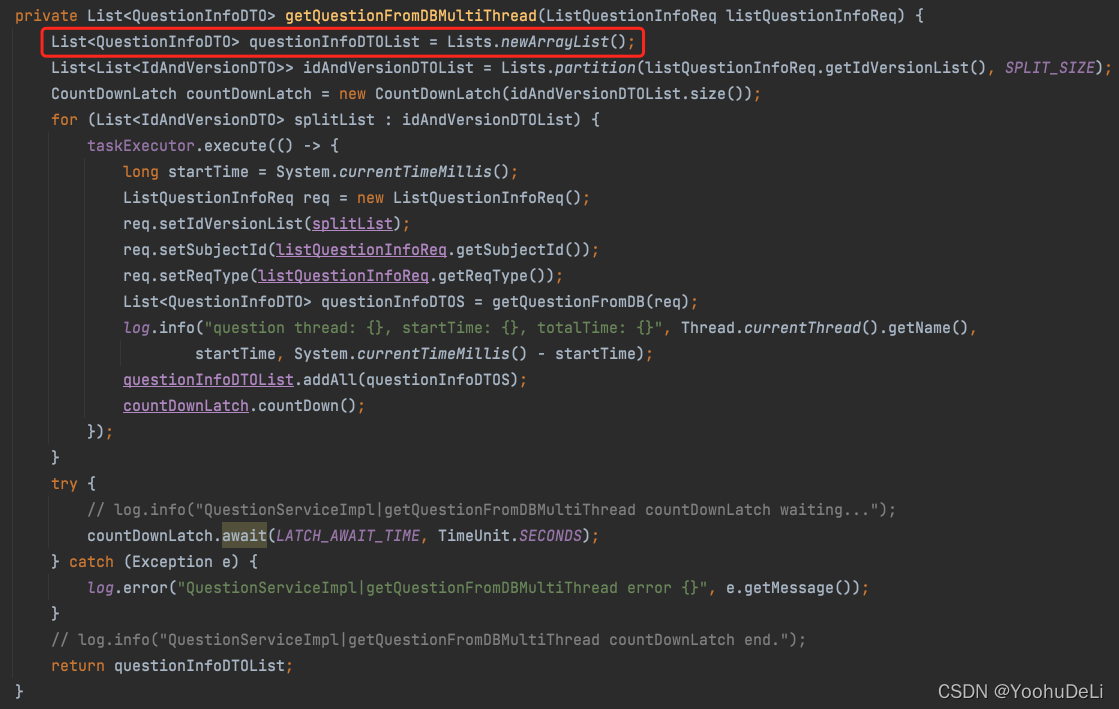
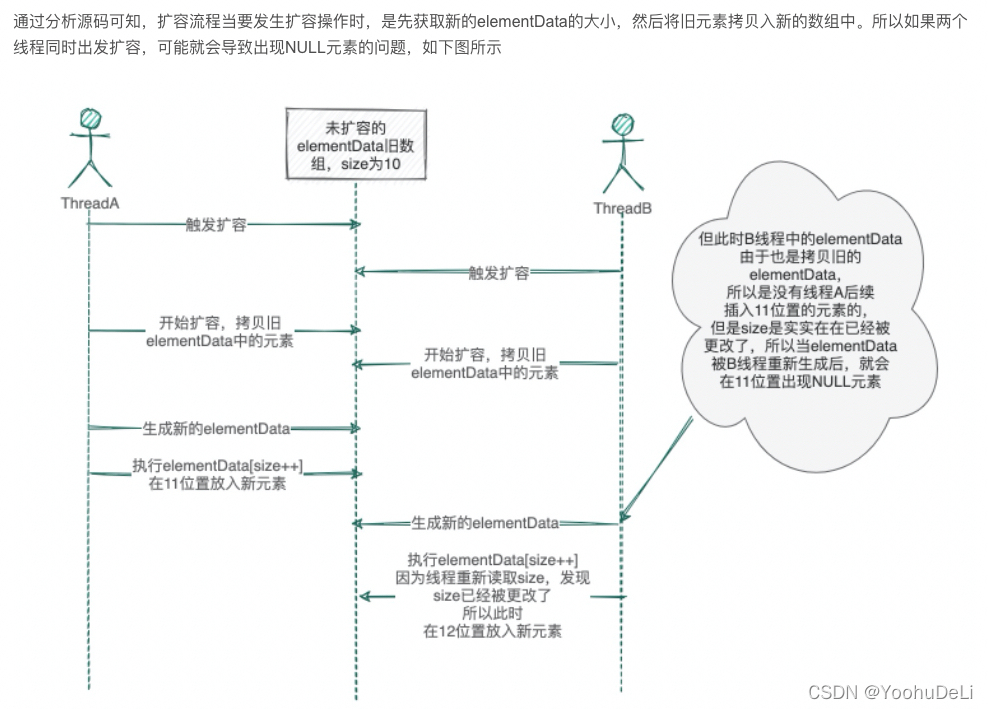
三、问题复现
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit;
public class TestSync {
private static final Integer n = 100;
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
arrayList();
System.out.println("arrayList时间:" + (System.currentTimeMillis() - startTime));
startTime = System.currentTimeMillis();
synchronizedList();
System.out.println("synchronizedList时间:" + (System.currentTimeMillis() - startTime));
startTime = System.currentTimeMillis();
copyArrayList();
System.out.println("copyArrayList时间:" + (System.currentTimeMillis() - startTime));
startTime = System.currentTimeMillis();
futureList();
System.out.println("futureList时间:" + (System.currentTimeMillis() - startTime));
}
private static void arrayList() {
List<Integer> testList = new ArrayList<>();
CountDownLatch countDownLatch = new CountDownLatch(n);
for (int i=0; i<n; i++) {
new Thread(() -> {
testList.add(1);
countDownLatch.countDown();
}).start();
}
try {
countDownLatch.await(10, TimeUnit.SECONDS);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println(testList);
}
private static void synchronizedList() {
List<Integer> testList = Collections.synchronizedList(new ArrayList<>());
CountDownLatch countDownLatch = new CountDownLatch(n);
for (int i=0; i<n; i++) {
new Thread(() -> {
testList.add(1);
countDownLatch.countDown();
}).start();
}
try {
countDownLatch.await(10, TimeUnit.SECONDS);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println(testList);
}
private static void copyArrayList() {
CopyOnWriteArrayList<Integer> testList = new CopyOnWriteArrayList<>();
CountDownLatch countDownLatch = new CountDownLatch(n);
for (int i=0; i<n; i++) {
new Thread(() -> {
testList.add(1);
countDownLatch.countDown();
}).start();
}
try {
countDownLatch.await(10, TimeUnit.SECONDS);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println(testList);
}
private static void futureList() {
List<CompletableFuture<Integer>> futureList = new ArrayList<>();
for (int i=0; i<n; i++) {
CompletableFuture<Integer> task = CompletableFuture.supplyAsync(() -> 1);
futureList.add(task);
}
CompletableFuture.allOf(futureList.toArray(new CompletableFuture[0])).join();
List<Integer> testList = new ArrayList<>();
futureList.forEach(data -> data.whenComplete((integer, throwable) -> {
if (Objects.nonNull(throwable)) {
return;
}
testList.add(integer);
}));
System.out.println(testList);
}
}
执行结果:
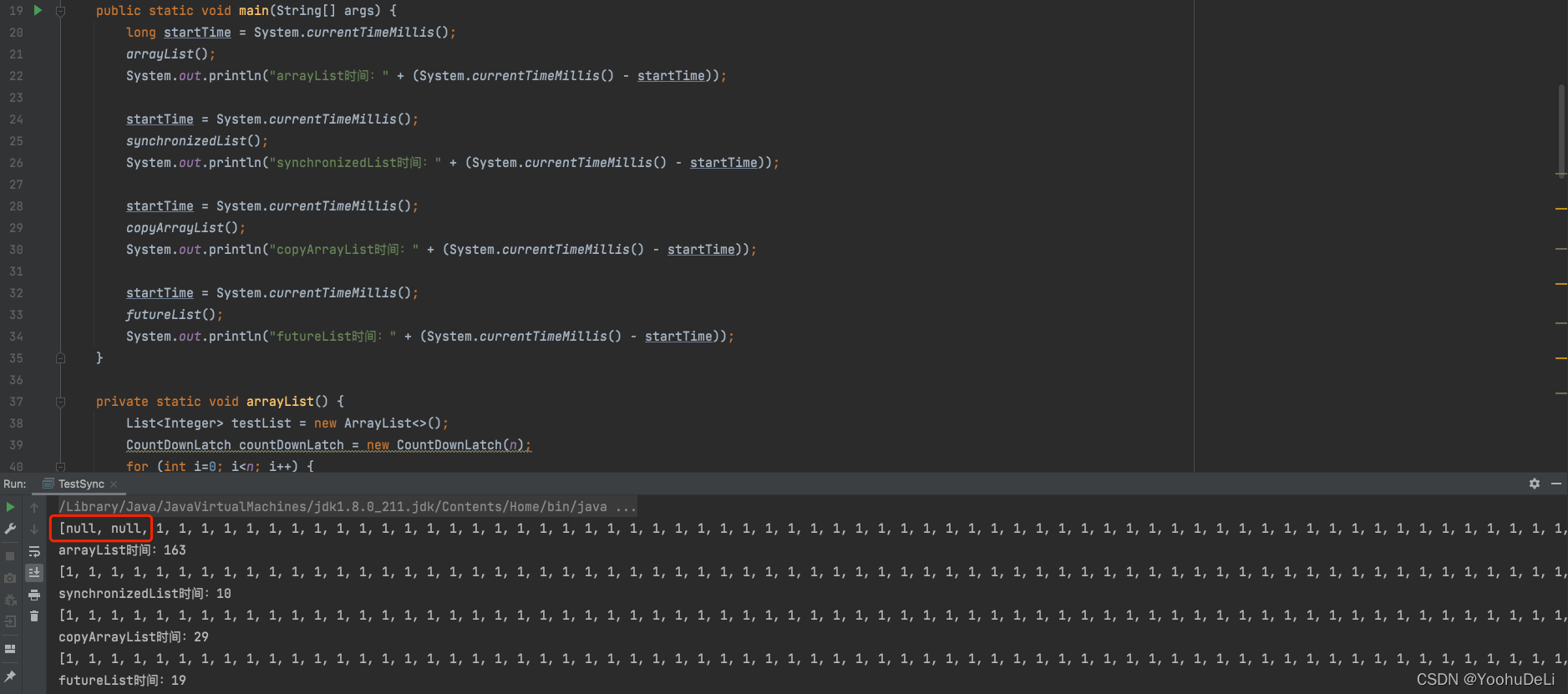