1.之前用的maven管理项目,现在公司用gradle,所以自己学习创建一个,特此记录一下(前提是你得配置了gradle)
1.首先是build.gradle文件
buildscript {
ext {
springBootVersion = '2.1.2.RELEASE'
}
repositories {
maven {
url 'http://maven.aliyun.com/nexus/content/groups/public/'
}
mavenCentral()
mavenLocal()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'org.springframework.boot'
apply plugin: 'io.spring.dependency-management'
group = 'com.inspur'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
maven {
url 'http://maven.aliyun.com/nexus/content/groups/public/'
}
maven {
url = 'http://repo.inspur.com/artifactory/ecbu-gradle-release-local'
credentials {
username 'bss'
password 'USTdYtIj'
}
}
mavenCentral()
mavenLocal()
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.mybatis.spring.boot:mybatis-spring-boot-starter:1.3.2')
compile('org.projectlombok:lombok:1.18.2')
runtime('mysql:mysql-connector-java')
compile('mysql:mysql-connector-java')
testCompile('org.springframework.boot:spring-boot-starter-test')
compile('com.alibaba:fastjson:1.2.5')
compile('com.github.pagehelper:pagehelper:5.1.2')
implementation 'org.springframework.boot:spring-boot-starter-freemarker'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.mybatis.spring.boot:mybatis-spring-boot-starter:1.3.2'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
2. 创建数据库表
CREATE TABLE `student` (
`id` int(11) NOT NULL,
`name` varchar(255) DEFAULT NULL,
`age` int(11) DEFAULT NULL,
`score` varchar(255) DEFAULT NULL,
`class1` varchar(255) DEFAULT NULL,
`school` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of student
-- ----------------------------
INSERT INTO `student` VALUES ('1', '熊大', '20', '100', '动物系', '北京大学');
INSERT INTO `student` VALUES ('2', '熊二', '21', '90', '动物系', '南京大学');
INSERT INTO `student` VALUES ('3', '光头强', '18', '100', '伐木系', '复旦大学');
3.创建项目结构
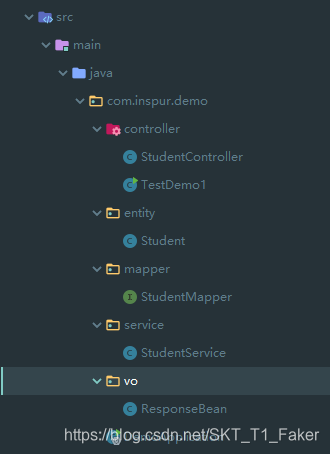
Controller
package com.inspur.demo.controller;
import com.inspur.demo.entity.Student;
import com.inspur.demo.service.StudentService;
import com.inspur.demo.vo.ResponseBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping(value = "/all")
public List<Student> getall(){
return studentService.findAll();
}
}
Service
package com.inspur.demo.service;
import com.inspur.demo.entity.Student;
import com.inspur.demo.mapper.StudentMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class StudentService {
@Autowired
private StudentMapper studentMapper;
public List<Student> findAll() {
return studentMapper.queryAll();
}
}
Mapper
package com.inspur.demo.mapper;
import com.inspur.demo.entity.Student;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface StudentMapper {
List<Student> queryAll();
}
Entity
package com.inspur.demo.entity;
import lombok.Data;
@Data
public class Student {
private Integer id;
private String name;
private Integer age;
private Integer score;
private String class1;
private String school;
public Student(){}
public Student(Integer id,String name,Integer age,Integer score,String class1,String school){
this.id=id;
this.name=name;
this.age=age;
this.score=score;
this.class1=class1;
this.school=school;
}
}
4.resources目录下添加mybatis的配置文件(文件名是 mybatis-config.xml)
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<setting name="callSettersOnNulls" value="true"/>
<setting name="logImpl" value="STDOUT_LOGGING"/>
<setting name="cacheEnabled" value="false"/>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
</configuration>
日志配置文件( 文件名是 logback-spring.xml)
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<include resource="org/springframework/boot/logging/logback/base.xml" />
<appender name="LOG_FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<Prudent>true</Prudent>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>log/logFile.%d{yyyy-MM-dd}.log</fileNamePattern>
<maxHistory>30</maxHistory>
<totalSizeCap>1GB</totalSizeCap>
</rollingPolicy>
<layout class="ch.qos.logback.classic.PatternLayout">
<Pattern> %d{yyyy-MM-dd HH:mm:ss} -%msg%n</Pattern>
</layout>
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss.SSS} %-5level --- [%15thread{15}] %-40logger{40} : %msg%n</pattern>
</encoder>
</appender>
<logger name="org" level="INFO"/>
<logger name="sun" level="INFO"/>
<logger name="com.inspur" level="INFO" />
<root level="INFO">
<appender-ref ref="LOG_FILE" />
<!--<appender-ref ref="FILE" />-->
</root>
</configuration>
5.添加mapper.xml文件(名字是 StudentMapper.xml)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.inspur.demo.mapper.StudentMapper">
<select id="queryAll" resultType="com.inspur.demo.entity.Student">
select * from student
</select>
</mapper>
6.添加application.yml文件
server:
port: 8081
spring:
application:
name: demo
datasource:
url: jdbc:mysql://localhost:3306/test?characterEncoding=utf8&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: root
jackson:
date-format: yyyy-MM-dd HH:mm:ss
time-zone: GMT+8
mybatis:
mapper-locations: classpath:mapper/**/*.xml
config-location: classpath:mybatis-config.xml
6.项目启动类
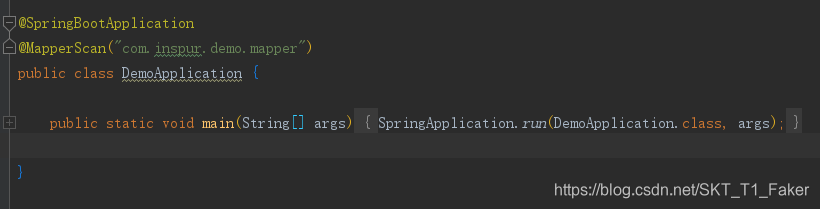
7.启动测试
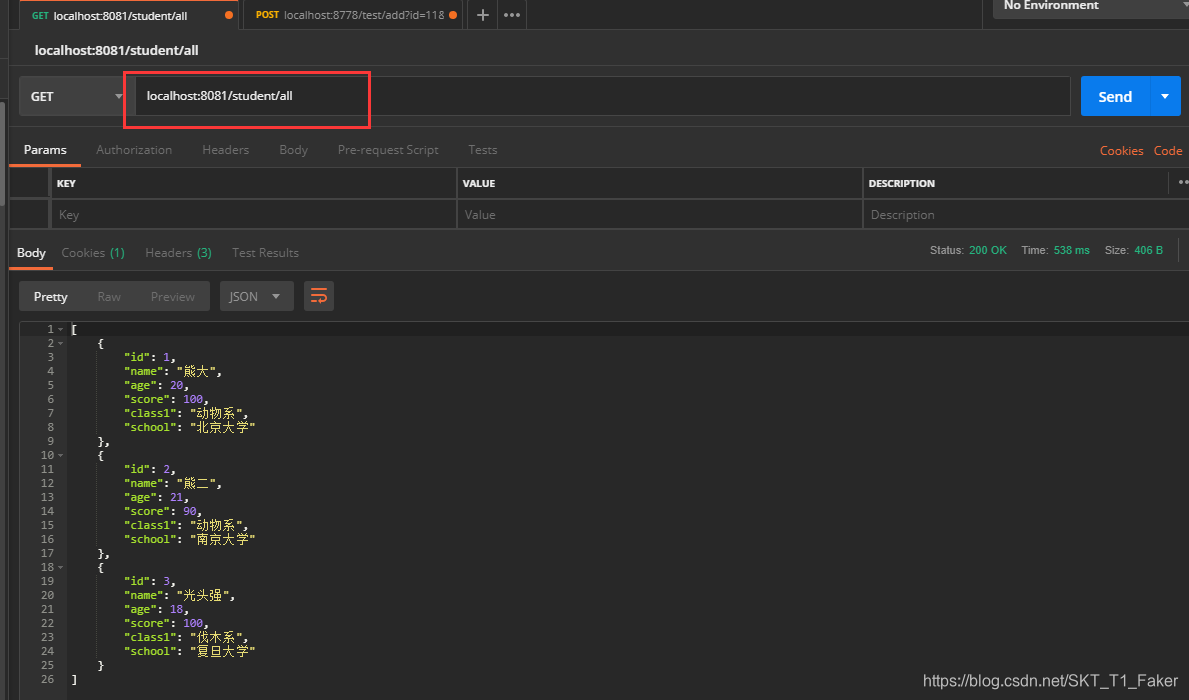
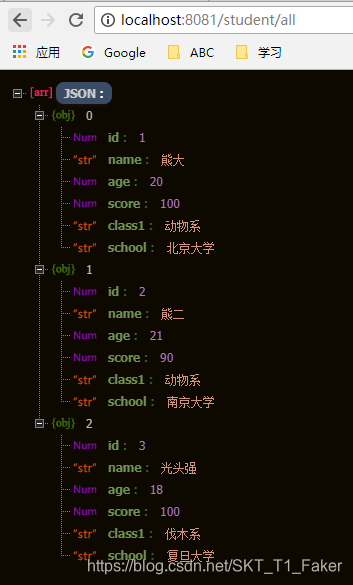
8.大功告成