当我们在实现数据结构vector时,我们发现使用mencpy时只能实现基本类型的拷贝,而不能实现自定义类型的拷贝,比如说字符串类型。这问题如何解决呢?在学习了模板和基于模板的类型萃取之后,我们就有方法是在实现基本类型的拷贝时使用memcpy,在遇到自定义类型时用for循环来拷贝。
#pragma once
#include <typeinfo.h>
#include <iostream>
#include <string>
using namespace std;
struct _TrueType
{};
struct _FalseType
{};
template <class tp>
class _TypeTraits
{
public:
typedef _FalseType __IsPODType;
};
template <>
class _TypeTraits<bool>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<int>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<char>
{
public:
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<float>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<double>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<unsigned char>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<short>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<unsigned short>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<unsigned int>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<unsigned long>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<long>
{
typedef _TrueType __IsPODType;
};
template <>
class _TypeTraits<long double>
{
typedef _TrueType __IsPODType;
};
template <class _tp>
class _TypeTraits<_tp*>
{
typedef _TrueType __IsPODType;
};
template <class T>
void Copy(T* dest, T* src, size_t size, _FalseType)
{
cout << "__FalseType:" << typeid(T).name() << endl;
size_t i = 0;
for (; i < size; i++)
{
dest[i] = src[i];
}
}
#include <memory.h>
template <class T>
void Copy(T* dest, T* src, size_t size, _TrueType)
{
cout << "__TrueType:" << typeid(T).name() << endl;
memcpy(dest, src, size);
}
#include "typetraits.h"
#include <stdlib.h>
void test()
{
char a[] = { 1, 2, 3, 4 };
char b[10];
Copy(b, a, 4, _TypeTraits<char>::__IsPODType());
string c[] = { "111", "222", "333", "444" };
string d[10];
Copy(d, c, 4, _TypeTraits<string>::__IsPODType());
}
int main()
{
test();
system("pause");
return 0;
}
运行结果为:

这样的代码是如何实现的呢?我们通过调用过程来看一看:
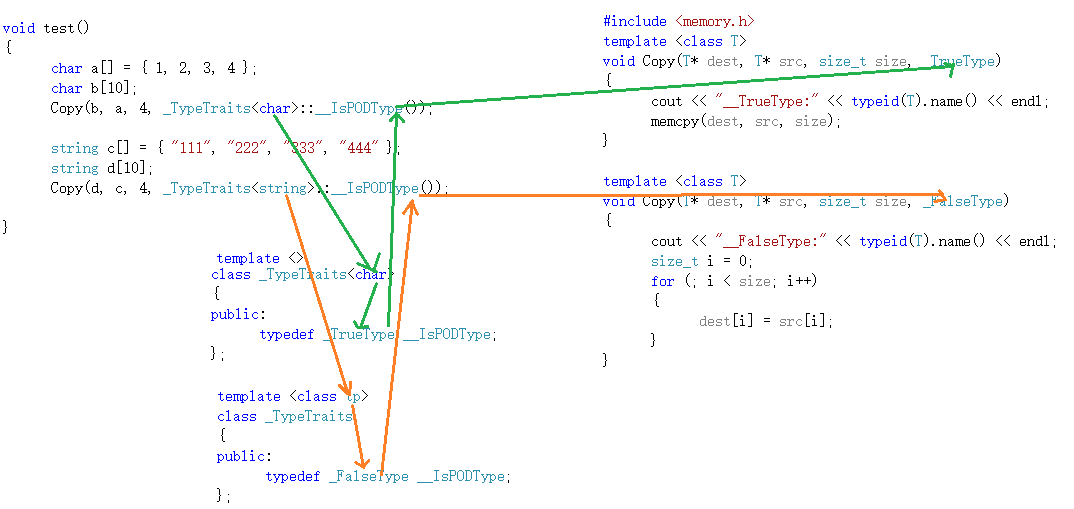
上面的这张图可以一目了然的屡清楚整个过程。