getopt_long 函数的使用网上已经有很多了,这里只是记录一下方便自己后续查找。首先函数原型声明:
#include <getopt.h>
int getopt_long(int argc, char *argv[],
const char *optstring,
const struct option *longopts, int *longindex);
函数是用于解析命令行参数的,与函数 getopt() 函数是相似的,它可以处理长选项,即两个短杠"--" 连接的参数选项。而且比 getopt() 多两个参数。
参数说明:
1,argc 一般就是 main 函数里的 argc
2,argv 同上
3,optstring 要匹配的参数,如:"ha" 或 "h:a" 或 "h:a::",带一个冒号的表示此选项须带参数,两个冒号的表示是可选参数
4,longopts 这个是长选项结构的指针,一般传的是数组
5,longindex 如果不为空,它将指向 longopts 的一个元素的位置,即longopts的数组下标
结构体类型 struct option 声明为:
struct option {
const char *name; /*这个就是长选项的名称*/
int has_arg; /*指示是否带参数*/
int *flag;
int val; /*如果flag=NULL, 匹配到时返回这个值*/
};
name is the name of the long option.
has_arg
is: no_argument (or 0) if the option does not take an
argument; required_argument (or 1) if the option requires
an argument; or optional_argument (or 2) if the option
takes an optional argument.
flag specifies how results are returned for a long option. If
flag is NULL, then getopt_long() returns val. (For
example, the calling program may set val to the equivalent
short option character.) Otherwise, getopt_long() returns
0, and flag points to a variable which is set to val if
the option is found, but left unchanged if the option is
not found.
val is the value to return, or to load into the variable
pointed to by flag.
直接用 man 手册上的例子说明:
#include <getopt.h>
#include <stdio.h> /* for printf */
#include <stdlib.h> /* for exit */
int main(int argc, char *argv[])
{
int c;
int digit_optind = 0;
while (1) {
int this_option_optind = optind ? optind : 1;
int option_index = 0;
/*长选项的匹配只要匹配到前n个字符相同就会返回,若是模棱两可时会返回?,同时stderr会有相应打印*/
static struct option long_options[] = {
{"add", required_argument, 0, 0 },
{"append", no_argument, 0, 0 },
{"delete", required_argument, 0, 0 },
{"verbose", no_argument, 0, 0 },
{"create", required_argument, 0, 'c'},
{"file", required_argument, 0, 0 },
{0, 0, 0, 0 }
};
/*短选项跟一个冒号表示有选项参数,两个冒号表示选项参数可选*/
c = getopt_long(argc, argv, "abc:d:012",
long_options, &option_index);
if (c == -1)
break;
switch (c) {
case 0: /* 匹配到长选项时,返回的val,即struct optaion 中的 val,这里为 0 则表示匹配到 add、append, delete, verbose, file时都会跟到这个 case, 而create 则匹配到字符'c'*/
printf("get option_index = %d, option %s", option_index, long_options[option_index].name);
if (optarg)
printf(" with arg %s", optarg);
printf("\n");
break;
case '0':
case '1':
case '2':
if (digit_optind != 0 && digit_optind != this_option_optind)
printf("digits occur in two different argv-elements.\n");
digit_optind = this_option_optind;
printf("option %c\n", c);
break;
case 'a':
printf("option a\n");
break;
case 'b':
printf("option b\n");
break;
case 'c':
printf("option c with value '%s'\n", optarg);
break;
case 'd':
printf("option d with value '%s'\n", optarg);
break;
case '?': /*ambiguous 模棱两可的时候返回? 如只输入 --a 则会匹配到 add 和 append*/
printf("it's ambiguous, please selete one\n");
break;
default:
printf("?? getopt returned character code 0%o ??\n", c);
}
}
if (optind < argc) {
printf("non-option ARGV-elements: ");
while (optind < argc)
printf("%s ", argv[optind++]);
printf("\n");
}
exit(EXIT_SUCCESS);
}
getopt_long() 函数支持短选项和长选项,如:
可以看到短选项时,如果需要带参数, 参数可以直接紧跟选项或是位于空格之后 。而长选项则需要加"=",如:
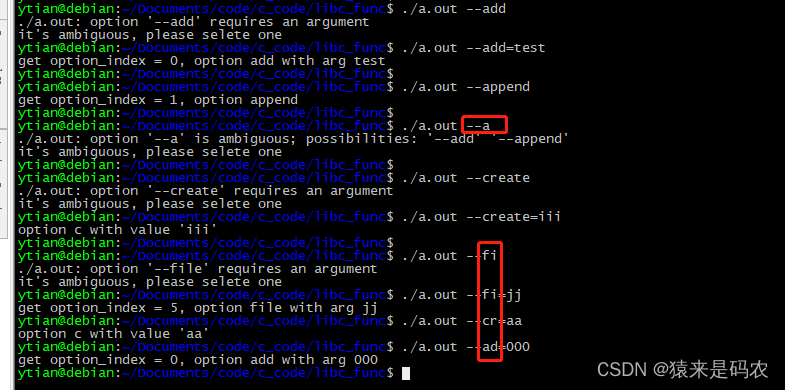
长选项只要匹配到前n(n<=strlen(name))个字符就可以。如果有多个匹配到则返回 ?,同时stderr 会有输出信息。这个函数里涉及到的全局变量:
1,optind 表示在argv中要处理的下一个元素的索引
2,optarg 当匹配到选项时,它指向的就是选项的参数,如上面的iii, jj, aa, 000