孔乙己:new的五种写法
这个是目标类:_INT
拥有一个字面常量构造函数,和一个平凡析构函数
可以从int构造,也可以隐式转换为int
也可以和int比较大小
class _INT
{
private:
int value;
public:
constexpr explicit _INT(int _Val0 = 0) noexcept:value(_Val0) {}
~_INT() {}
operator int()
{
return value;
}
bool operator ==(int _Right)
{
return value == _Right;
}
bool operator <(int _Right)
{
return value < _Right;
}
};
第一种写法:
-
auto _Ptr1 = new _INT(1);
对应释放方式:delete _Ptr1;
第二种写法:
-
auto _Ptr2 = new (malloc(sizeof(_INT))) _INT(2);
对应释放方式:free(_Ptr2);
第三种写法:
-
auto _Ptr3 = new (operator new(sizeof(_INT))) _INT(3);
对应释放方式:
_Ptr3->~_INT();
operator delete(_Ptr3);
或( delete _Ptr3; )
第四种写法:
char memory[0x1000];
-
auto _Ptr4 = new (memory) _INT(4);
对应释放方式:
_Ptr4->~_INT();
memset(_Ptr4, 0, sizeof(_INT));
第五种写法:
allocator<_INT> alloc;
- auto _Ptr5 = alloc.allocate(1);
-
allocator_traits<decltype(alloc)>::construct(alloc,_Ptr5, 5);
对应释放方式:
allocator_traits<decltype(alloc)>::destroy(alloc, _Ptr5);
alloc.deallocate(_Ptr5, 1);
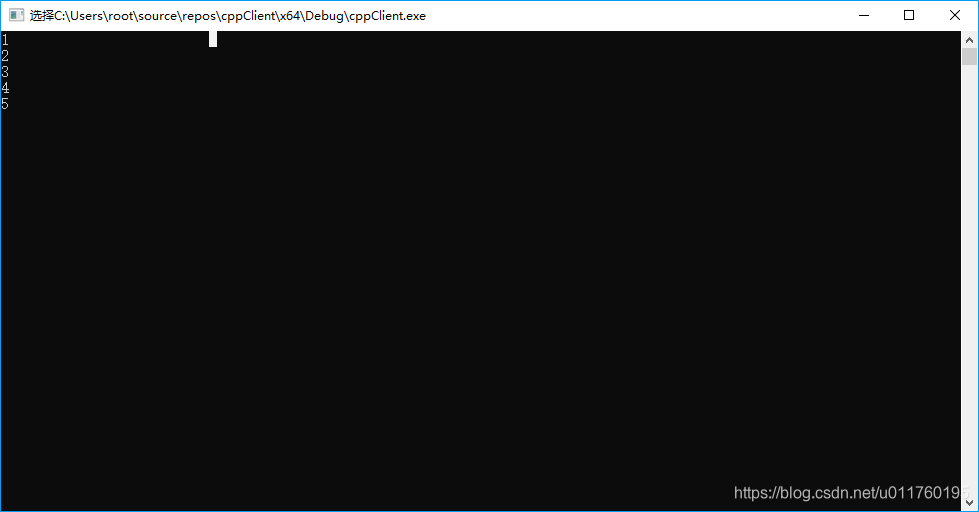
#include <iostream>
#include <memory>
#include <list>
#include <vector>
#include <memory>
using namespace std;
class _INT
{
private:
int value;
public:
constexpr explicit _INT(int _Val0 = 0) noexcept:value(_Val0) {}
~_INT() {}
operator int()
{
return value;
}
bool operator ==(int _Right)
{
return value == _Right;
}
bool operator <(int _Right)
{
return value < _Right;
}
};
int main()
{
char memory[0x1000];
allocator<_INT> alloc;
auto _Ptr1 = new _INT(1);
auto _Ptr2 = new (malloc(sizeof(_INT))) _INT(2);
auto _Ptr3 = new (operator new(sizeof(_INT))) _INT(3);
auto _Ptr4 = new (memory) _INT(4);
auto _Ptr5 = alloc.allocate(1);
allocator_traits<decltype(alloc)>::construct(alloc,_Ptr5, 5);
cout << *_Ptr1 << endl;
cout << *_Ptr2 << endl;
cout << *_Ptr3 << endl;
cout << *_Ptr4 << endl;
cout << *_Ptr5 << endl;
delete _Ptr1;
free(_Ptr2);
_Ptr3->~_INT();
operator delete(_Ptr3); // delete _Ptr3
memset(_Ptr4, 0, sizeof(_INT));
allocator_traits<decltype(alloc)>::destroy(alloc, _Ptr5);
alloc.deallocate(_Ptr5, 1);
return 0;
}