文章目录
- 生产者和消费者模型的概念
- 基于阻塞队列下的生产者和消费者模型
- 信号量
- 基于循环队列下的生产者和消费者模型
- 线程池的概念
- 读者-写者模型
- 自旋锁
生产者和消费者模型的概念
优点:
1,将生产环节和消费环节解耦。
2,极大的提高效率。
3,支持并发。
321原则
3种关系:
1,生产者和生产者:竞争 和 互斥。
2,消费者和消费者:竞争 和 互斥。
3,生产者和消费者: 同步 和 互斥。
2种角色:
生产者和消费者:本质是执行流
1个交易场所:一段缓冲区(缓冲区)。
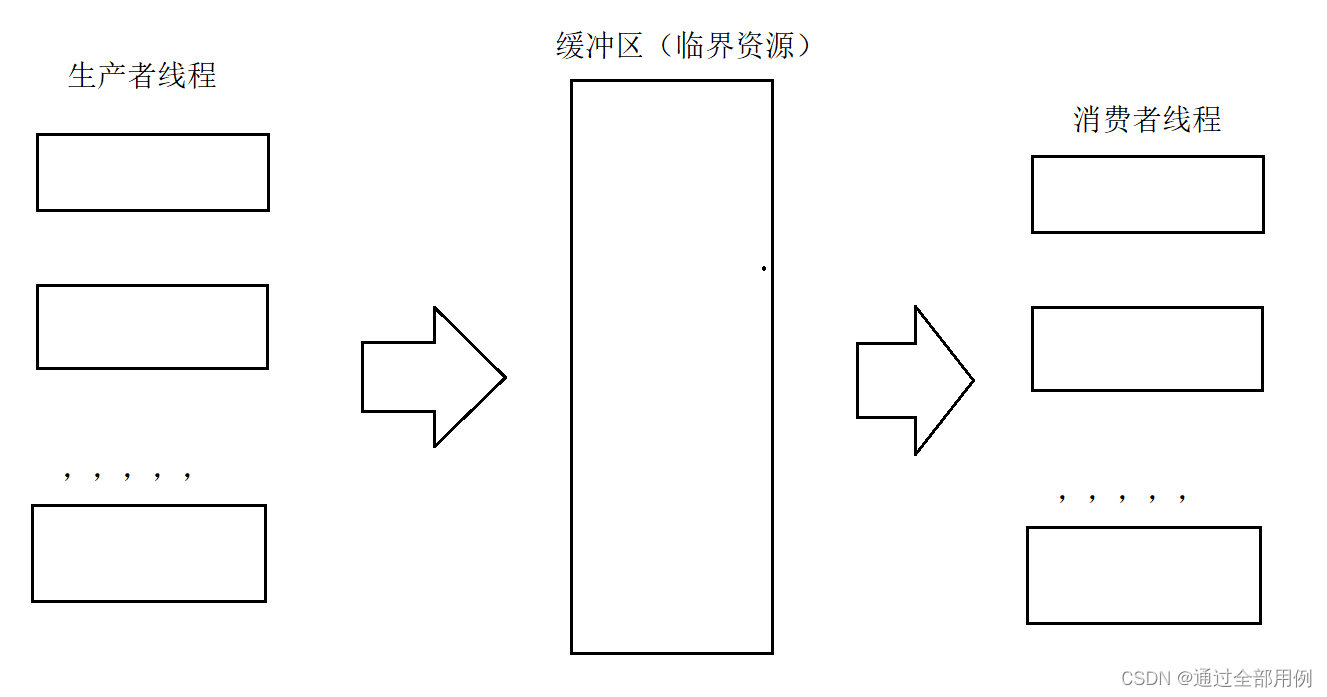
基于阻塞队列下的生产者和消费者模型
当队列为空时,从队列获取元素的操作将会被阻塞,直到队列中被放入了元素,
当队列满时,往队列里存放元素的操作也会被阻塞。
主要练习的是条件变量,并对条件变量的操作函数进行掌握
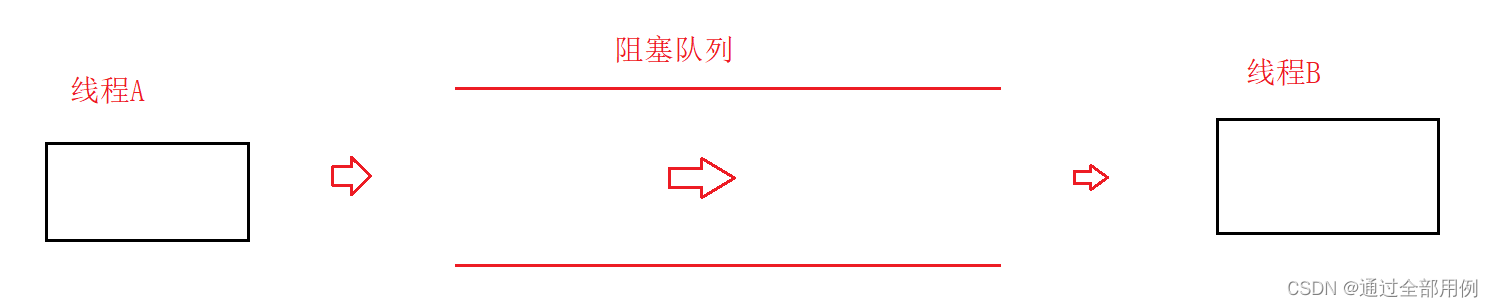
C++代码
amespace chen
{
const int default_cap = 5;
template <class T>
class block_queue
{
public:
block_queue()
: _cap(default_cap)
{
pthread_mutex_init(&_mtx, nullptr);
pthread_cond_init(&_isfull, nullptr);
pthread_cond_init(&_isempty, nullptr);
}
void pq_lock()
{
pthread_mutex_lock(&_mtx);
}
void pq_unlock()
{
pthread_mutex_unlock(&_mtx);
}
void consumer_wait()
{
pthread_cond_wait(&_isempty, &_mtx);
}
void wakeup_consumer()
{
pthread_cond_signal(&_isempty);
}
void product_wait()
{
pthread_cond_wait(&_isfull, &_mtx);
}
void wakeup_product()
{
pthread_cond_signal(&_isfull);
}
bool isfull()
{
return _cap == _bq.size();
}
bool isempty()
{
return _bq.size() == 0;
}
void Push(const T& in)
{
pq_lock();
while(isfull())
{
cout << "队列为满: " << _cap << endl;
product_wait();
}
_bq.push(in);
wakeup_consumer();
pq_unlock();
}
void Pop(T* out)
{
pq_lock();
while(isempty())
{
consumer_wait();
}
*out = _bq.front();
_bq.pop();
wakeup_product();
pq_unlock();
}
~block_queue()
{
pthread_mutex_destroy(&_mtx);
pthread_cond_destroy(&_isfull);
pthread_cond_destroy(&_isempty);
}
private:
queue<T> _bq;
int _cap;
pthread_mutex_t _mtx;
pthread_cond_t _isfull;
pthread_cond_t _isempty;
};
}
信号量
什么是信号量呢?
本质就是一个计数器,描述临界资源的数量。
临界资源本质上可以划分一个一个的小资源,通过信号量,完全可以让多个线程去
同时访问临界资源中的不同区域,从而实现并发。
线程操作函数:
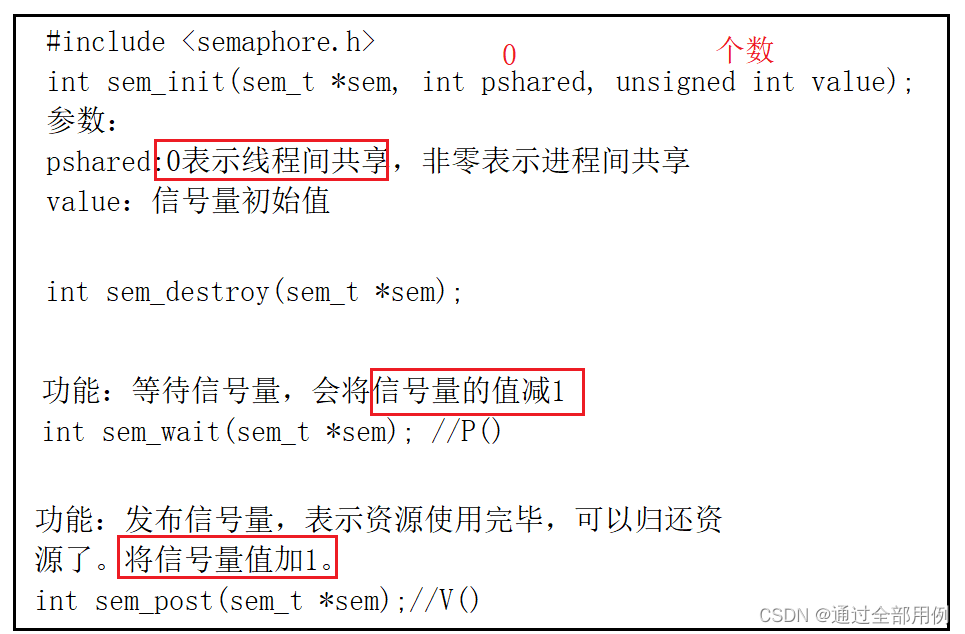
基于循环队列下的生产者和消费者模型
复习一下循环队列
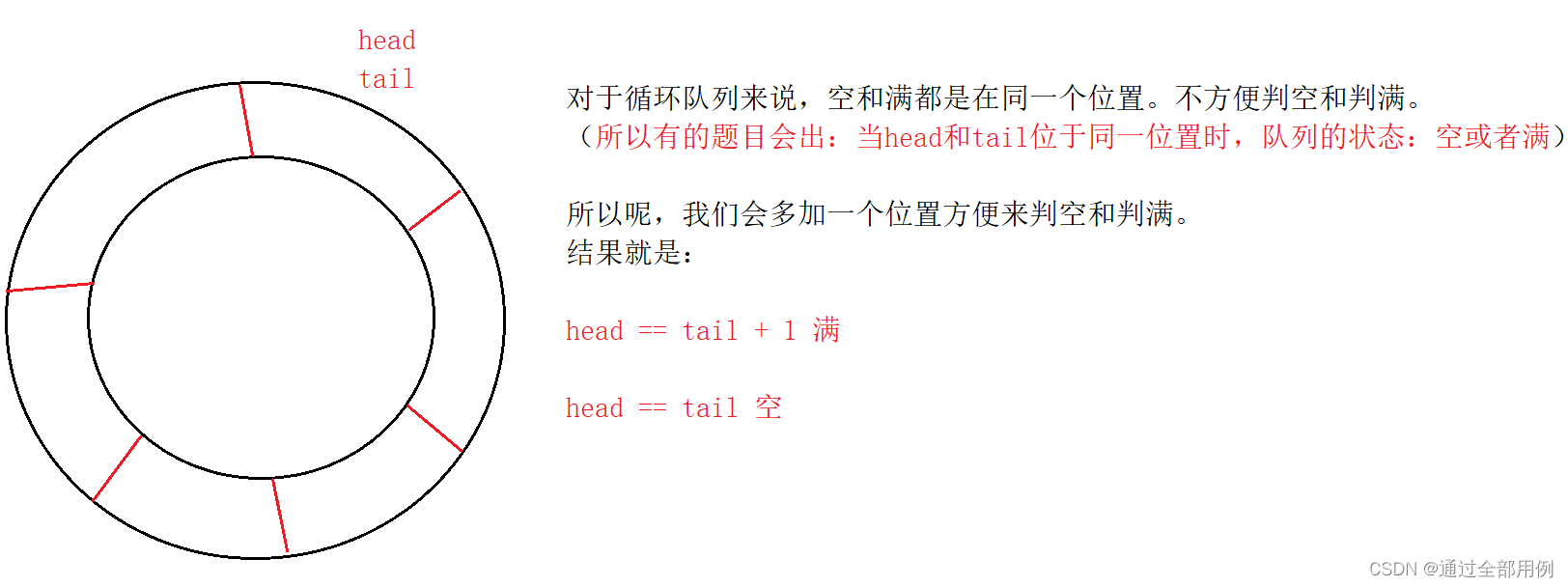
我们如何实现基于循环队列下的生产者和消费者模型呢? 我们这里不需要多加一个位置
利用信号量:定义两个信号量。 一个表示sem_blank 临界资源格子的数量, 一个表示sem_data临界资源数据的数量。
同一个位置,为空或者为满,生产者和消费者需要同步互斥。
不在同一个位置,可以并发执行。
C++代码:
#pragma once
#include <iostream>
#include <pthread.h>
#include <semaphore.h>
#include <time.h>
#include <unistd.h>
#include <vector>
using namespace std;
namespace chen
{
template <class T>
class ring_queue
{
public:
ring_queue(int cap = 10)
: _cap(cap)
,_pstep(0)
,_cstep(0)
{
_ring_queue.resize(_cap,0);
pthread_mutex_init(&_pmtx, nullptr);
pthread_mutex_init(&_cmtx, nullptr);
sem_init(&_blanksem, 0, 10);
sem_init(&_datasem, 0, 0);
}
void Push(const T &val)
{
sem_wait(&_blanksem);
pthread_mutex_lock(&_pmtx);
_ring_queue[_pstep] = val;
cout << "生产下标" << _pstep << endl;
_pstep++;
_pstep %= _cap;
pthread_mutex_unlock(&_pmtx);
sem_post(&_datasem);
}
void Pop(T *out)
{
sem_wait(&_datasem);
pthread_mutex_lock(&_cmtx);
*out = _ring_queue[_cstep];
cout << "消费下标" << _cstep << endl;
_cstep++;
_cstep %= _cap;
pthread_mutex_unlock(&_cmtx);
sem_post(&_blanksem);
}
~ring_queue()
{
pthread_mutex_destroy(&_pmtx);
pthread_mutex_destroy(&_cmtx);
sem_destroy(&_blanksem);
sem_destroy(&_datasem);
}
private:
vector<T> _ring_queue;
int _cap;
pthread_mutex_t _pmtx;
pthread_mutex_t _cmtx;
sem_t _blanksem;
sem_t _datasem;
int _pstep;
int _cstep;
};
}
线程池的概念
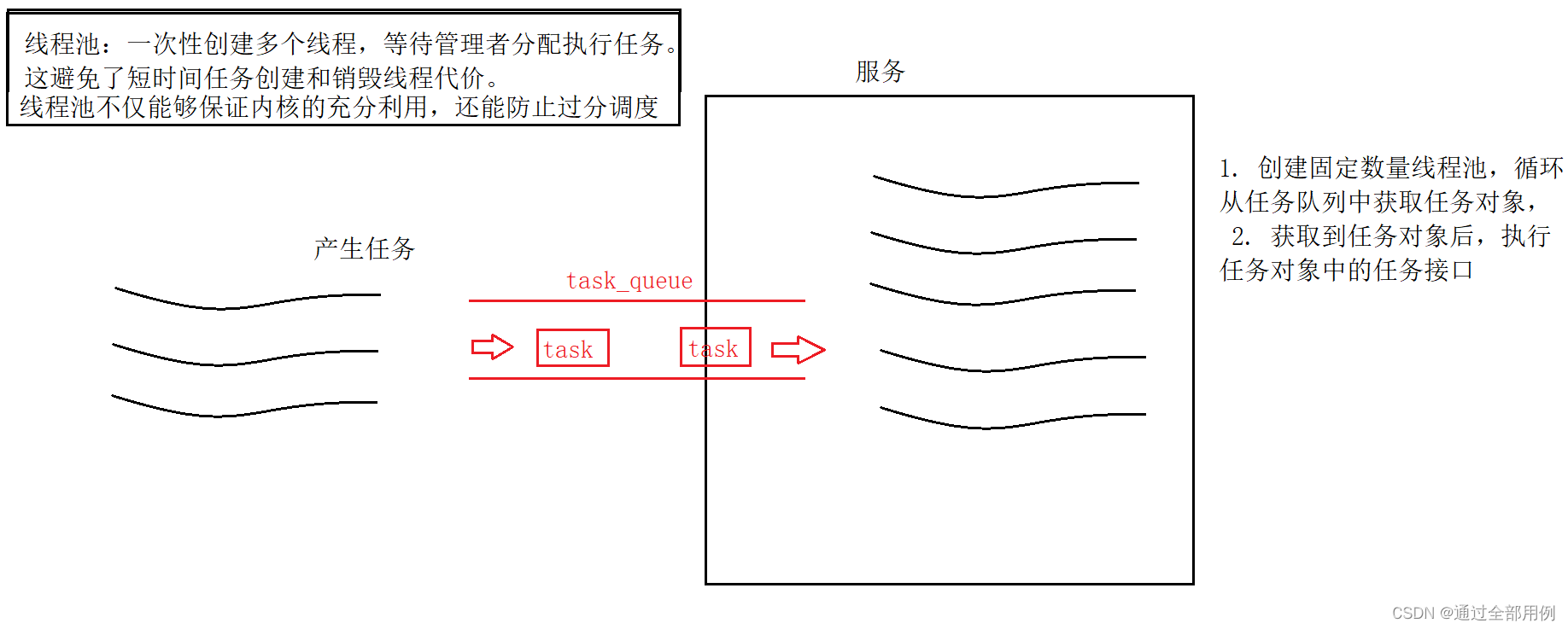
#pragma once
#include <iostream>
#include <pthread.h>
#include <queue>
#include <unistd.h>
using namespace std;
namespace chen
{
template<class T>
class thread_pool
{
public:
thread_pool()
:_num(5)
{
pthread_cond_init(&_cond, nullptr);
pthread_mutex_init(&_mtx, nullptr);
}
void Lock()
{
pthread_mutex_lock(&_mtx);
}
void Unlock()
{
pthread_mutex_unlock(&_mtx);
}
void Wait()
{
pthread_cond_wait(&_cond, &_mtx);
}
void Wakeup()
{
pthread_cond_signal(&_cond);
}
static void* pthread_run(void* args)
{
pthread_detach(pthread_self());
thread_pool<T>* tp = (thread_pool<T>*)args;
while(true)
{
tp->Lock();
while(tp->_task_queue.empty())
{
tp->Wait();
}
tp->pop_task();
tp->Unlock();
sleep(5);
}
}
void init_thread_pool()
{
pthread_t tid;
for(int i = 0; i < _num; i++)
{
pthread_create(&tid, nullptr, pthread_run, (void*)this);
}
}
void push_task(const T& in)
{
_task_queue.push(in);
Wakeup();
}
void pop_task()
{
_task_queue.pop();
cout << "我是线程:" << pthread_self() << "正在处理任务" << endl;
}
~thread_pool()
{
pthread_cond_destroy(&_cond);
pthread_mutex_destroy(&_mtx);
}
private:
int _num;
queue<T> _task_queue;
pthread_cond_t _cond;
pthread_mutex_t _mtx;
};
}
读者-写者模型
适合的是多读少写的场景
写独占,读共享,读锁优先级高
321原则
3:3种关系:
读者和读者 (无关系,因为读者不会取走数据,与消费者生产者模型不同,是因为消费者会取走资源)
写者和写者:互斥
读者和写者:互斥,同步
2:2种角色 2个执行流(线程)
1个交易场所:一段缓冲区 或者一个STL容器(queue,vector)
优先级的概念:
读者优先:当读者和写者同时到来的时候,让读者先访问。
写者优先:当读者和写者同时来的时候, 当前读者和将要晚来的读者都要等待,都不进入临界区,当临界区没有读者的时候
该写者才进入临界区。
自旋锁
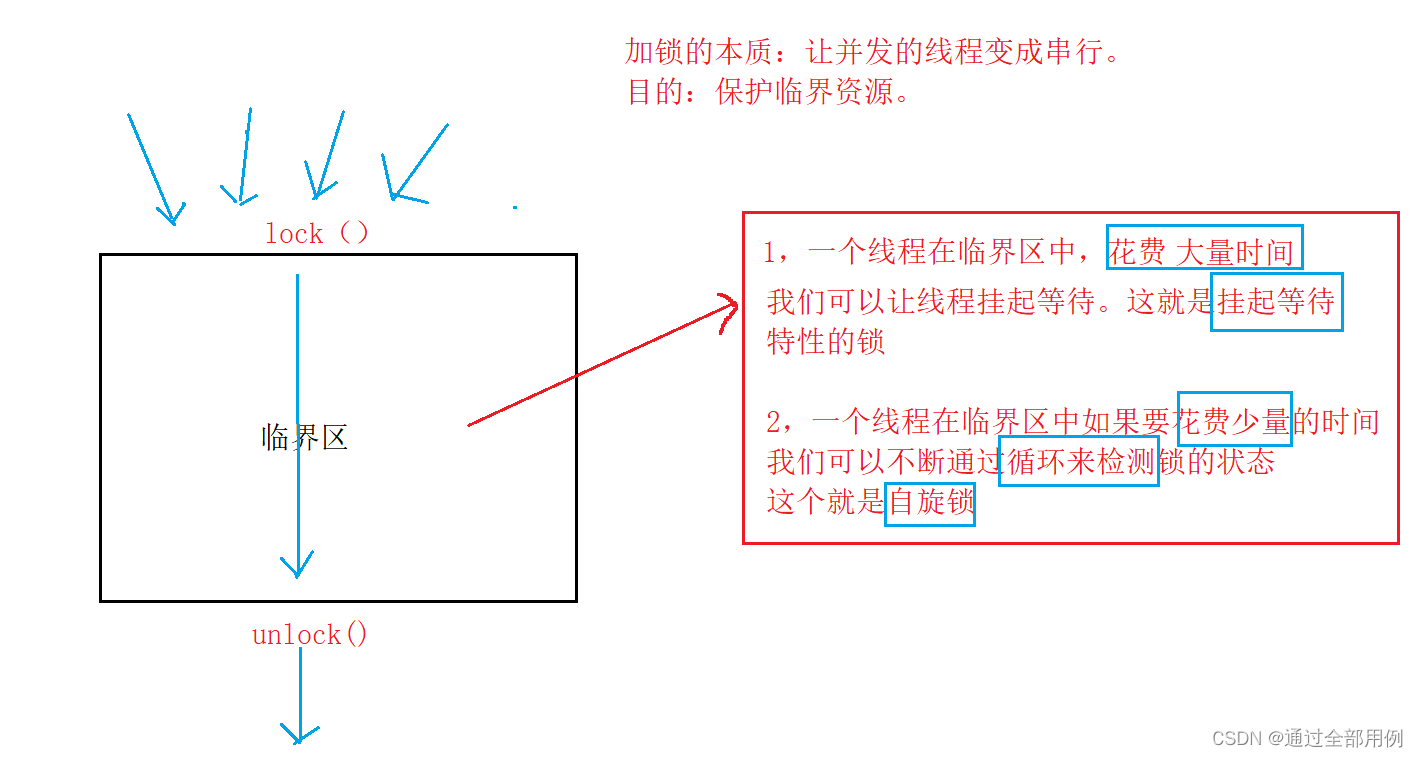
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)